How to Convert String to Hex in Python
-
Using the
encode()
Method to Convert String to Hexadecimal in Python -
Using the
binascii
Module to Convert String to Hexadecimal in Python -
Using the
hex()
Function in Combination Withmap()
andord()
to Convert String to Hexadecimal in Python - Conclusion
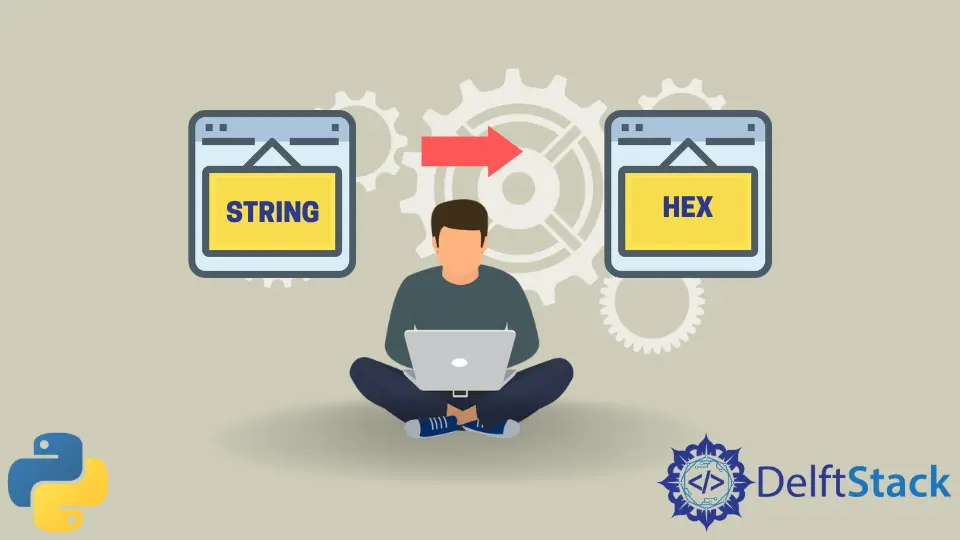
Converting a string to hexadecimal in Python refers to the process of transforming a sequence of characters into its equivalent representation in the hexadecimal number system.
In this article, we’ll discuss the various ways to convert a string to hexadecimal in Python.
Using the encode()
Method to Convert String to Hexadecimal in Python
In Python, the encode()
method is a string method used to convert a string into a sequence of bytes. It takes an encoding scheme as an argument, such as utf-8
, utf-16
, ascii
, etc., and returns a bytes object containing the encoded representation of the original string.
The result of the encode()
method is a sequence of bytes, which can be further processed using various methods. For example, the hex()
function can be applied to convert each byte into its hexadecimal representation.
However, it’s important to note that hex()
is typically used for integers, so each byte needs to be converted to an integer before applying hex()
. This two-step process allows for the conversion of the original string into a hexadecimal representation using the encode()
and hex()
functions in combination.
Example Code 1:
s = "Sample String".encode("utf-8")
print(s.hex())
Output:
53616d706c6520537472696e67
In the code above, we first assign the string "Sample String"
to the variable s
. Next, we use the encode()
method with the encoding scheme 'utf-8'
to convert the string into a sequence of bytes.
The resulting bytes object is then processed using the hex()
function, which converts each byte to its hexadecimal representation. Finally, we display the hexadecimal representation of the original string.
The output, 53616d706c6520537472696e67
, represents the ASCII or Unicode values of each character in the string "Sample String"
in hexadecimal format. Let’s have another example of using the encode()
method.
Example Code 2:
string_value = "Delftstack"
hex_representation = string_value.encode("utf-8").hex()
print(hex_representation)
Output:
44656c6674737461636b
In this code, we begin by assigning the string "Delftstack"
to the variable string_value
. Using the encode()
method with the 'utf-8'
encoding scheme, we convert the string into a sequence of bytes.
The resulting bytes object is then processed with the hex()
method, efficiently converting each byte to its hexadecimal representation. Finally, we display the hexadecimal representation of the original string.
The output, 44656c6674737461636b
, signifies the ASCII or Unicode values of each character in the string "Delftstack"
presented in hexadecimal format.
Using the binascii
Module to Convert String to Hexadecimal in Python
The binascii
module in Python provides functions for binary-to-text and text-to-binary conversions, including hexadecimal representations. When we need to convert a string to its hexadecimal representation, we utilize the hexlify()
function within the binascii
module.
Example Code:
import binascii
string_value = "Delftstack"
hex_representation = binascii.hexlify(string_value.encode("utf-8")).decode("utf-8")
print(hex_representation)
Output:
44656c6674737461636b
In this code snippet, we start by importing the binascii
module. We then assign the string "Delftstack"
to the variable string_value
.
Next, using the encode()
method with the 'utf-8'
encoding, we convert the string into a sequence of bytes. Then, we apply the hexlify()
function from the binascii
module to efficiently convert the bytes to their hexadecimal representation.
The decode('utf-8')
method is then employed to obtain a readable string from the hexadecimal-encoded bytes. Finally, we display the resulting hexadecimal representation of the original string.
The output, 44656c6674737461636b
, represents the ASCII or Unicode values of each character in the string "Delftstack"
in hexadecimal format.
Using the hex()
Function in Combination With map()
and ord()
to Convert String to Hexadecimal in Python
Again, the hex()
function is typically used to convert integers to a hexadecimal string. If we try to use hex()
directly on a string, we will encounter a TypeError
.
However, by using the map()
and ord()
functions in combination, we can achieve a conversion from a string to its hexadecimal representation.
The map()
function is employed to apply a lambda
function to each character of a given string. This lambda
function utilizes the ord()
function to obtain the ASCII values of the characters.
Subsequently, the hex()
function converts these values to their hexadecimal representations. The result is a concatenated string of hexadecimal values, effectively encoding the original string into its hexadecimal equivalent.
Example Code:
string_value = "Delftstack"
hex_representation = "".join(map(lambda x: hex(ord(x))[2:], string_value))
print(hex_representation)
Output:
44656c6674737461636b
In this code, we initialize the variable string_value
with the string "Delftstack"
.
To convert each character of the string to its hexadecimal representation, we use the map()
function in combination with a lambda function, and this lambda
function applies the ord()
function to obtain the ASCII values of the characters and then uses the hex()
function to convert these values to their hexadecimal representation, with the [2:]
slice removing the '0x'
prefix.
Next, the map()
results are joined into a single string using join()
, creating the hex_representation
. Finally, we display the resulting hexadecimal representation of the original string.
The output 44656c6674737461636b
signifies the ASCII or Unicode values of each character in the string "Delftstack"
represented in hexadecimal format.
Conclusion
In conclusion, this article explored diverse methods for converting a string to its hexadecimal representation in Python. The encode()
method proved fundamental, allowing the transformation of a string into bytes, which could then be processed using functions like hex()
.
The binascii
module offered a streamlined approach through the hexlify()
function. Additionally, the use of the hex()
function in combination with map()
and ord()
showcased an inventive way to achieve string-to-hexadecimal conversion.
Each method was exemplified through clear and concise code snippets, highlighting Python’s versatility in handling this common encoding task.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn