How to Use SCP Protocol in Python
- Use the SCP Module to Use SCP Protocol in Python
-
Use the
subprocess.run()
Function to Use SCP Protocol in Python
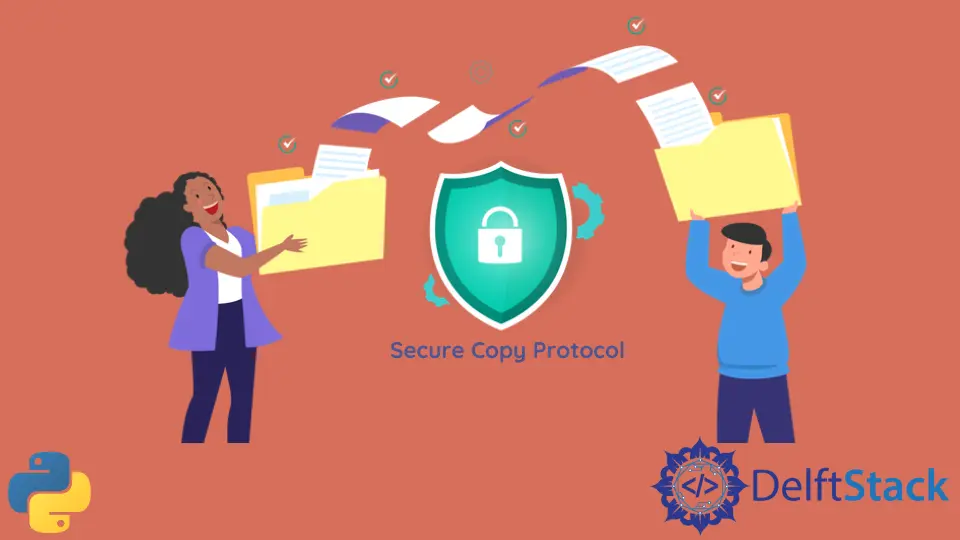
The SCP, Secure Copy Protocol, safely moves files from remote servers to hosts and vice-versa. This protocol is based on the SSH protocol.
The SCP protocol uses this Secure Shell (SSH) to transfer files securely over a network with proper authentication from all endpoints.
This tutorial will demonstrate how to share and receive files using the SCP protocol in Python.
Use the SCP Module to Use SCP Protocol in Python
The SCP module in Python can transfer the files using the SCP1 protocol. The SCP1 protocol uses SSH1 for single file transfer.
The SCP module uses the Paramiko library to securely connect remote hosts and servers using SSH.
Let us see an example of uploading and retrieving files using SCP in Python.
from paramiko import SSHClient
from scp import SCPClient
ssh_ob = SSHClient()
ssh_ob.load_system_host_keys()
ssh_ob.connect("sample.com")
scp = SCPClient(ssh_ob.get_transport())
scp.put("sampletxt1.txt", "sampletxt2.txt")
scp.get("sampletxt2.txt")
scp.put("sample", recursive=True, remote_path="/home/sample_user")
scp.close()
We will now analyze what is happening in the above code.
We use the paramiko.SSHClient
class to create a secure connection to an SSH server. The scp.SCPClient
returns an object by taking the transport of the SSH connection, which is returned using the get_transport()
function.
We upload the files to the server using the scp.put()
function and retrieve files using the scp.get()
function. After transferring files, we close the connection using the close()
function.
We can also use the putfo()
function to upload file-like objects using the SCP module.
Example:
import io
from paramiko import SSHClient
from scp import SCPClient
ssh = SSHClient()
ssh.load_system_host_keys()
ssh.connect("sample.com")
scp = SCPClient(ssh.get_transport())
f = io.BytesIO()
f.write(b"content")
f.seek(0)
scp.putfo(f, "/sample_dir/sampletxt.txt")
scp.close()
fl.close()
In the above example, we used the io.BytesIO()
constructor to create an in-memory file object. We wrote some data in bytes and uploaded it to a text file using the scp.putfo()
function.
Use the subprocess.run()
Function to Use SCP Protocol in Python
The subprocess module can run bash commands remotely from a Python program. We can use the subprocess.run()
function to run a given command.
We can use the SCP bash command to transfer files using SSH. This can be executed using the subprocess.run()
function discussed earlier.
Example:
import subprocess
subprocess.run(["scp", "sample.txt", "user@server:/path/sample.txt"])
We transferred a text file using the SCP command in the above example. We need to generate the SSH key on the source and install it on the destination beforehand to authenticate SCP with your key.
The subprocess.run()
function was introduced in Python 3.5. We can also use other functions to run the SCP bash command like the subprocess.Popen()
, os.system()
, and more.
In conclusion, we can use the SCP protocol with Python by using the SCP module or running the bash command SCP from different functions. It is crucial to have the necessary keys for authentication for transferring files.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn