Python Selenium Headless: Open Chrome Browser in the Headless Mode
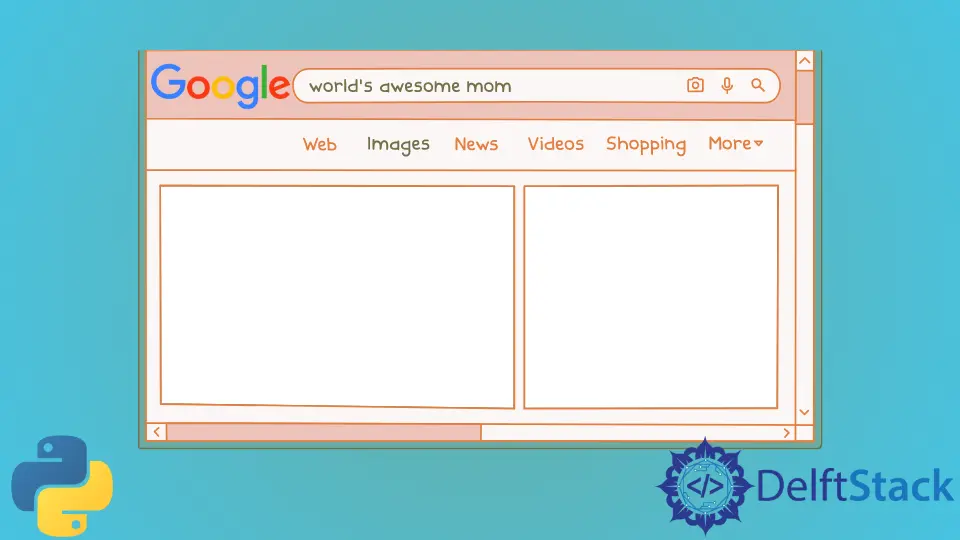
This tutorial explains how we can run our browser headless mode with Selenium in Python.
Use Selenium in Python to Run Chrome Browser in Headless Mode
To talk about the headless browser, you can also call them a real browser, but they are running in the background; you will not be able to see them anywhere but still running in the background.
There will be a couple of scenarios where you need this headless browser.
Because when you work in the normal browser, you will see the UI coming up and operating other applications while working on a local system. So, you will not be able to perform any other operation causing the additional operation to run in front of you.
Suppose you run your script in headless mode. So that you can continue with your work, there are several browsers available to use a headless mode like phantomJS
, HtmlUnit
, and much more, see here.
We also have the headless option with Chrome and Firefox. To see how you can run the test in headless mode in Selenium with Chrome, we first need to create a Python file.
We will jump into the code by importing some required classes and modules.
import time
from selenium.webdriver.common.by import By
from webdriver_manager.chrome import ChromeDriverManager
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
We just noticed that webdriver.Chrom()
has different options, as you can see below.
We used a service
argument that should start the Chrome session. The ChromeDriverManager()
will help us to download the driver and set the path
.
We will use the get()
method and pass it a URL
where we will try to find the search box, and then we will use some random text that we want to search by using find_element()
.
DV = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
DV.get("http://www.google.com")
DV.find_element(By.NAME, "q").send_keys("Elon Musk")
time.sleep(2)
If we run the python script, you will notice that it will not run in the headless mode and go to the search box and search the given query.
Now we will use the second argument called options
, and we need to provide options
. We have an options
class from different packages; you can use it for Opera, Chrome, and Firefox.
Since we are using Chrome, we will use the Options()
class from Chrome.options
.So we will create an object named OP
to call the Options()
class.
There are two options
or different ways to run your test in headless mode. First, you must use the add_argument()
method and pass --headless
inside it.
To make this effect, we must pass OP
to the options
parameter.
import time
from selenium.webdriver.common.by import By
from webdriver_manager.chrome import ChromeDriverManager
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
OP = Options()
OP.add_argument("--headless")
DV = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=OP)
DV.get("http://www.google.com")
DV.find_element(By.NAME, "q").send_keys("Elon Musk")
When you are done, you will see the test will run in the headless mode, and you’ll not be able to see anything.
To check whether it is searched or not, we will capture the screenshot in the headless mode using the get_screenshot_as_file()
method. It will open a browser in headless mode and immediately grab the screenshot.
DV.get_screenshot_as_file(os.getcwd() + "/screenshot.png")
Now we got a screenshot t of what is happening in the background.
Suppose you are someone who generally makes spelling mistakes, and sometimes it is hard to remember OP.add_argument('--headless')
. Then, we also have one more option: type property called headless
.
By default, it is set to False
, and you can change it to True
, so you do not need to use add_argument()
.
import time
from selenium.webdriver.common.by import By
from webdriver_manager.chrome import ChromeDriverManager
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
import os
OP = Options()
OP.headless = True
# OP.add_argument('--headless')
DV = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=OP)
DV.get("http://www.google.com")
# DV.find_element(By.NAME,'q').send_keys('Elon Musk')
DV.find_element(By.NAME, "q").send_keys("mark zuckerberg")
DV.get_screenshot_as_file(os.getcwd() + "/screenshot.png")
After rerunning this script, we have got a screenshot of what operation is performing in the background.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn