How to Get the Running Time of Python Code
-
Advantages of
timeit
: -
Use the
timeit
Module to Compare the Execution Time of Two Snippets -
Use the
timeit
Module’s Command-Line Interface
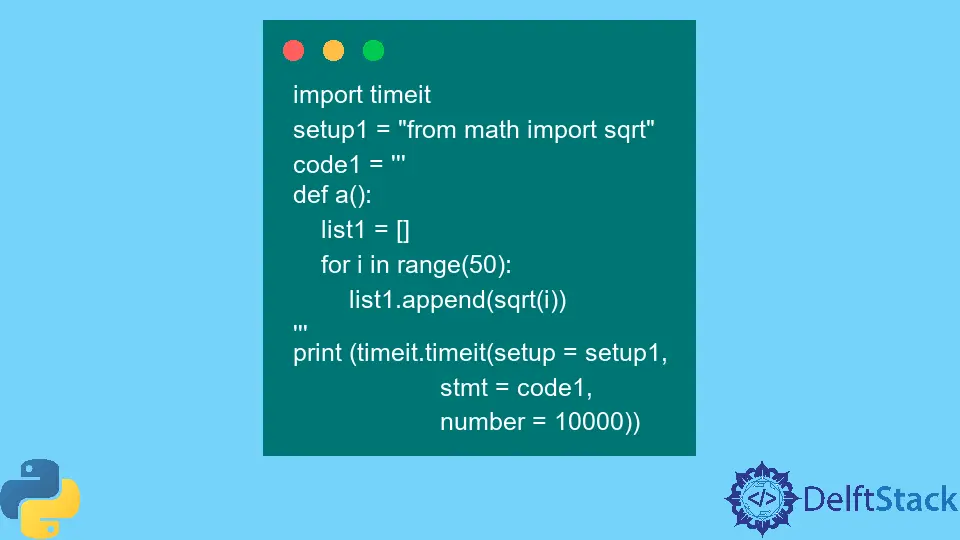
The timeit
module is one of the simplest and easiest to implement modules among all the category tools that profile the execution of the Python code. The tutorial discusses the use and implementation of the timeit
module in Python.
Python contains a built-in library timeit
that can compute the execution time of tiny snippets of the Python code. The module works by running the given python code millions of times and predicts the least time elapsed during the execution of that code from all the possible results.
Advantages of timeit
:
- Provides an efficient result with precision. The accuracy of the execution time rendered through this function is simply higher than by utilizing the simple
time
module. - It runs the given code snippet over a million times, which offers much more relevant readings of the time that elapsed during its runtime.
- It further has an added command-line interface apart from the generic callable interface, which is easy to understand and apply.
Moving on, let us depict and focus on the syntax of the timeit()
function from the timeit
module.
timeit.timeit(stmt, setup, timer, number)
The timeit.timeit()
function takes in four parameters, all of which have been briefly explained for ease of understanding the function below:
stmt
: As the name suggests, it is the statement that needs to be measured. The default value ispass
in this case.setup
: Signifies the code that must run before thestmt
is tackled. Once again, it has a default aspass
.timer
: A basictimeit.Timer
object. It already has a decent default value assigned to it, which does not usually need a change.number
: Signifies the number of runs of the given code snippet that the programmer would like to run for.
We will see different examples of how the timeit.timeit()
function from the timeit
module can be implemented.
Use the timeit
Module for Calculating the Execution Time of a Simple Code Snippet
This method defines a code snippet in a variable in between triple quotes (''')
.
The following code uses the timeit
module for calculating the execution time of a simple code snippet.
import timeit
setup1 = "from math import sqrt"
code1 = """
def a():
list1 = []
for i in range(50):
list1.append(sqrt(i))
"""
print(timeit.timeit(setup=setup1, stmt=code1, number=10000))
The above program provides the output as the execution time taken in seconds. This execution time results from a million iterations of the given code snippet.
We should also note that it is good to involve all the essential import statements in the setup argument.
Use the timeit
Module to Compare the Execution Time of Two Snippets
The timeit
module can also be utilized for comparing the execution time of two given snippets of code. Here, we will take two functions used for Binary search
and Linear Search
respectively and compare their execution times.
The following code uses the timeit
module to compare the execution time of two snippets.
import timeit
# function for binary search
def b_s(mylist, find):
while len(mylist) > 0:
mid = (len(mylist)) // 2
if mylist[mid] == find:
return True
elif mylist[mid] < find:
mylist = mylist[:mid]
else:
mylist = mylist[mid + 1 :]
return False
# function for linear search
def l_s(mylist, find):
for x in mylist:
if x == find:
return True
return False
def b_time():
SETUP_CODE = """
from __main__ import b_s
from random import randint"""
TEST_CODE = """
mylist = [x for x in range(10000)]
find = randint(0, len(mylist))
b_s(mylist, find)"""
times = timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)
print("Binary search time: {}".format(min(times)))
def l_time():
SETUP_CODE = """
from __main__ import l_s
from random import randint"""
TEST_CODE = """
mylist = [x for x in range(10000)]
find = randint(0, len(mylist))
l_s(mylist, find)
"""
times = timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)
print("Linear search time: {}".format(min(times)))
if __name__ == "__main__":
l_time()
b_time()
Use the timeit
Module’s Command-Line Interface
Let us take a simple function code and its syntax in the command-line interface and tackle all the arguments one at a time.
The following code uses the timeit
module’s command-line interface.
C:\Windows\System32>python3 -m timeit -s "from math import sqrt" -n 10000 -r 5 'a = sqrt(34532)'
Here, we have taken the same function as the first example in the article.
Moving on, let us consider all the arguments used herein the command-line interface.
timeit
: A simple positional argument that specifies the existence and use of thetimeit
module.-s
: The setup argument.-n
: The number argument.-r
: The repeated argument (optional).'code snippet'
: The code snippet is written directly within single quotes in the command-line interface.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn