How to Configure Logging to Syslog in Python
-
Log Messages to
syslog
Using theSyslog
Module in Python -
Log Messages to
Syslog
Using thelogging
Module in Python
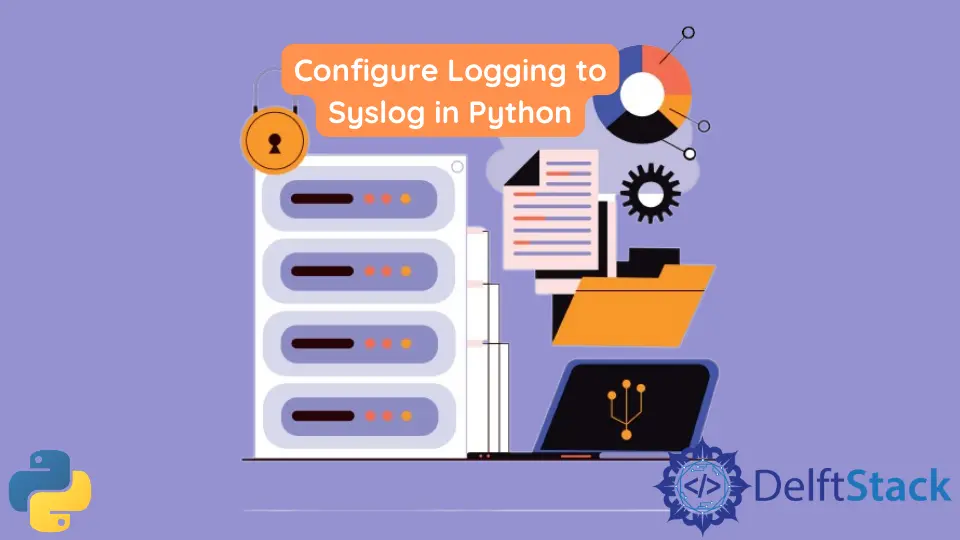
System Logging Protocol or Syslog
is software for Unix-based operating systems such as macOS and Linux that is a standard for message logging.
Syslog
is a standard network-based logging protocol that allows us to send system log and event messages to a server, known as the Syslog
server. This service collects log messages and event messages from several different independent machines at one place for analysis, monitoring, and assessment.
Let us understand this service with the help of an example.
Suppose you have five computers. One of these five computers is a Syslog
server. The other four computers are servers that are hosting four web applications. These four web applications are independent of each other and generate some log messages.
These log messages include details about the users that logged in, users that logged out, what page was accessed by which user, what error was found at what page, how many times was a specific page accessed in the last hour, how many online transactions took place in the last hour, and so on.
Since there are so many applications and log messages, hopping onto individual computers and reviewing them is a complex task because the number of applications can be even more. So, all these applications log their messages to the fifth computer or the Syslog
server.
Suppose someone wishes to review and monitor these log messages from several applications. In that case, they can do that from one place.
This strategy not only makes reviewing easier but also efficient and productive. One can even develop applications to review and monitor these log messages based on their taste and requirements or use existing products available in the market.
Python programming language is a universal language that can build real-world applications. This article will tackle logging messages to Syslog
using Python.
Log Messages to syslog
Using the Syslog
Module in Python
We can log messages using a Python module, syslog
. It is a part of the Python standard library and acts as an interface for the Unix syslog
library routines.
This module has two methods with the same name, syslog()
, that can log messages. Here, the concept of function overloading is used.
Following is the function signature for both methods.
syslog.syslog(message)
syslog.syslog(priority, message)
The first method accepts a string message, and the second method takes a string message along with a priority. Both the methods send a string message to the system logger.
There are eight priority levels available in this module which are as follows in a high to low order.
LOG_EMERG
-syslog.LOG_EMERG
: Used for emergencies.LOG_ALERT
-syslog.LOG_ALERT
: Used for alerts.LOG_CRIT
-syslog.LOG_CRIT
: Used for critical messages.LOG_ERR
-syslog.LOG_ERR
: Used for errors.LOG_WARNING
-syslog.LOG_WARNING
: Used for warnings.LOG_NOTICE
-syslog.LOG_NOTICE
: Used for notices.LOG_INFO
-syslog.LOG_INFO
: Used for informational messages.LOG_DEBUG
-syslog.LOG_DEBUG
: Used for debugging messages.
