How to Comment Out Multilines in R
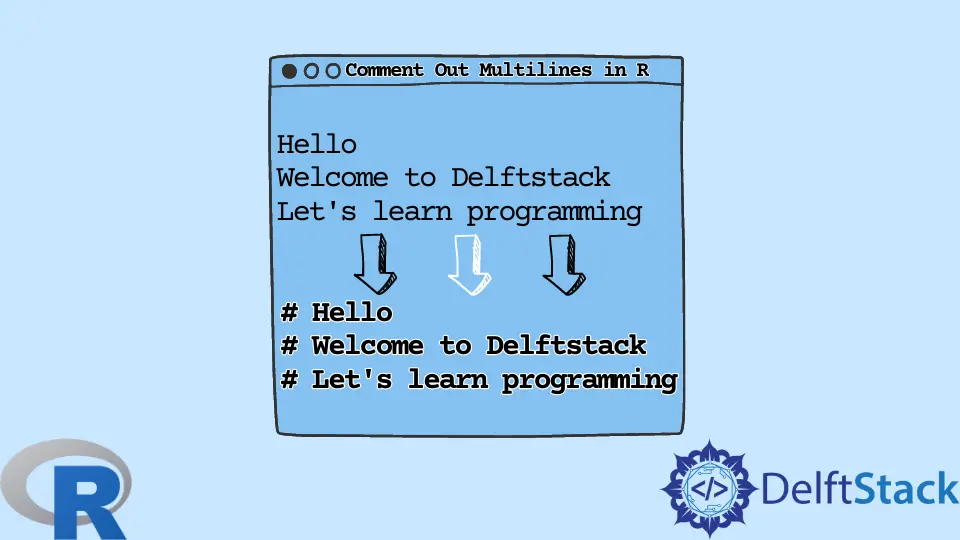
When you want to comment out multiline of R code, the conventional way to do it would be to place a #
character at the beginning of each of the lines you need to comment out since R does not support multiline comments.
Performing that task is ok if the number of lines of code to comment out is small. But if you need to comment out a really long block of code, a specialized code editor capable of adding a #
character to each line in a selected block could be useful. In RStudio, you can do that by using the Ctrl+Shift+C key combination in Windows, or Command+Shift+C in OSX. The RStudio documentation offers more information on keyboard shortcuts.
Notepad++ recognizes R code and also allows you to comment out code with the Ctrl+Q or Command+Q shortcut. If you use Emacs, you can also use M-x comment-region
. To revert the action, use M-x uncomment region
.
Use scan()
to Insert Arbitrary Text
You can also use the scan()
function to insert any arbitrary text inside your code, after which you need to use rm()
to remove the text from memory. Make sure that the last line of the commented code before the rm()
must be blank. Since scan()
loads the text in a variable, it is necessary to keep the commented code or text within reasonable size limits.
Below is how you can use this method.
comments <- scan(what="character")
Place your comments here
You can place code also:
some_data <- 1:1000
Just leave the last line blank.
rm(comments)
Use a Function to Comment Multiline in R
The following FormatComment
function takes the text from the clipboard and formats it with a #
symbol at the beginning of each line. So if you’re using a text editor incapable of commenting out blocks of code automatically, you can obtain the same result by copying the code to the clipboard and calling the FormatComment
function from the console to get a commented-out version of it. Then, you can replace the original code block with the commented one.
FormatComment<-function() {
y <- as.list(readClipboard())
spacer <- function(x) paste("#", paste(" ", collapse=""), x, sep="")
z <- sapply(y, spacer)
zz <- as.matrix(as.data.frame(z))
dimnames(zz) <- list(c(rep("", nrow(zz))), c(""))
writeClipboard(noquote(zz), format = 1)
return(noquote(zz))
}
To try it, just copy whatever text you want to include as a comment and call the FormatComment
function. For example, if you’re going to convert this text into a comment.
This is some text
I want to insert
as a comment
in the middle of
my R script.
Just select the entire block and copy it to the clipboard. Then call the FormatComment
function like this:
FormatComment()
And you will get the following output:
# This is some text
# I want to insert
# as a comment
# in the middle of
# my R script.