How to Round Up to the Nearest Ten in Python
-
Use the
ceil()
Function to Round Up to the Nearest Ten in Python -
Use the
floor()
Function to Round Up to the Nearest Ten in Python -
Use the
round()
Function to Round Up to the Nearest Ten in Python - Conclusion
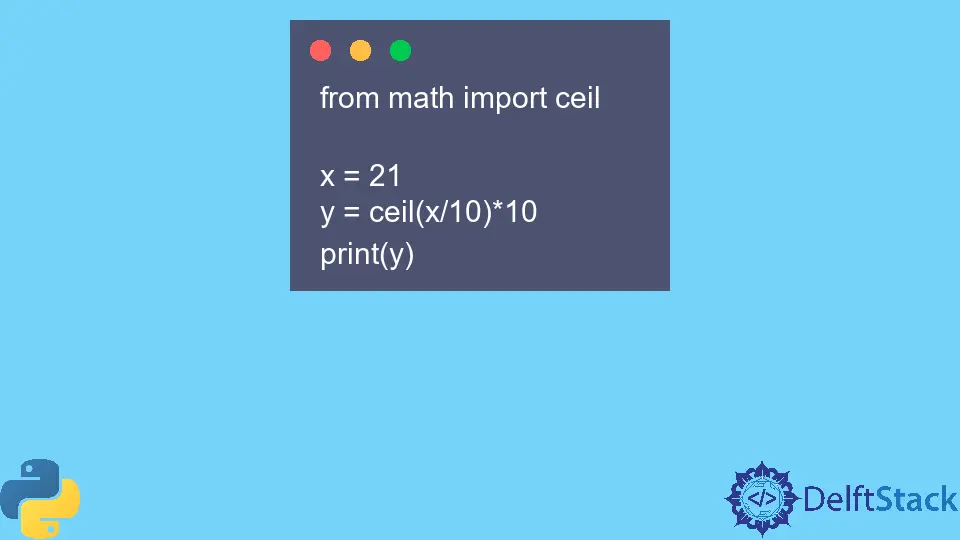
In this tutorial, we delve into Python’s various methods for rounding numbers to the nearest ten. From utilizing the ceil()
and floor()
functions to introducing the versatile round()
function, this guide equips us with efficient techniques for handling rounding scenarios in our code.
Use the ceil()
Function to Round Up to the Nearest Ten in Python
The ceil()
function belongs to the math
module, and it is used to round a floating point number to the nearest integer greater than or equal to the given number.
Basic Syntax:
import math
result = math.ceil(x)
In the syntax, the x
is the number you want to round up. The ceil()
function returns the smallest integer equal ro greater than x
, and it rounds up the number to the next integer.
Let’s have a basic example of using the ceil()
function.
Example Code:
from math import ceil
n = 2.1
print(ceil(n))
Output:
3
In the code above, we import the ceil()
function from the math
module and use it to round the floating-point number 2.1
up to the nearest integer. The output is 3
because the ceil()
function rounds the floating-point number 2.1
up to the nearest integer greater than or equal to it.
Now, if we want to round an integer to the nearest ten, we have to convert the number to a floating point first by dividing it by 10, and then we can pass it to the ceil()
function, and we can multiply the result with 10 to get the required number.
Let’s look at the following example for better understanding.
Example Code:
from math import ceil
x = 21
y = ceil(x / 10) * 10
print(y)
Output:
30
In the code, we first initialize a variable x = 21
. Next, we use the ceil()
function from the math
module to round the result of x / 10
to the nearest integer greater than or equal to it.
Specifically, we divide 21
by 10
, resulting in 2.1
, and apply ceil()
to round it up to 3
. Subsequently, we multiply this result by 10
, yielding the final value of 30
.
Finally, we print the value of y
which is 30
, representing the result of rounding 21
to the nearest ten using the ceil()
function.
Use the floor()
Function to Round Up to the Nearest Ten in Python
The floor()
function of the math
module is used to round the given floating point number to the nearest integer that is less than or equal to the given number.
Basic Syntax:
import math
result = math.floor(x)
In the syntax, the x
is the number you want to round down. The function floor()
returns the largest integer equal to or less than x
, and it rounds down the number to the previous integer.
For example, if we use the floor()
function in the above code instead of the ceil()
function, the result will be 20
.
from math import floor
x = 21
y = floor(x / 10) * 10
print(y)
Output:
20
In the code, we employ the floor()
function from the math
module to round down the result of x / 10
to the nearest integer less than or equal to it. Specifically, dividing 21
by 10
yields 2.1
, and the floor()
function ensures this value is rounded down to 2
.
Then, multiplying this result by 10
produces the final value of 20
. Finally, we use the print()
function to display the value of y
.
Consequently, the output of the code is 20
, representing the result of rounding 21
down to the nearest ten using the floor()
function.
Use the round()
Function to Round Up to the Nearest Ten in Python
The round()
function is a Python built-in function that is used for rounding a number to a specified number of digits after the decimal point or to the nearest integer if no precision is specified. The round()
is a mixture of the floor()
and ceil()
functions, and it rounds a floating point number to the nearest integer, which can be less than, greater than, or equal to the input number.
The function will start from the last significant digit of a floating point number; if it is greater than 5, the current significant digit will be dropped, and the digit before it will be increased by one number. But if the significant digit is less than or equal to 5, the significant digit before it will remain the same.
Basic Syntax:
rounded_number = round(number, ndigits)
In this syntax, we use the round()
function to round a numeric value specified by the variable number
. The optional parameter ndigits
determines the precision after the decimal point; if omitted, the function rounds to the nearest integer, and the result is stored in the variable rounded_number
.
Let’s show an example where the significant digit is less than 5.
Example Code:
x = 21
y3 = round(x / 10) * 10
print(y3)
Output:
20
In the code above, we initialize a variable x = 21
. We then employ the round()
function to round the result of x / 10
to the nearest integer.
Specifically, the division yields 2.1
, and rounding this value results in 2
. Multiplying this rounded result by 10
produces the final value of 20
.
Consequently, we use the print()
function to display the value of y3
. Therefore, the output of the code is 20,
representing the result of rounding 21
down to the nearest ten using the round()
function.
Now, let’s have another example where the significant digit is greater than 5.
Example Code:
x = 26
y3 = round(x / 10) * 10
print(y3)
Output:
30
In the code above, we round the result of x / 10
to the nearest integer.
In this case, the division yields 2.6
, and rounding it results in 3
. Multiplying this rounded result by 10
produces the final value of 30
.
Consequently, we use the print()
function to display the value of y3
. Therefore, the output of the code is 30
, indicating the result of rounding 26
up to the nearest ten using the round()
function.
We can see in both code examples above that the round()
function will act as the ceil()
function if the significant digit is greater than 5. If the digit is less than or equal to 5, it will act as the floor()
function.
Conclusion
In this tutorial, we explored different Python methods for rounding numbers to the nearest ten. Beginning with the ceil()
function, we rounded up floating-point numbers.
We then showcased the floor()
function for rounding down. Lastly, we introduced the versatile round()
function, illustrating its behavior with examples.
By examining these methods, we aimed to equip Python developers with efficient techniques for rounding numbers in their code.