How to Swap Two Values in Python
- Swap Two Values Using Tuple Swap in Python
- Swap Two Values Using a Temporary Variable in Python
- Swap Two Values Using XOR Logic in Python
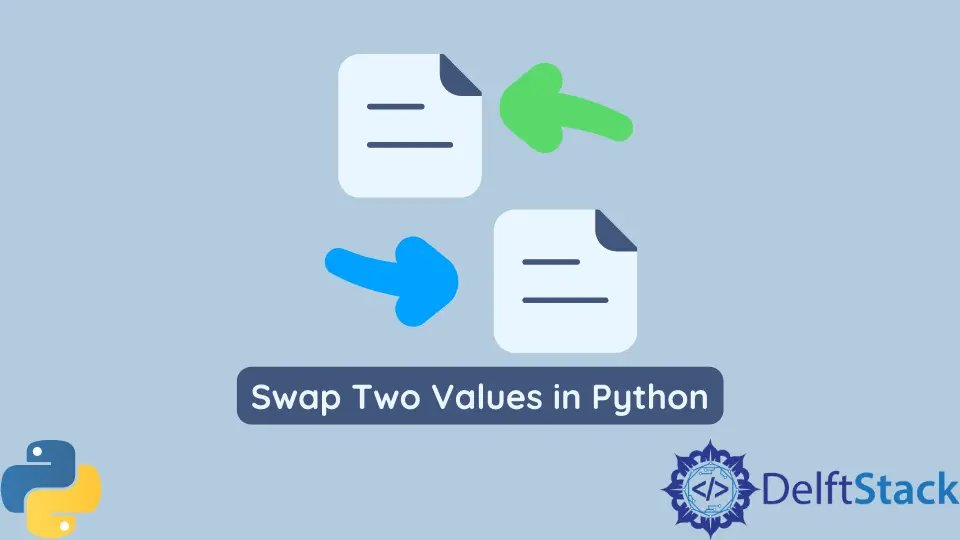
When dealing with data or programming in general, we land up in situations where we have to swap the values of two variables. For example, if a
is storing 5
and b
is storing 25
, a
will store 25
after swapping, and b
will store 5
.
This article will talk about different ways that we can use to perform swapping of values between two variables.
Swap Two Values Using Tuple Swap in Python
In Python, we can use an assignment expression or tuple swap to swap to values. Refer to the following code.
a = 5
b = 25
print("Before swapping")
print("a:", a, "b:", b)
a, b = b, a # Swapping
print("After swapping")
print("a:", a, "b:", b)
Output:
Before swapping
a: 5 b: 25
After swapping
a: 25 b: 5
Notice the expression a, b = b, a
. What this expression essentially does is to create a tuple of two values on the right side and then on the left side. Next, the first value of the tuple on the right side is assigned to the first variable of the tuple on the left side. Lastly, the second value of the tuple on the right side is assigned to the second variable of the tuple on the left side. This operation swaps the two values.
Swap Two Values Using a Temporary Variable in Python
In this method, a temporary variable is used to swap two values. Consider two variables, a
and b
and a temporary variable, temp
. First, the value of a
will be copied to temp
. Then the value of b
will be assigned to a
. Lastly, the value of temp
will be assigned to b
, and the temp
variable will be either deleted or set to None
.
Refer to the following code for the same.
a = 5
b = 25
print("Before swapping")
print("a:", a, "b:", b)
temp = a # Step 1
a = b # Step 2
b = temp # Step 3
del temp # (optional)
print("After swapping")
print("a:", a, "b:", b)
Output:
Before swapping
a: 5 b: 25
After swapping
a: 25 b: 5
Swap Two Values Using XOR Logic in Python
In Python, XOR
is represented with the ^
symbol. And, we can use it to perform swapping in the following way. Note that this approach only works for integers. For float values, we will get the following error.
TypeError: unsupported operand type(s) for ^=: 'float' and 'float'`.
a = 5
b = 25
print("Before swapping")
print("a:", a, "b:", b)
a ^= b # Step 1
b ^= a # Step 2
a ^= b # Step 3
print("After swapping")
print("a:", a, "b:", b)
Output:
Before swapping
a: 5 b: 25
After swapping
a: 25 b: 5