How to Suppress Warnings in Python
-
Use the
filterwarnings()
Function to Suppress Warnings in Python -
Use the
-Wignore
Option to Suppress Warnings in Python -
Use the
PYTHONWARNINGS
Environment Variable to Suppress Warnings in Python
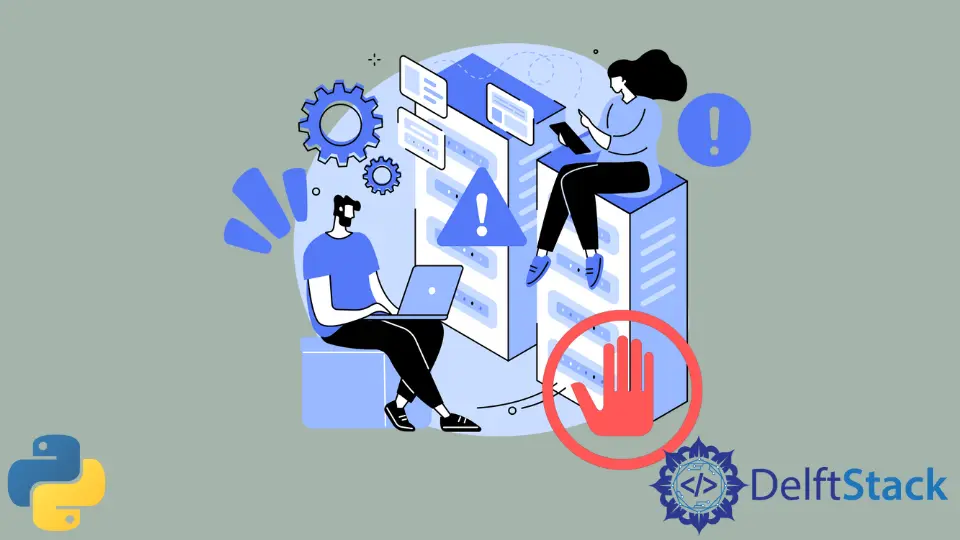
Warnings in Python are raised when some outdated class, function, keyword, etc., are used. These are not like errors. When an error occurs in a program, the program terminates. But, if there are warnings in the program, it continues to run.
This tutorial demonstrates how to suppress the warnings in the programs in Python.
Use the filterwarnings()
Function to Suppress Warnings in Python
The warnings
module handles warnings in Python. We can show warnings raised by the user with the warn() function. We can use the filterwarnings()
function to perform actions on specific warnings.
For example,
import warnings
warnings.filterwarnings(
"ignore",
".*do not.*",
)
warnings.warn("DelftStack")
warnings.warn("Do not show this message")
Output:
<string>:3: UserWarning: DelftStack
As observed, the action ignore
in the filter is triggered when the Do not show this message warning
is raised, and only the DelftStack
warning is shown.
We can suppress all the warnings by just using the ignore
action.
See the code below.
import warnings
warnings.filterwarnings("ignore")
warnings.warn("DelftStack")
warnings.warn("Do not show this message")
print("No Warning Shown")
Output:
No Warning Shown
Use the -Wignore
Option to Suppress Warnings in Python
The -W
option helps to keep control of whether the warning has to be printed or not. But the option has to be given a specific value. It is not necessary to provide only one value. We can offer more than one value to the option, but the -W
option will consider the last value.
To completely suppress the warnings -Wignore
option is used. We have to use this in the command prompt while running the file, as shown below.
python -W warningsexample.py
Use the PYTHONWARNINGS
Environment Variable to Suppress Warnings in Python
We can export a new environment variable in Python 2.7 and up. We can export PYTHONWARNINGS
and set it to ignore to suppress the warnings raised in the Python program.