We are now done with some concise introduction to the module. Let us understand how to use this module with the help of an example. Refer to the following Python code for this.
import syslog
syslog.syslog("A test message.")
syslog.syslog(syslog.LOG_EMERG, "A message with LOG_EMERG priority.")
syslog.syslog(syslog.LOG_ALERT, "A message with LOG_ALERT priority.")
syslog.syslog(syslog.LOG_CRIT, "A message with LOG_CRIT priority.")
syslog.syslog(syslog.LOG_ERR, "A message with LOG_ERR priority.")
syslog.syslog(syslog.LOG_WARNING, "A message with LOG_WARNING priority.")
syslog.syslog(syslog.LOG_NOTICE, "A message with LOG_NOTICE priority.")
syslog.syslog(syslog.LOG_INFO, "A message with LOG_INFO priority.")
syslog.syslog(syslog.LOG_DEBUG, "A message with LOG_DEBUG priority.")
syslog.syslog(syslog.LOG_INFO, "Test message with INFO priority.")
Log Messages to Syslog
Using the logging
Module in Python
Python has yet another module, logging
, containing methods and classes that assist applications and libraries with a flexible event logging system.
This module is also a part of the Python standard library. The logging
module provides many features and flexibility for event logging.
The logging
module has a class Logger
that contains the implementation of the actual logging logic. Developers and programmers have to instantiate this class to perform logging.
Note that this class should never be instantiated directly. One must use a module-level function getLogger()
or logging.getLogger(name)
to create a logger.
Here, name
is the name of the logger. The Logger
class has a bunch of methods such as propogate()
, setLevel()
, isEnabledFor()
, getEffectiveLevel()
, and log()
.
For the sake of this article, we will only focus on the following methods.
debug(msg)
: A method to log messages with levelDEBUG
.info(msg)
: A method to log messages with levelINFO
.warning(msg)
: A method to log messages with levelWARNING
.error(msg)
: A method to log messages with levelERROR
.critical(msg)
: A method to log messages with levelCRITICAL
.log(level, msg)
: A method to log messages with the specified integer level.exception(msg)
: A method to log messages with levelERROR
.addHandler(hdlr)
: A method to add a specified handler to the logger.
This library has the following logging levels along with their numeric values.
CRITICAL
:50
ERROR
:40
WARNING
:30
INFO
:20
DEBUG
:10
NOTSET
:0
The logging module has a class Handler
that is used to specify a location for log messages. A Handler
class object is added to a logger with the help of the addHandler()
method discussed above.
Apart from these two classes, the logging
module has two more classes, namely, Formatter
and Filter
. The Formatter
class is used to define the layout and format of log messages, and the Filter
class is used for filtering log messages.
For now, that is all we have to know about these features.
Now we are done with some concise introduction to the logging
module; let us understand how to use this module to log messages with the help of an example. Refer to the following Python code for this.
import logging
from logging.handlers import SysLogHandler
logger = logging.getLogger("Logger")
handler = SysLogHandler(address="/var/run/syslog")
logger.addHandler(handler)
logger.debug("A message with level DEBUG.")
logger.info("A message with level INFO.")
logger.warning("A message with level WARNING.")
logger.error("A message with level ERROR.")
logger.critical("A message with level CRITICAL.")
logger.exception("A message with level ERROR.")
logger.log(250, "A message with level 250 numeric value.")
The Python script above first creates a logger with the help of the getLogger()
method and provides the name Logger
.
Next, it creates a handler using the SysLogHandler
class. This class allows developers and programmers to send log messages to a remote or local Unix syslog
.
This class accepts a parameter (one of the three parameters), address
, which refers to the log messages’ destination. To learn about this class in detail, refer to the official documentation here.
Then, we add this handler to the logger with the help of the addHandler()
method. Lastly, using all the logging methods discussed above, we log messages of all the levels and one with the 250
level.
To learn about the logging
module and syslog
module, refer to the offical documentation here and here respectively.