Soundex in Python
-
Introduction to
soundex
in Python -
Use
soundex
in Python -
Things the
soundex
Function Ignores -
Rules of
soundex
in Python - Conclusion
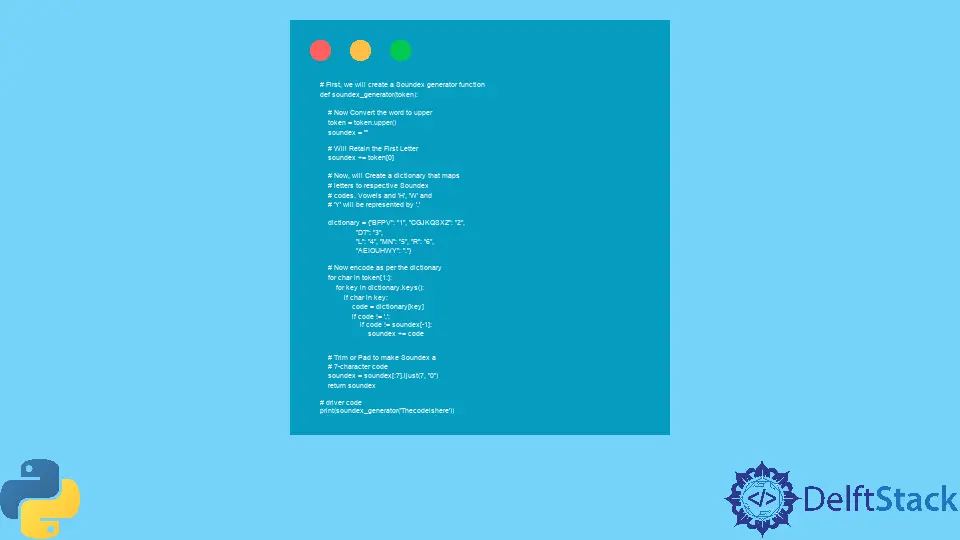
The soundex
function for Python is a function that converts a string of text into a Soundex code. It is helpful for indexing names in databases or finding similar ones.
The Soundex code for a name is based on how it sounds, not how it is spelled. It is a handy tool for comparing words pronounced differently but with the exact spelling.
It is beneficial when searching for names in databases.
Introduction to soundex
in Python
The soundex
function is a simple phonetic algorithm used to encode strings. The algorithm is designed to produce codes similar to those produced by the Soundex system.
The function is written in Python and can be easily implemented in any Python project. The soundex
function takes a string as input and returns a code based on the sound of the string.
It assigns a code to each word based on how it is pronounced, so words pronounced differently but with the same code are considered equivalent. It can be a big help when searching for names that may be spelled differently but are pronounced the same.
It can be useful when you compare words that are pronounced differently but have the exact spelling. For example, cat
and bat
have the same soundex
code.
Use soundex
in Python
The soundex
function in Python takes a string as an input and outputs a code representing the sound of the string. The code is based on how the string sounds, not spelling.
It makes it helpful in matching names pronounced differently but with the same sound.
The soundex
process is a means of indexing names by sound. It is a simple phonetic algorithm for indexing words by sound, as pronounced in English.
The goal is to produce a Soundex code that captures the name’s essential sound while disregarding spelling differences.
To use the soundex
function, you first need to import the module that contains it; then, you can call the function with a string as an argument. The function will return the Soundex code for the string.
The Soundex code is a four-digit code used to represent a name’s pronunciation. The code is derived from the first letter of the name, followed by three numbers representing the name’s three consonants.
The code is designed to be pronounceable so that words with similar pronunciations will have the same code.
Example Code:
# First, we will create a Soundex generator function
def soundex_generator(token):
# Now Convert the word to upper
token = token.upper()
soundex = ""
# Will Retain the First Letter
soundex += token[0]
# Now, will Create a dictionary that maps
# letters to respective Soundex
# codes. Vowels and 'H', 'W' and
# 'Y' will be represented by '.'
dictionary = {
"BFPV": "1",
"CGJKQSXZ": "2",
"DT": "3",
"L": "4",
"MN": "5",
"R": "6",
"AEIOUHWY": ".",
}
# Now encode as per the dictionary
for char in token[1:]:
for key in dictionary.keys():
if char in key:
code = dictionary[key]
if code != ".":
if code != soundex[-1]:
soundex += code
# Trim or Pad to make Soundex a
# 7-character code
soundex = soundex[:7].ljust(7, "0")
return soundex
# driver code
print(soundex_generator("Thecodeishere"))
Output:
T232600
Things the soundex
Function Ignores
The soundex
process ignores non-alphabetic characters in a name, so punctuation and spaces are not considered.
In addition, soundex
only codes the first letter of a surname if it is a consonant, so surnames that begin with vowels are not coded.
Finally, soundex
codes names according to their pronunciation, so variants of the same name that are pronounced differently (such as Smith and Smyth) will have different codes.
Rules of soundex
in Python
However, the Soundex process has several rules.
- First, it is not well-suited for indexing names from languages other than English since the
soundex
code is based on English phonetics. - Second, the
soundex
code for a given name can vary depending on the English dialect used. For instance, the nameSmith
would be coded asS530
in General American English but asS520
in Southern English. - Third, the Soundex process can sometimes produce ambiguous results, especially when two or more names have the same
soundex
code. For instance, the namesSmith
andSmythe
both have thesoundex
codeS530
, making it challenging to find a specific name when using a Soundex-based index. - Fourth, the Soundex process is not very effective at indexing names that are pronounced differently than spelled. For instance, the name
Stevenson
is pronouncedSTEH-ven-son
, but it would be coded asS315
based on its spelling, making it challenging to find a name when using a Soundex-based index.
Overall, the Soundex process is a simple and effective means of indexing names by sound. However, it has several limitations that should be considered when using it.
Conclusion
To conclude the article on soundex
in Python, we’ve discussed the working and rules of soundex
. Furthermore, we have discussed a practical example demonstrating the working of soundex
in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn