How to Find Mode of a Vector in R
- Method 1: Using the table() Function
- Method 2: Using dplyr Package
- Method 3: Custom Function for Mode Calculation
- Conclusion
- FAQ
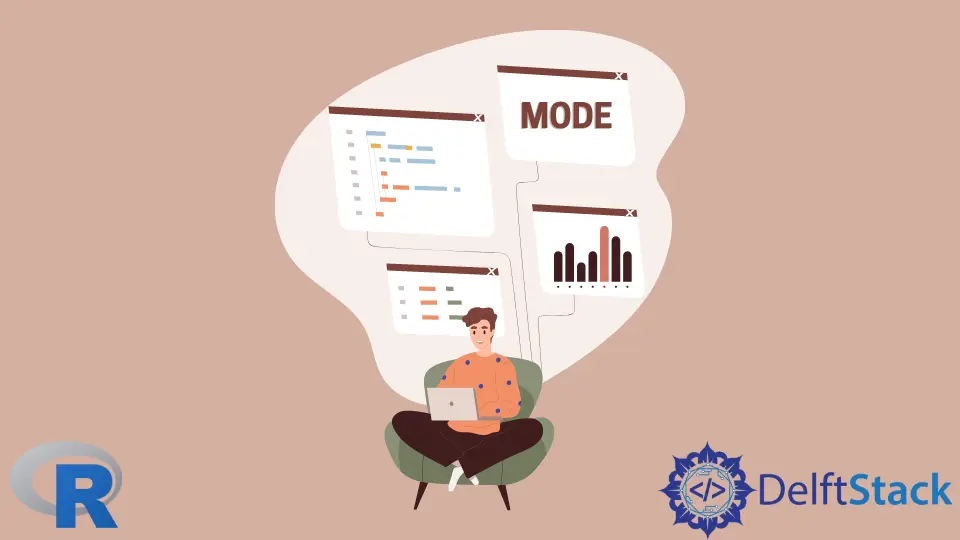
Finding the mode of a vector in R can be a bit tricky, especially since R does not have a built-in function for this specific task. The mode is the value that appears most frequently in a dataset, and identifying it can be crucial for data analysis.
In this article, we will explore several methods to find the mode of a vector in R. Whether you are a beginner or an experienced R user, you will find these techniques helpful for your data analysis needs. Let’s dive into the world of R and uncover how to efficiently determine the mode of a vector.
Method 1: Using the table() Function
One of the simplest ways to find the mode in R is by utilizing the table()
function. This function creates a frequency table of the elements in the vector. By examining this table, you can easily identify the mode.
my_vector <- c(1, 2, 2, 3, 4, 4, 4, 5)
frequency_table <- table(my_vector)
mode_value <- as.numeric(names(frequency_table[frequency_table == max(frequency_table)]))
mode_value
Output:
4
The table()
function counts the occurrences of each value in the vector. In this example, we first create a vector called my_vector
with some repeated values. Then, we generate a frequency table with table(my_vector)
, which counts how many times each number appears. The next step is to find the maximum frequency using max(frequency_table)
, and we extract the corresponding names (the modes) using names()
. Finally, we convert the mode to numeric format. This method is efficient and straightforward, making it ideal for quick analysis.
Method 2: Using dplyr Package
If you prefer a tidyverse approach, the dplyr
package provides a clean and efficient way to find the mode. This method is particularly useful when dealing with larger datasets or when you want to integrate mode calculation into a data manipulation pipeline.
library(dplyr)
my_vector <- c(1, 2, 2, 3, 4, 4, 4, 5)
mode_value <- my_vector %>%
as.data.frame() %>%
group_by(value = .) %>%
summarise(count = n()) %>%
arrange(desc(count)) %>%
slice(1) %>%
pull(value)
mode_value
Output:
4
In this method, we first convert the vector into a data frame using as.data.frame()
. The group_by()
function groups the data by the values in the vector, and summarise(count = n())
counts the occurrences of each value. We then use arrange(desc(count))
to sort the data frame in descending order based on the count. Finally, slice(1)
extracts the first row, which contains the mode, and pull(value)
retrieves the mode value. This method is not only elegant but also integrates seamlessly with other dplyr
functions for more complex data manipulation tasks.
Method 3: Custom Function for Mode Calculation
Creating a custom function to find the mode allows for flexibility and reusability in your R scripts. This method can be particularly useful when you need to calculate the mode multiple times throughout your analysis.
find_mode <- function(vec) {
unique_vec <- unique(vec)
mode_value <- unique_vec[which.max(tabulate(match(vec, unique_vec)))]
return(mode_value)
}
my_vector <- c(1, 2, 2, 3, 4, 4, 4, 5)
mode_value <- find_mode(my_vector)
mode_value
Output:
4
In this custom function, find_mode
, we first identify the unique values in the vector using unique()
. The match()
function finds the positions of the unique values in the original vector, and tabulate()
counts how many times each unique value appears. Finally, which.max()
identifies the position of the maximum count, allowing us to extract the mode. This method is flexible and can be reused easily, making it a valuable tool in your R toolkit.
Conclusion
Finding the mode of a vector in R may not be as straightforward as other statistical measures, but with the methods we discussed—using the table()
function, the dplyr
package, and a custom function—you can easily determine the mode in any dataset. Each method has its advantages, so you can choose one based on your preferences and the context of your analysis. Armed with these techniques, you’re now ready to tackle mode calculations in R confidently. Happy coding!
FAQ
- What is the mode in statistics?
The mode is the value that appears most frequently in a dataset.
-
Why is finding the mode important?
The mode helps to identify the most common value in a dataset, which can provide insights into trends and patterns. -
Can there be more than one mode in a dataset?
Yes, a dataset can be unimodal (one mode), bimodal (two modes), or multimodal (multiple modes). -
Does R have a built-in function for mode?
No, R does not have a built-in function for mode, but you can calculate it using various methods as discussed. -
How can I install the dplyr package in R?
You can install dplyr by running the command install.packages(“dplyr”) in your R console.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook