How to Set Attributes of a Class in Python
-
Use the
setattr()
Function to Set Attributes of a Class in Python - Where to Use the Set Attribute in Python
-
How to Use the
setattr()
to Add a New Attribute to a Class in Python - Why Does Using Set Attribute Inside a Class Cause Recursion in Python
- How Can I Use Set Attribute Properly to Avoid Infinite Recursion Errors in Python
-
How to Fix the Recursion Error in
MicroPython
- Conclusion
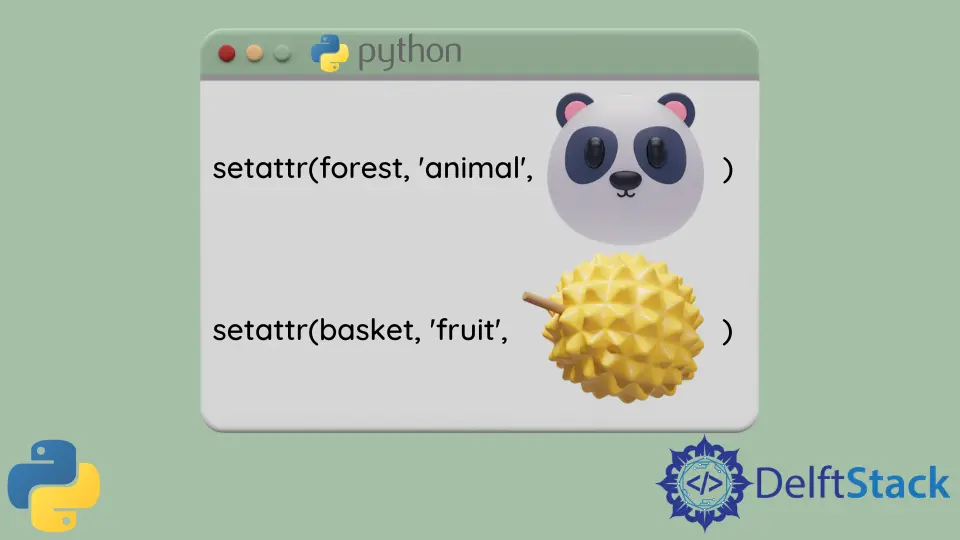
Python’s setattr()
method comes from PEP383 and allows it to control and access attributes dynamically to alter their values.
Use the setattr()
Function to Set Attributes of a Class in Python
Python’s setattr()
function is used to set values for the attributes of a class. In programming, where the variable name is not static, the setattr()
method comes in very handy as it provides ease of use.
Syntax of Set Attribute in Python
The set attribute technique serves as an alternative to utilizing constructors and object methods. It can be used for passing arguments to attributes by executing setattr()
when an attribute allocation is made.
The syntax for the set attribute is below.
setattr(self, name, value)
The above syntax specifies three things which are the following.
self
- The object’s name whose attribute you are assigning.name
- The assigned variable’s name.value
- The assigned variable’s value.
The set attribute returns None
. Note that you can only provide strings as the name for an attribute.
Where to Use the Set Attribute in Python
Let us demonstrate the usage of setattr()
with a class of baskets
and use the setattr()
method to provide its values.
class Baskets:
fruit = "Plum"
bas = Baskets()
print("Old fruit: ")
print(bas.fruit)
setattr(bas, "fruit", "Apple")
print("Fruit Now is: ")
print(bas.fruit)
Output:
Old fruit:
Plum
Fruit Now is:
Apple
The Baskets
class has a fruit. The setattr()
function has been called once to alter the basket’s fruit.
How to Use the setattr()
to Add a New Attribute to a Class in Python
If you want to add a new field to the class, you may use the setattr()
method with the same syntax.
