Urllib2 in Python 3
-
the
urllib
in Python 3 -
Understand the Root Cause of the
ModuleNotFoundError: No module named 'urllib2'
in Python -
Replicate the
ModuleNotFoundError: No module named 'urllib2'
in Python -
Solve the
ModuleNotFoundError: No module named 'urllib2'
in Python
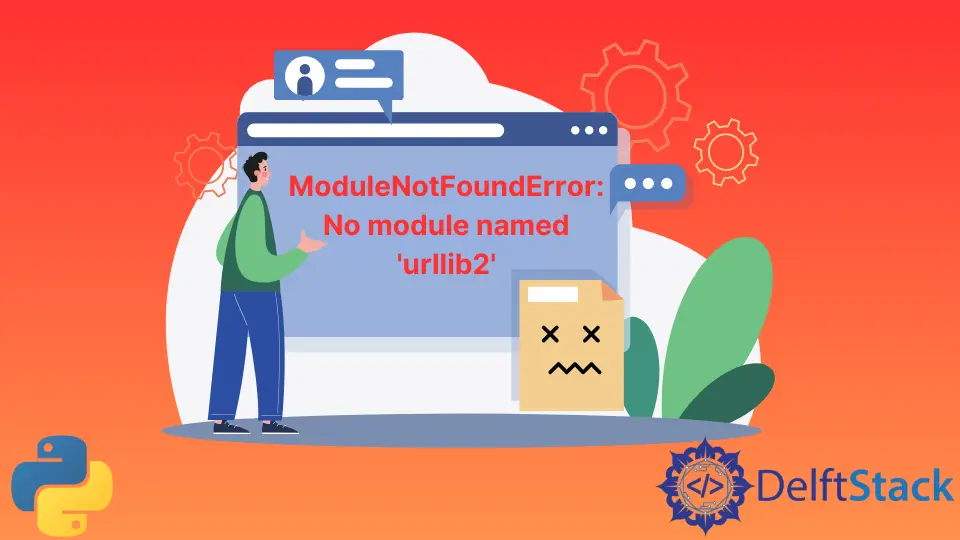
In this tutorial, we aim to explore methods to solve the issue of ModuleNotFoundError: No module named 'urllib2'
in Python.
the urllib
in Python 3
The Python module for processing URLs is called the urllib
package. URLs can be fetched with it, and it can fetch URLs using various protocols and the urlopen
method.
Some other methods associated with the urllib
package are:
urllib.parse
- This method is mainly used to parse the data within a particular URL.urllib.request
- This method is mainly used to request and process the URL passed as a parameter.urllib.robotparser
- This method parsesrobot.txt
types of files.urllib.error
- This method is mainly used to identify any errors raised while using theurllib.request
function.
Understand the Root Cause of the ModuleNotFoundError: No module named 'urllib2'
in Python
The error message most generally faced when it comes to urllib2
in Python 3 is the ModuleNotFoundError
. This error is mainly because the urllib2
is no longer supported in Python 3.
According to the urllib2
documentation, the urllib2
module has been split across several modules in Python 3 named urllib.request
and urllib.error
. Now let us understand how to replicate this issue.
Replicate the ModuleNotFoundError: No module named 'urllib2'
in Python
The above issue can be illustrated with the help of the following block of code.
import urllib2
Output:
ModuleNotFoundError: No module named 'urllib2'
Another scenario that produces the error is using the urlopen
function within the urllib2
package. This function has been moved to a new place called request
within the package in Python 3.
This problem can be replicated with the help of the following block of code.
import urllib2.request
response = urllib2.urlopen("http://www.google.com")
html = response.read()
print(html)
The output of the above code can be illustrated as follows.
ModuleNotFoundError: No module named 'urllib2'
Now that we have seen how to replicate the issue let us try to correct it.
Solve the ModuleNotFoundError: No module named 'urllib2'
in Python
To solve the error, we need some changes to the code above.
- Use
urllib.request
instead ofurllib2
. - Use
urlopen("http://www.google.com/")
instead ofurllib2.urlopen("http://www.google.com")
.
The above changes are implemented with the help of the following block of code.
from urllib.request import urlopen
html = urlopen("http://www.google.com/").read()
print(html)
The above code would successfully read out the elements of the page http://www.google.com/
.
Thus, we have successfully solved the problem associated with urllib2
in Python 3.