How to Send Email in Python
Najwa Riyaz
Feb 02, 2024
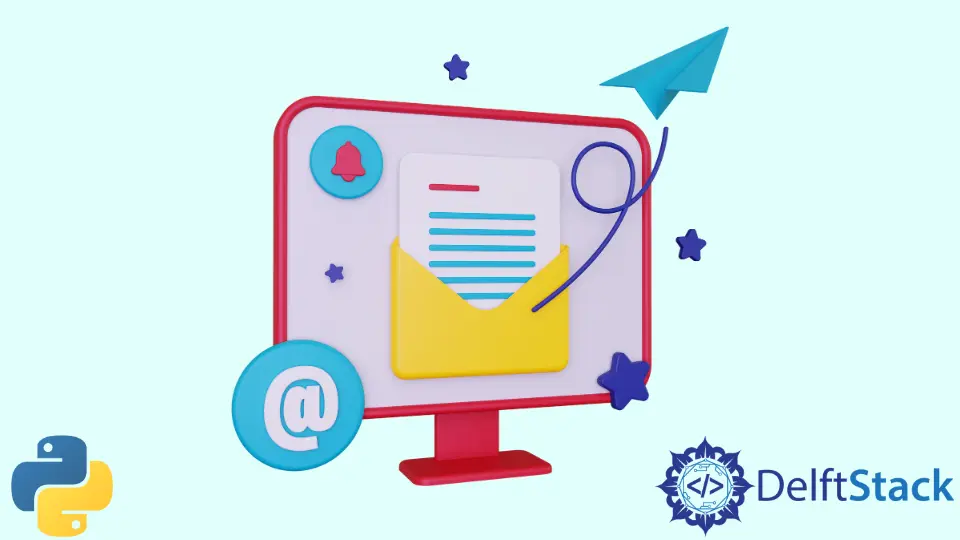
To send an email using Python, use the following functions from the smtplib
library.
SMTP(SMTP_SERVER, SMTP_PORT)
- creates an SMTP session as per the SMTP server and the SMTP port details.starttls()
- to start the TLS for the security setup.login(SMTP_USERNAME, SMTP_PASSWORD)
- for providing the email sender’s authentication details.sendmail(EMAIL_FROM, EMAIL_TO, the_message)
- to send the message from the Sender to the Receiver.quit()
to quit the SMTP Session.
Here is an example that demonstrates the sending of mail through the Gmail SMTP server.
import smtplib
SMTP_SERVER = "smtp.gmail.com"
SMTP_PORT = 587
SMTP_USERNAME = "sender_username_here@gmail.com"
SMTP_PASSWORD = "sender_password_here"
EMAIL_FROM = "sender_username_here@gmail.com"
EMAIL_TO = "receiver_username_here@gmail.com"
EMAIL_SUBJECT = "Attention:Subject here"
EMAIL_MESSAGE = "The message here"
s = smtplib.SMTP(SMTP_SERVER, SMTP_PORT)
s.starttls()
s.login(SMTP_USERNAME, SMTP_PASSWORD)
message = "Subject: {}\n\n{}".format(EMAIL_SUBJECT, EMAIL_MESSAGE)
s.sendmail(EMAIL_FROM, EMAIL_TO, message)
s.quit()
The output may look as follows as per the connection built at that point in your system -
(221, b"2.0.0 closing connection t12sm4676768pfc.133 - gsmtp")
As a result, the email is sent. The receiver receives the message successfully.
Note:
- In case of Gmail, provide the following SMTP details :
SMTP_SERVER = "smtp.gmail.com"
SMTP_PORT = 587
Also, the following setting needs to be enabled in Gmail before executing the code.
https://myaccount.google.com/lesssecureapps
- In case of Yahoo Mail, provide the following SMTP details :
SMTP_SERVER = "smtp.mail.yahoo.com"
SMTP_PORT = 587
Also, generate a Yahoo App Password
before executing the code. Check the link below.
https://help.yahoo.com/kb/generate-separate-password-sln15241.html
- Similarly, if you wish to use any other mail provider, use its respective SMTP server details and pre-requisites.