How to Create Word Cloud in Python
-
Install the
wordcloud
Package in Python - Import Pertinent Libraries in Python
- Generate Word Cloud in Python
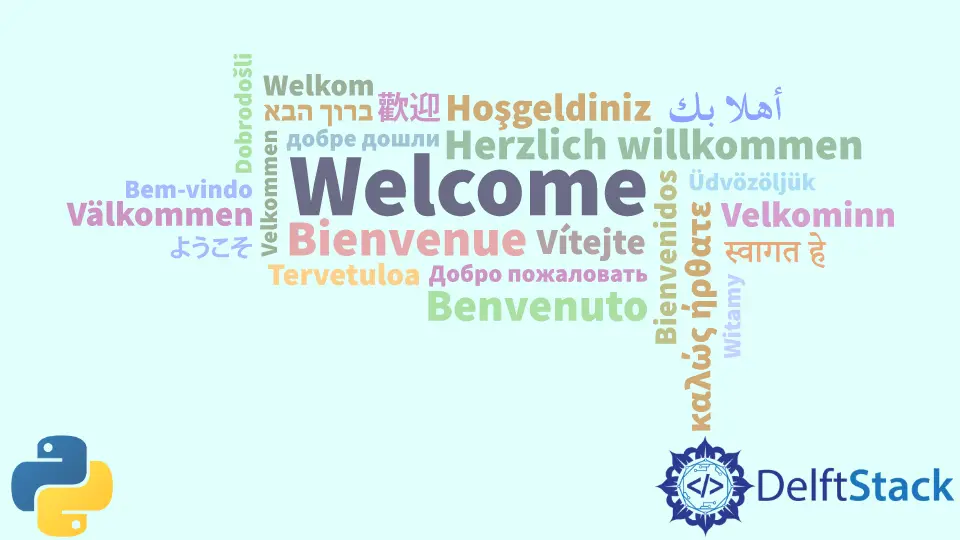
This tutorial will go through a method to create a word cloud in Python using the wordcloud
package.
Install the wordcloud
Package in Python
First, we will have to install the wordcloud
package in Python, including the Matplotlib
package.
pip install wordcloud
The above command will install the wordcloud
and the Matplotlib
packages, which we will use to create the word cloud.
Now, let us import the libraries we need to create a word cloud, namely WordCloud
, STOPWORDS
and matplotlib.pyplot
.
Import Pertinent Libraries in Python
from wordcloud import WordCloud, STOPWORDS
import matplotlib.pyplot as plt
The above code will import all the required libraries.
Let us now create a set of stopwords to help us avoid adding stopwords in the word cloud from our sample string. You can learn more about this process by reading about How to Remove Stop Words in Python.
stopwords = set(STOPWORDS)
Let us take a sample string from which we will create a word cloud containing the most frequent words in our string.
text_str = (
"peep gate do it but peep heal gate also not heal do it but gate peep peep peep"
)
Generate Word Cloud in Python
We will use this string in our function to create the word cloud. Let us now create a function present_wordcloud()
where we use the Worcloud()
function with all the suitable parameters to create the word cloud.
def present_wordcloud(stri, title=None):
wordcloud = WordCloud(
background_color="white",
stopwords=stopwords,
max_words=300,
max_font_size=50,
scale=3,
random_state=1,
).generate(str(stri))
In the above code, we pass suitable parameters to our function and pass our string to the generate()
function for generating word cloud. We will now display our word cloud using the Matplotlib
library by adding the below code in our present_wordcloud()
function.
def present_wordcloud(stri, title=None):
wordcloud = WordCloud(
background_color="white",
stopwords=stopwords,
max_words=200,
max_font_size=40,
scale=3,
random_state=1,
).generate(str(stri))
fig = plt.figure(1, figsize=(9, 9))
plt.axis("off")
if title:
fig.suptitle(title, fontsize=18)
fig.subplots_adjust(top=2.4)
plt.imshow(wordcloud)
plt.show()
As shown above, we display our word cloud by adding the figure size, the subtitle, and the font size to our plt
screen. The plt.imshow()
function will display the word cloud on a screen.
Now run the present_wordcloud()
function to see the word cloud generated for our sample string by running the code below.
if __name__ == "__main__":
present_wordcloud(text_str)
By running our main function, we get the below output.
We got a word cloud from our sample string where peep
is the most frequent word in our sentence.
Thus we have successfully created a word cloud in Python. If you’re interested in further understanding word processing in Python, check out Bigrams in Python.