The setattr()
adds a new variable in an existing class and sets the stated data when the given variable doesn’t occur in the class.
class Baskets:
fruit = "Banana"
vegetable = "Potato"
bas = Baskets()
# Adding candy to the basket
setattr(bas, "candy", "Kit Kat")
print("Added:", bas.candy)
Output:
Added: Kit Kat
Even if we remove an attribute from the above code, we can still add it afterward using setattr()
.
Different Syntaxes for Set Attribute in Python
The setattr(object,name,value)
is the shorter syntax used instead of using the object.__setattr__(name, value)
, making both methods the same.
The object.__setattr__()
is inside class definition and uses super()
to get to the next attribute.
The syntax above is not valid in two cases.
- The object already has a predefined dictionary
__setattr__
method, caused by errors. - The object’s class also has a
__getattr__
(Get Attribute) method. The__getattr__
method obstructs all searches regardless of the attribute’s presence.
Why Does Using Set Attribute Inside a Class Cause Recursion in Python
The aim is to perform the code when calling a routine whenever a member parameter is created and set the member parameter.
All updations for deleted values and assignment of attributes change the instance dictionary and not the actual class.
The __setattr__
method is called directly to update the instance dictionary if it has been set in this case.
The problem arises if you use the following syntax: self.name = value __setattr__()
to write to an instance variable because that would be a recursive operation. It leads to a recursion error in Python.
How Can I Use Set Attribute Properly to Avoid Infinite Recursion Errors in Python
Sometimes using __setattr__
can lead to coding errors like infinite recursion loops.
If you want to avoid using interlaced classes while defining classes containing read and write methods, you can overwrite the __setattr__
method.
However, as explained above, the __init__
function might lead to unwanted recursion conflicts. It is because __setattr__
is continuously invoked.
class StAttrTest(object):
def __init__(self, run1):
self.run1 = run1
# setattr method with if else
def __setattr__(self, name, value):
if name == " Lenn1 ":
print("Lenn1 test")
else:
setattr(self, name, value)
cm = StAttrTest(1)
This code runs when you execute the above program but gets trapped in an unending recursion fault.
There are various methodologies for eliminating an endless recurrence loop.
- Add the
@property
decorator inside the class definition, as demonstrated below.
@property
def device(self):
return self
Python provides the @property
functionality to access instance variables like public variables while utilizing existing getters and setters to confirm updated values and prevent explicit access or data manipulation.
- There is a quick fix to avoid the problem by removing the example attribute in the
__init__
method.
class AttrTest(object):
# init without extra attribute
def __init__(self):
self.device = self
The above method fixes the issue but is not a good practice and just a quick fix.
- You can also use an object directly under the set attribute method.
object.__setattr__(self, name, value)
MicroPython
sometimes does not accept the above solutions, so a different methodology is needed. MicroPython
is a reimplementation that strives to be as consistent as feasible, allowing developers to quickly move code from the computer to a microprocessor or integrated device.
If you are an advanced coder, you can follow the following fix to the recursive errors that arise with the set attribute method.
How to Fix the Recursion Error in MicroPython
This method involves alternating the object’s dictionary value using the object.__dict__
. The technique consists of updating the dictionary value of the object within the __setattr__
method.
class StAtrTest(object):
# setattr with object's dictionary overridden
def __setattr__(self, name, value):
print(name, value)
self.__dict__[name] = value
def __init__(self, var_O):
self.var_O = var_O
# Testing
up = StAtrTest(var_O=17)
# Changing
up.var_O = 218
Output:
var_O 17
var_O 218
The method as described here is often considered the most effective technique for resolving endless recursion faults in Python and MicroPython
.
Conclusion
This informative guide discussed using Python’s set attribute method, an excellent method of writing attributes in a dictionary’s instance, and updating class variables for concise coding.
The text provides various effective ways of using the __setattr__
in Python so that you are not caught in an infinite recursion error while designing classes with reading and writing methods. It also highlights the difference between setattr()
and __setattr__
methods.
Remember, the best and recommended method is the usage of the object.__dict__
.