How to Send UDP Packet in Python
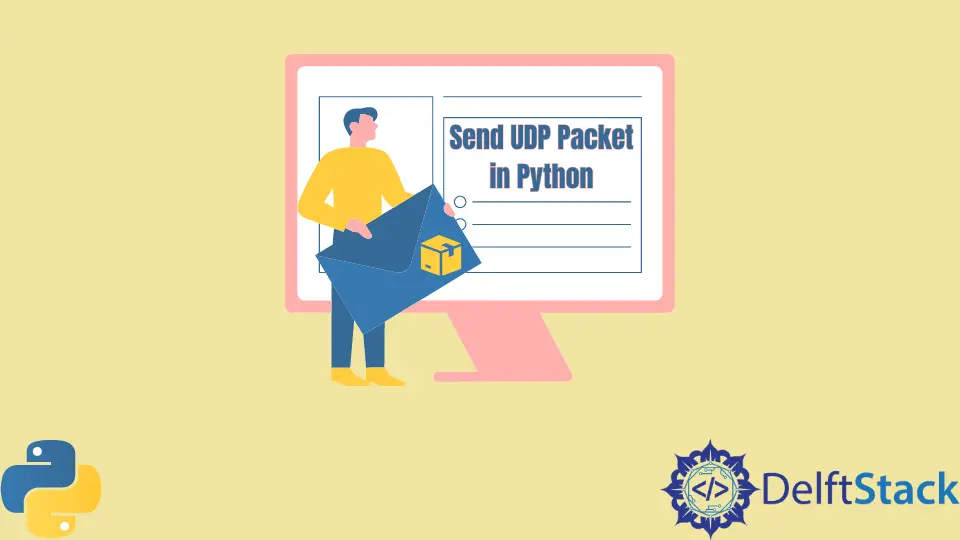
Today, we will learn about User Datagram Protocol (UDP) and see how we can send UDP packets using Python programming.
Send UDP Packet in Python
The User Datagram Protocol (UDP) and TCP/IP operate differently. UDP is a message-oriented protocol, as opposed to TCP, which is a stream-oriented protocol that ensures that all data is transferred in the proper sequence.
Setting up a UDP socket is a little easier since UDP does not require a connection that will last for a long time. On the other hand, unlike TCP, delivery of UDP messages is not assured, and they must fit within a single packet.
How can we do it in Python? Python has a built-in module called socket
that you have to import.
import socket
Once we have imported the socket
module, we must state the port number and IP address to which we will attempt to send UDP messages.
IP = "127.0.0.1"
PORT = 5003
Message = "Info"
It’s time to build the socket
via which we will send our UDP message to the server now that we have stated these few variables.
Sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
Sock.sendto(Message, (IP, PORT))
You have to create two files; client.py
and server.py
. Both the client and server files must be in the corresponding server and client systems.
Once the client program has been created, the next step is to make the server program, which will monitor the specified IP address and port number for any UDP packets.
Note that the client Python script must execute before this server otherwise, the client Python script will fail.
We can create another socket
that will precisely resemble the socket
we made in our client application once we’ve imported the socket
module and provided our IP address and port number.
Example Code for Server.py
File:
import socket
IP = "127.0.0.1"
PORT = 5003
MESSAGE = "The meeting is From 10 pm."
print(f"sending information")
print(f"{MESSAGE} to {IP}:{PORT}")
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.sendto(MESSAGE.encode(), (IP, PORT))
Output:
sending information
The meeting is From 10 pm. to 127.0.0.1:5003
You should also write the code to maintain your script continually listening to this connection till it terminates once you have built your server socket
.
It takes the form of a straightforward while
loop, as demonstrated below:
Example Code for Client.py
File:
import socket
IP = "127.0.0.1"
PORT = 5003
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.bind((IP, PORT))
print(f"start listening to {IP}:{PORT}")
while True:
data, addr = sock.recvfrom(1024)
print(f"received message: {data}")
Output:
start listening to 127.0.0.1:5003
received message: The meeting is From 10 pm.
In this way, we can send UDP packets in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn