How to Split an Integer Into Digits in Python
- Use List Comprehension to Split an Integer Into Digits in Python
-
Use the
math.ceil()
andmath.log()
Functions to Split an Integer Into Digits in Python -
Use the
map()
andstr.split()
Functions to Split an Integer Into Digits in Python -
Use a
for
Loop to Split an Integer Into Digits in Python - Conclusion
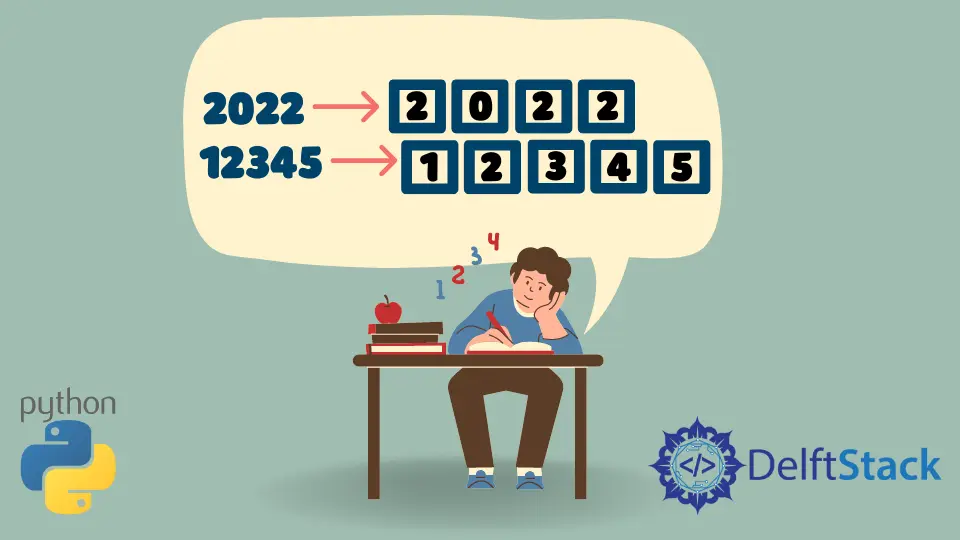
To split integer into digits in Python, it involves breaking down the numeric value for individual manipulation, often used in programming tasks.
This tutorial explores different methods on how to split number into digits python, including list comprehension, math.ceil()
and math.log()
, map()
and str.split()
, and a loop-based approach.
Use List Comprehension to Split an Integer Into Digits in Python
List comprehension is a way to create lists that are to be formed based on given values of an already existing list.
In this method, str()
and int()
functions are also used along with list comprehension to split the integer into digits. The str()
and int()
functions are used to convert a number to a string and then to a given string as an integer, respectively.
Basic Syntax:
x = [int(a) for a in str(num)]
Parameter:
num
: The integer you want to split into its digits. It’s the input value for the list comprehension.
The syntax generates a list by iterating through the characters of the string representation of the integer num
. Applying the int()
function to each individual character then converts them into integers, resulting in list x
containing the individual digits of the original integer, with each character as a separate element.
Code Input:
num = 13579
x = [int(a) for a in str(num)]
print(x)
Output:
In the above code example, the int
value 13579
in the variable num
is converted into a string. Using a list comprehension, we iterate through each character in the string and convert it back to an integer value, creating a list named x
.
The printed output is [1, 3, 5, 7, 9]
.
Use the math.ceil()
and math.log()
Functions to Split an Integer Into Digits in Python
Splitting an integer into digits in Python is faster without converting the number to a string first. Utilizing the math.ceil()
function for rounding and math.log()
for natural logarithms, both accessible through the math module, enhances efficiency.
The math
module is a standard and always accessible module in Python, granting access to fundamental C library functions.
Basic Syntax:
x = [(num // (10**i)) % 10 for i in range(places)]
Parameters:
-
num
: This parameter represents the integer you want to split into its digits. -
i
: This variable is used within the list comprehension to iterate through a range of values, representing the positions of the digits in the integer. -
places
: The places parameter defines the number of digits in the integer, which determines the range ofi
values to consider during the iteration.
The syntax efficiently splits the integer num
into individual digits in Python. It iterates through a range corresponding to each digit’s position and uses the expression (num // (10 ** i)) % 10
to extract each individual digit.
The extracted digits are collected in the list x
, offering a compact method for breaking down an integer into its digits.
Code Input:
import math
n = 13579
x = [(n // (10**i)) % 10 for i in range(math.ceil(math.log(n, 10)) - 1, -1, -1)]
print(x)
Output:
In the above in code example, the math module is imported for mathematical functions and constants. The integer 13579
is assigned to variable n
.
Using a list comprehension, we iterate through indices corresponding to digit positions of individual numbers, calculating each digit’s value and storing them in the list x
. The output represents the individual digits of the original number.
Use the map()
and str.split()
Functions to Split an Integer Into Digits in Python
The map()
function applies a specified function to each item in an iterable, using the item as a parameter.
The split()
method divides a string into a list, requiring two parameters: a separator
and an optional maxsplit
parameter.
The number needs to be already in the string format so that this method can be used.
Basic Syntax:
list1 = str1.split()
listofint = list(map(int, list1))
The parameter in the 1st line list1 = str1.split()
:
str1
: The input string that you want to split into a list. It contains space-separated numbers.
The parameters in the next line listofint = list(map(int, list1))
:
-
int
: A built-in function used for converting a string to an integer. -
list1
: The list of strings obtained after splitting the input string using thesplit()
method.
The second line in the following code above uses the map()
and int()
functions to convert the string list to an integer list.
Code Input:
str1 = "1 3 5 7 9"
list1 = str1.split()
map_object = map(int, list1)
listofint = list(map_object)
print(listofint)
Output:
In the above code, the string str1 = "1 3 5 7 9"
is split into individual number strings using the split()
method, forming a list called list1
. Applying the int
function through a map_object
converts these strings to integers, resulting in the listofint
.
The printed output displays the integers from the original string in an organized list.
Use a for
Loop to Split an Integer Into Digits in Python
In this method, we use a loop and perform the slicing technique till the specified number of digits (A=1
, in this case), and then finally, use the int()
function for conversion into an integer.
Code Input:
str1 = "13579"
A = 1
result = []
for i in range(0, len(str1), A):
result.append(int(str1[i : i + A]))
print("The resultant list : " + str(result))
Output:
In the above code, starting with the string str1 = "1 3 5 7 9"
, we use a for
loop to extract individual digits. A substring of length 1
is converted to integers and added to the result list.
The output message demonstrates the efficient separation of the string into its constituent digits in list form.
Conclusion
This article explores four methods on how to split a number into digits python. It covers string conversion with list comprehension, a mathematical approach using math.ceil()
and math.log()
, working with string-format integers using map()
and str.split()
, and a loop-based method.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn