SSH Using Python
-
Use the
paramiko
Library in Python to Create SSH Connections and Run Commands -
Use the
subprocess
Module to Create SSH Connections and Run Commands
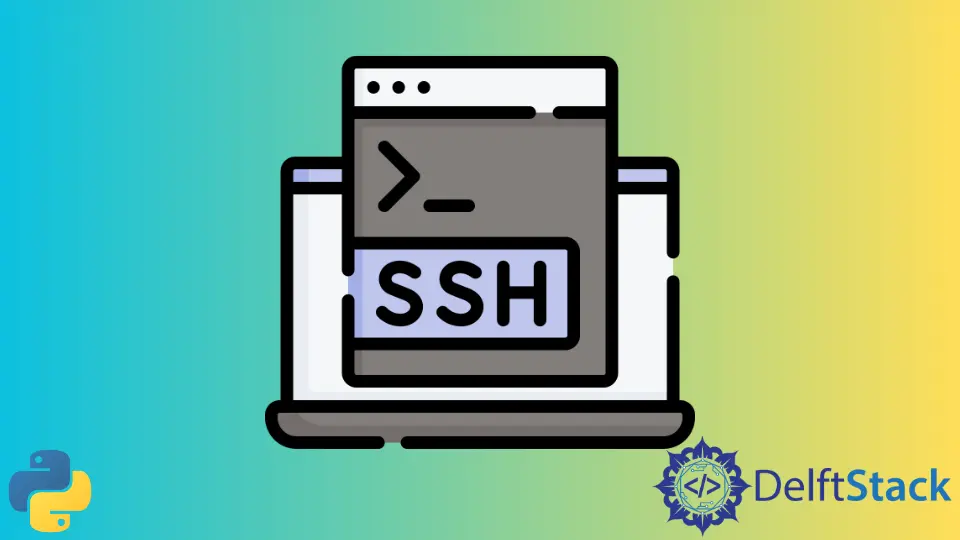
SSH (stands for Secure Socket Shell
) is a highly used network protocol for secure, encrypted communication services over an unknown and insecure network. It allows us to send commands between two devices over such a network remotely and has many applications.
Python has modules available that allow such SSH execution. This tutorial will discuss how to run shell commands to a remote device after securing the SSH connection.
Use the paramiko
Library in Python to Create SSH Connections and Run Commands
The paramiko
module is frequently used for implementing SSH protocols in Python. It allows both server and client-side communication.
Check the code below for running shell commands on a remote device using this module.
ssh = paramiko.SSHClient()
ssh.connect(server, username=username, password=password)
ssh_stdin, ssh_stdout, ssh_stderr = ssh.exec_command(cmd_to_execute)
The SSHClient()
function specifies an SSH server session. We connect to the device using the proper authentication details in the connect
function. The required commands can be executed on this device using the exec_command()
function.
Another module named spur
is derived from this module but has a slightly better interface.
Use the subprocess
Module to Create SSH Connections and Run Commands
The check_output()
function from the subprocess
module can be used to run commands passed to it, and the function returns the output of this command. We can create passwordless SSH connections with this function to execute the required commands.
The following code shows an example of this.
subprocess.check_output(["ssh", "my_server", "echo /*/"])
Another method that can be used from this module is the Popen()
. It is similar to the os.Popen()
function from the os
module to run commands and external programs. In this module, the Popen
is a class and not a method. It is a little complicated to use this due to various parameters that need to be specified along with the required command. It combines all the 4 os.popen()
functions into one. It also executes the command as a separate process. We can use it to create SSH connections and run commands, as shown below.
subprocess.Popen(
"ssh {user}@{host} {cmd}".format(user=user, host=host, cmd="ls -l"),
shell=True,
stdout=subprocess.PIPE,
stderr=subprocess.PIPE,
).communicate()
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn