How to Match Newline Characters in R Regex
-
Matching Newlines in R Using
\n
for Linux/Unix -
Matching Newlines in R Using
\r\n
for Windows - Matching Newlines in R Using `
-
Matching Newlines in R Using
\r?\n
for Cross-Platform -
Matching Newlines in R Using
(\r\n|\r|\n)
for Cross-Platform With Old Mac - Test Regex With Newline Sequences
- Conclusion
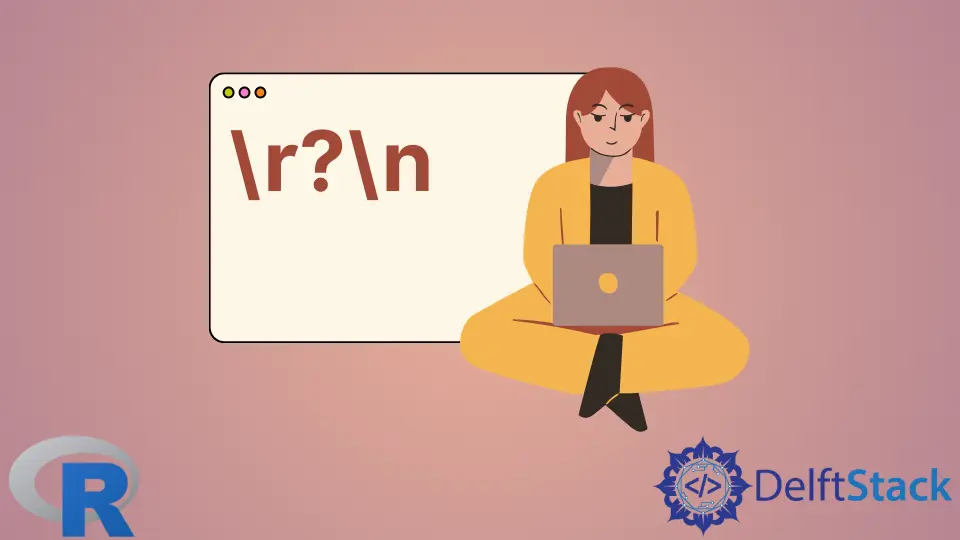
Regular expressions (regex) are powerful tools for pattern matching in strings, and they play a crucial role in text processing tasks. In this detailed guide, we explore various techniques to match newline sequences, ensuring compatibility across Linux, Windows, and even older Mac environments.
Matching Newlines in R Using \n
for Linux/Unix
In the context of Linux/Unix, the regex pattern \n
specifically targets and matches newline characters, a crucial operation for effective text processing in these systems.
Let’s dive into a complete working example using the grepl
function in R with Perl mode (perl = TRUE
). We’ll use the sample text This is a line of text.\n
to illustrate the concept.
Example:
# Sample text with a newline character (LF - Linux/Unix)
text <- "This is a line of text.\n"
# Matching Linux/Unix newline (\n) using 'grepl' with Perl mode
pattern <- "\n"
result <- grepl(pattern, text, perl = TRUE)
# Output the result
print(result)
We start with a sample text containing a newline character (\n
). This character is common in Linux/Unix systems to represent the end of a line.
We focus on matching the Linux/Unix newline character \n
. The pattern is a simple representation of this character.
Then the grepl
function is used for pattern matching. Setting perl = TRUE
enables Perl-compatible regular expressions, providing a broader set of regex features.
Finally, we print the result of the regex match. The result is a logical value (TRUE
if a match is found, FALSE
otherwise).
Output:
[1] TRUE
In this output, the result would be TRUE
since the sample text contains the Linux/Unix newline character \n
. This method allows us to efficiently identify and work with newline characters in R.
Matching Newlines in R Using \r\n
for Windows
For Windows newline representation (CRLF - Carriage Return + Line Feed), the regex pattern \r\n
efficiently matches the combined carriage return and line feed, which is crucial for accurate text processing.
Let’s delve into a thorough example using the grepl
function in R with Perl mode (perl = TRUE
). To illustrate the concept, we’ll work with the sample text This is a line of text.\r\n
.
Example:
# Sample text with a Windows newline (\r\n)
text <- "This is a line of text.\r\n"
# Matching Windows newline (\r\n) using 'grepl' with Perl mode
pattern <- "\r\n"
result <- grepl(pattern, text, perl = TRUE)
# Output the result
print(result)
We begin with a sample text containing a Windows newline character (\r\n
). In Windows environments, a newline is typically represented by a combination of carriage return and line feed.
Then, we are specifically targeting the Windows newline character \r\n
. The pattern is a straightforward representation of this sequence.
Utilizing the grepl
function, we initiate the regex pattern-matching process. The perl = TRUE
parameter ensures Perl-compatible regular expressions, allowing us to handle the special characters involved in Windows newlines.
The critical moment arrives as we print the result of our regex match. The result is a logical value (TRUE
if a match is found, FALSE
otherwise).
Output:
[1] TRUE
The output of the code hinges on whether the pattern matches the Windows newline character in the given text. In this example, the result would be TRUE
as the sample text contains the Windows newline sequence \r\n
.
