Subprocess.check_output in Python
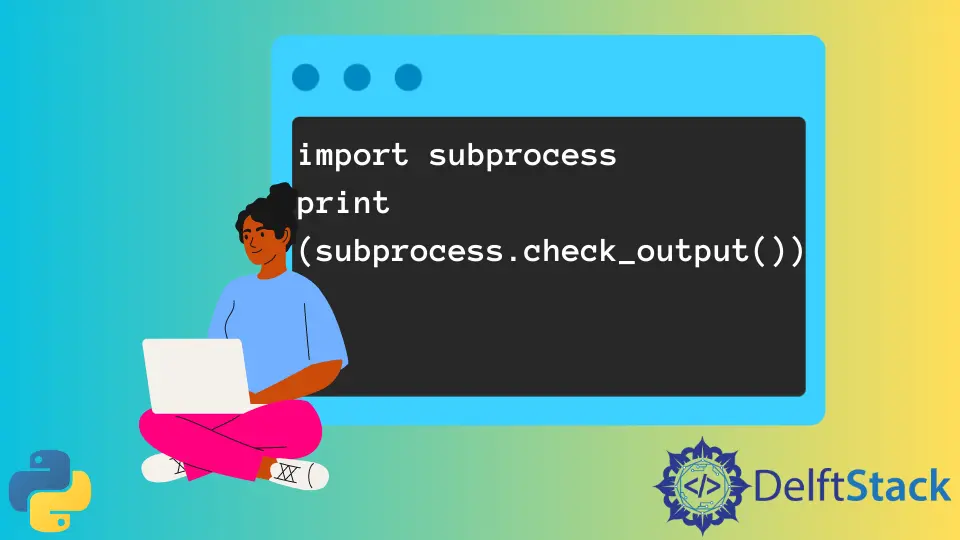
The subprocess
module is an integral part of Python and helps achieve parallel programming. This module can spawn new processes and work with programs controlled using the command-line tool.
It stores different functions that can help us run commands via a command-line tool. One such function is the subprocess.check_output()
function in Python.
This tutorial will demonstrate the subprocess.check_output()
function in Python.
Use subprocess.check_output()
in Python
This function was introduced with Python 2.7. We can use it to run a command in the command prompt with arguments in Python.
The subprocess.check_output()
function will return the output of the given command as bytes. A CalledProcessError
object is raised if the function returns a non-zero code.
A CalledProcessError
object has two attributes. These are the returncode
and output
attributes.
A simple example of this function is shown below.
import subprocess
print(subprocess.check_output(["echo", "Sample Message"], shell=False))
Output:
b'Sample Message\n'
We run the echo
command in the above example displaying a message. The output returned is a bytes string with the required message.
There are several arguments associated with this function. As seen in the command, we can provide the command with its arguments in the function.
Apart from that, we also have the stdin
, stderr
, shell
, and universal_newlines
arguments.
The first three arguments are the standard input, output, and error file handles. We can specify their values as PIPE
objects.
As discussed, the subprocess
module creates new child processes. The PIPE
constants send or receive information from the parent process.
The shell
argument can be either True
or False
. The command will run through the shell if set to True
.
However, it is not recommended to run commands through shell via Python. This can pose a serious security risk while reading input from untrusted sources that can raise security threats like shell injection.
If the shell
argument is set to True
, we can use the pipes.quotes()
function to escape whitespace and metacharacters of the shell within the passed string.
The universal_newlines
argument is set to True
, then the object provided as standard input, output, and errors are opened in text mode. In other functions, the same argument was used with an alias with the name of the text
argument.
Python 3.5 introduced a new function called subprocess.run()
. This function gained popularity over the traditional subprocess.check_output()
method to run commands; it returns a CompletedProcess
object.
The subprocess.run()
function also takes many arguments, most of which are not available in the subprocess.check_output()
method. Most of these arguments are passed via the interface.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn