How to Read PDF in Python
- Method 1: Using PyPDF2
- Method 2: Using pdfplumber
- Method 3: Using PyMuPDF
- Method 4: Using pdfminer.six
- Conclusion
- FAQ
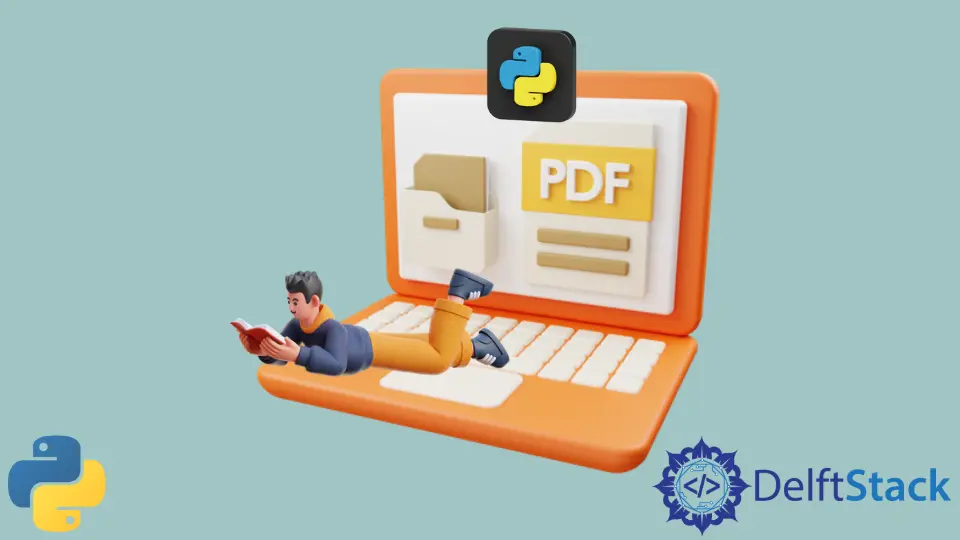
Reading PDF files in Python can be an essential skill for developers and data analysts alike. Whether you’re extracting text for data analysis, processing forms, or even just reading reports, knowing how to handle PDFs efficiently can save you a lot of time and effort.
In this tutorial, we will explore various methods to read PDFs in Python using popular libraries. We’ll cover everything from installation to practical code examples, ensuring you have a solid understanding of how to work with PDF files in your projects. Let’s dive in!
Method 1: Using PyPDF2
One of the most popular libraries for reading PDFs in Python is PyPDF2. This library allows you to extract text and metadata from PDF files easily. To get started, you first need to install the library using pip:
pip install PyPDF2
Once installed, you can start reading PDF files. Here’s a simple example to demonstrate how to extract text from a PDF file:
import PyPDF2
pdf_file = open('sample.pdf', 'rb')
reader = PyPDF2.PdfReader(pdf_file)
text = ''
for page in reader.pages:
text += page.extract_text()
pdf_file.close()
print(text)
Output:
This is an example of text extracted from a PDF file.
In this code, we open the PDF file in binary mode and create a PdfReader
object. We then loop through each page of the PDF, extracting the text using the extract_text()
method. Finally, we close the file and print the extracted text. PyPDF2 is particularly useful for simple text extraction tasks, but its capabilities extend to handling metadata and merging multiple PDFs as well.
Method 2: Using pdfplumber
If you need more advanced features for reading PDFs, pdfplumber is an excellent choice. This library provides more control over the text extraction process, allowing you to handle complex layouts and tables effectively. To install pdfplumber, run the following command:
pip install pdfplumber
Here’s how to use pdfplumber to extract text from a PDF:
import pdfplumber
with pdfplumber.open('sample.pdf') as pdf:
text = ''
for page in pdf.pages:
text += page.extract_text()
print(text)
Output:
This is an example of text extracted from a PDF file using pdfplumber.
In this example, we utilize a context manager to open the PDF file, which ensures that the file is properly closed after we’re done. The extract_text()
method from pdfplumber works similarly to PyPDF2 but often yields better results with more complex PDF layouts. This makes pdfplumber a great option when dealing with PDFs that contain tables or non-standard formatting.
Method 3: Using PyMuPDF
Another powerful library for reading PDFs in Python is PyMuPDF (also known as fitz). This library is highly efficient and can handle a wide variety of PDF files. To install PyMuPDF, use the following command:
pip install PyMuPDF
Here’s a sample code snippet that demonstrates how to read a PDF using PyMuPDF:
import fitz
pdf_document = fitz.open('sample.pdf')
text = ''
for page_num in range(len(pdf_document)):
page = pdf_document[page_num]
text += page.get_text()
pdf_document.close()
print(text)
Output:
This is an example of text extracted from a PDF file using PyMuPDF.
In this code, we open the PDF document and iterate through each page using its index. The get_text()
method fetches the text from each page. PyMuPDF not only allows text extraction but also provides features for rendering pages, adding annotations, and manipulating PDFs, making it a versatile choice for more complex operations.
Method 4: Using pdfminer.six
For those who need to extract text with high accuracy, especially from PDFs with intricate layouts, pdfminer.six is a robust option. This library is designed for detailed text extraction and is particularly useful for analyzing PDFs with complex structures. To install pdfminer.six, use:
pip install pdfminer.six
Here’s how to extract text using pdfminer.six:
from pdfminer.high_level import extract_text
text = extract_text('sample.pdf')
print(text)
Output:
This is an example of text extracted from a PDF file using pdfminer.six.
In this example, we use the extract_text
function from the pdfminer.high_level
module, which simplifies the text extraction process. This library excels in scenarios where accuracy and layout preservation are crucial. While it may require a steeper learning curve compared to others, the results are often worth the effort, especially for professional applications.
Conclusion
Reading PDF files in Python opens up a world of possibilities for data extraction and analysis. Whether you choose PyPDF2 for its simplicity, pdfplumber for its advanced features, PyMuPDF for its versatility, or pdfminer.six for high accuracy, each library has its unique strengths. By mastering these tools, you can efficiently handle PDF data in your projects and enhance your overall productivity. Now that you have a solid understanding of how to read PDFs in Python, you can explore these libraries further and find the one that best suits your needs.
FAQ
-
What is the best library for reading PDFs in Python?
The best library depends on your needs. PyPDF2 is great for basic tasks, pdfplumber excels with complex layouts, PyMuPDF offers versatility, and pdfminer.six is best for high accuracy. -
Can I extract images from PDF files using these libraries?
Yes, some libraries like PyMuPDF allow you to extract images, while others focus primarily on text extraction. -
Is it possible to edit PDF files using Python?
Yes, libraries like PyMuPDF and PyPDF2 can be used to edit PDFs, such as merging or modifying content. -
Are these libraries free to use?
Yes, all the libraries mentioned in this article are open-source and free to use. -
How do I handle encrypted PDF files in Python?
Some libraries, like PyPDF2, can handle encrypted PDFs if you provide the correct password.