Matching Newlines in R Using `
` for Old Mac
In the context of older Mac systems, the regex pattern \r
serves to match a carriage return character. Alternatively, the pattern (\r)
provides another method to achieve the same, effectively capturing carriage return characters in older Mac environments.
Let’s delve into a practical example using the grepl
function in R with Perl mode (perl = TRUE
). We’ll use the sample text This is a line of text.\r
to illustrate the concept.
Example:
# Sample text with an older Mac newline (\r)
text <- "This is a line of text.\r"
# Matching older Mac newline (\r) using 'grepl' with Perl mode
pattern <- "\r"
result <- grepl(pattern, text, perl = TRUE)
# Output the result
print(result)
We start with a sample text containing an older Mac newline character (\r
). In older Mac systems, a newline is typically represented by a carriage return.
For this method, our focus is on matching the older Mac newline character \r
. The pattern is a concise representation of this single character.
Initiating the regex pattern matching process, we employ the grepl
function with Perl mode (perl = TRUE
). This mode allows us to work with special characters, such as the carriage return in this case.
The moment of truth arrives as we print the result of our regex match. The result is a logical value (TRUE
if a match is found, FALSE
otherwise).
Output:
[1] TRUE
The output of the code hinges on whether the pattern matches the older Mac newline character in the given text. In this example, the result would be TRUE
as the sample text contains the older Mac newline character \r
.
Matching Newlines in R Using \r?\n
for Cross-Platform
The cross-platform regex pattern \r?\n
is designed to match newline characters, accommodating both Linux/Unix and Windows environments. This method ensures versatility in newline matching across different operating systems.
Let’s dive into a practical example using the grepl
function in R with Perl mode (perl = TRUE
). We’ll use the sample text This is a line of text.\n
to demonstrate the effectiveness of the \r?\n
method.
# Sample text with a newline character (LF - Linux/Unix)
text <- "This is a line of text.\n"
# '\r?\n' newline matching using 'grepl' with Perl mode
pattern <- "\r?\n"
result <- grepl(pattern, text, perl = TRUE)
# Output the result
print(result)
We start with a sample text containing a newline character (\n
). This character is commonly used to represent the end of a line in Linux/Unix systems.
In the \r?\n
method, the regex pattern is concise and effective. It captures the presence of a carriage return (\r
) followed by an optional newline (\n
).
This pattern accommodates newline representations from Linux/Unix (\n
) and Windows (\r\n
).
We initiate the regex pattern matching process using the grepl
function with Perl mode (perl = TRUE
). This mode ensures compatibility with special characters used in newline representations.
The moment of truth arrives as we print the result of our regex match. The result is a logical value (TRUE
if a match is found, FALSE
otherwise).
[1] TRUE
The output of the code depends on whether the pattern matches any of the newline characters in the given text. In this example, the result would be TRUE
since the sample text contains the Linux/Unix newline character \n
.
Matching Newlines in R Using (\r\n|\r|\n)
for Cross-Platform With Old Mac
In a cross-platform context, the regex pattern (\r\n|\r|\n)
effectively matches newline characters, providing compatibility across Linux/Unix, Windows, and older systems. This method ensures comprehensive newline matching across various operating environments.
Let’s delve into a practical example using the grepl
function in R with Perl mode (perl = TRUE
). We’ll use the sample text This is a line of text.\n
to demonstrate the cross-platform newline matching concept.
Example:
# Sample text with a newline character (LF - Linux/Unix)
text <- "This is a line of text.\n"
# Cross-Platform newline matching using 'grepl' with Perl mode
pattern <- "(\r\n|\r|\n)"
result <- grepl(pattern, text, perl = TRUE)
# Output the result
print(result)
We start with a sample text containing a newline character (\n
). This character is commonly used to represent the end of a line in Linux/Unix systems.
In the Cross-Platform Method, we use a regex pattern that encompasses newline representations from Linux/Unix (\n
), Windows (\r\n
), and older Mac systems (\r
). The pattern is a combination of alternatives enclosed in parentheses.
We initiate the regex pattern matching process using the grepl
function with Perl mode (perl = TRUE
). This mode ensures compatibility with special characters used in newline representations.
The critical moment arrives as we print the result of our regex match. The result is a logical value (TRUE
if a match is found, FALSE
otherwise).
[1] TRUE
The output of the code depends on whether the pattern matches any of the newline characters in the given text. In this example, the result would be TRUE
since the sample text contains the Linux/Unix newline character \n
.
Test Regex With Newline Sequences
Many websites offer the possibility to test regular expressions. Most of them work like Linux environments, finding matches on strings with line feeds when testing the \n
pattern, but they don’t find a match when you test the \r\n
pattern.
Examples of these sites are Regex101 and regex tester in extendsclass.
Other testing websites could show different behaviors, just like it happens with different operating environments. For example, Regex Storm works more like Windows platforms, finding matches between strings with line breaks and the pattern \r\n
.
Conclusion
In conclusion, mastering newline matching in R ensures smoother text processing across diverse systems. The simplicity of \n
for Linux, \r\n
for Windows, and \r?\n
for cross-platform versatility makes these regex methods invaluable.
By integrating these techniques, R users can streamline their text processing workflows and enhance the adaptability of their data manipulation tasks.