How to Print Stack Trace in Python
- Using the traceback Module
- Using the sys Module
- Customizing Stack Trace Output
- Logging Stack Traces
- Conclusion
- FAQ
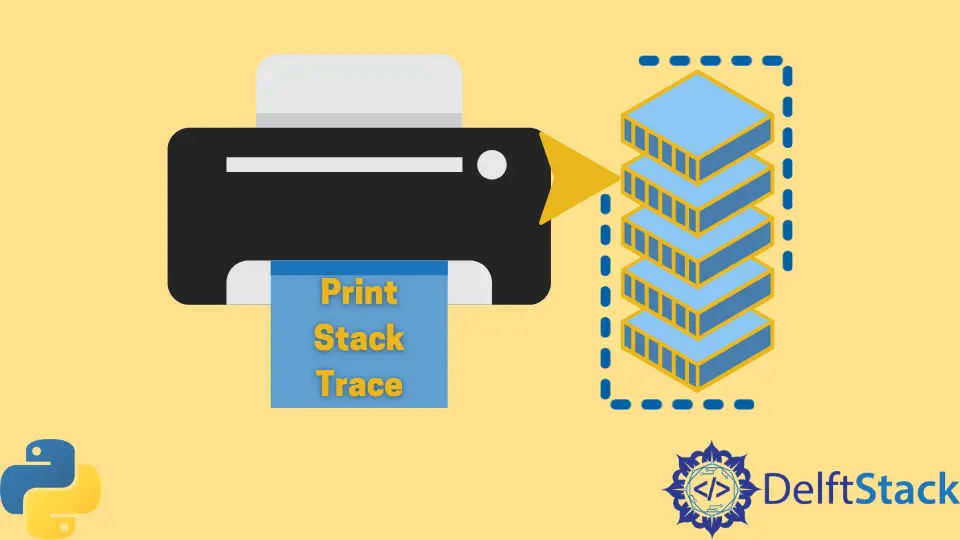
When programming in Python, encountering errors is a common experience. Understanding how to print a stack trace can be incredibly helpful for debugging. A stack trace provides a snapshot of the call stack at a specific point in time, usually when an exception occurs.
This tutorial will guide you through various methods to print stack traces in Python, empowering you to diagnose issues in your code quickly. Whether you are a beginner or an experienced developer, mastering stack traces will enhance your debugging skills and improve your coding efficiency. Let’s dive into the methods and see how you can effectively print stack traces in Python.
Using the traceback Module
The traceback
module is a built-in Python library that provides utilities for extracting, formatting, and printing stack traces. It’s an essential tool for any developer looking to gain insights into errors. Here’s how you can use it:
import traceback
def faulty_function():
return 1 / 0
try:
faulty_function()
except Exception:
traceback.print_exc()
Output:
Traceback (most recent call last):
File "script.py", line 8, in <module>
faulty_function()
File "script.py", line 5, in faulty_function
return 1 / 0
ZeroDivisionError: division by zero
In this example, we define a function called faulty_function
that deliberately raises a ZeroDivisionError
. We wrap the function call in a try
block and catch any exceptions that occur. When an exception is caught, traceback.print_exc()
is called, which prints the stack trace to the console. This stack trace shows the sequence of calls that led to the error, making it easier to pinpoint the problem.
Using the sys Module
Another way to print a stack trace is by using the sys
module, which provides access to system-specific parameters and functions. The sys.exc_info()
function can be used to retrieve information about the most recent exception. Here’s how it works:
import sys
import traceback
def another_faulty_function():
return [][1]
try:
another_faulty_function()
except Exception:
exc_type, exc_value, exc_traceback = sys.exc_info()
traceback.print_exception(exc_type, exc_value, exc_traceback)
Output:
Traceback (most recent call last):
File "script.py", line 8, in <module>
another_faulty_function()
File "script.py", line 5, in another_faulty_function
return [][1]
IndexError: list index out of range
In this example, another_faulty_function
raises an IndexError
by trying to access an index in an empty list. The sys.exc_info()
function captures the exception type, value, and traceback, which are then passed to traceback.print_exception()
. This method provides more control over how the stack trace is printed and can be useful for logging or displaying errors in a user-friendly manner.
Customizing Stack Trace Output
You can also customize the output of your stack trace by using the traceback
module’s formatting functions. This approach allows you to tailor the information displayed according to your needs. Here’s an example:
import traceback
def custom_faulty_function():
return 'a' + 1
try:
custom_faulty_function()
except Exception as e:
formatted_traceback = traceback.format_exc()
print("Custom Error Message:")
print(formatted_traceback)
Output:
Custom Error Message:
Traceback (most recent call last):
File "script.py", line 8, in <module>
custom_faulty_function()
File "script.py", line 5, in custom_faulty_function
return 'a' + 1
TypeError: can only concatenate str (not "int") to str
In this case, we define a custom_faulty_function
that raises a TypeError
. Instead of printing the stack trace directly, we use traceback.format_exc()
to get a formatted string of the stack trace. This allows us to prepend a custom error message before displaying the traceback, making it clearer for the end-user or developer reviewing the logs.
Logging Stack Traces
For production applications, it’s often best to log stack traces instead of printing them to the console. The logging
module in Python provides a robust way to log errors, including stack traces. Here’s how you can implement logging for stack traces:
import logging
import traceback
logging.basicConfig(level=logging.ERROR, filename='app.log')
def log_faulty_function():
return 1 / 0
try:
log_faulty_function()
except Exception:
logging.error("An error occurred", exc_info=True)
Output:
An error occurred
Traceback (most recent call last):
File "script.py", line 8, in <module>
log_faulty_function()
File "script.py", line 5, in log_faulty_function
return 1 / 0
ZeroDivisionError: division by zero
In this example, we configure the logging system to log error messages to a file called app.log
. When an exception occurs, we call logging.error()
with the message and set exc_info=True
to include the stack trace in the log. This method is particularly useful for long-running applications where you want to keep a record of errors without cluttering the console output.
Conclusion
Printing stack traces in Python is an essential skill for debugging and error handling. By utilizing the built-in traceback
and sys
modules, along with the logging
module for production environments, you can efficiently diagnose issues in your code. Each method discussed offers unique advantages, allowing you to choose the best approach based on your specific needs. By mastering these techniques, you can enhance your debugging capabilities and write more robust Python applications.
FAQ
-
What is a stack trace in Python?
A stack trace is a report of the active stack frames at a certain point in time, usually when an exception occurs, and it helps identify the source of the error. -
How do I print a stack trace in Python?
You can print a stack trace using thetraceback
module, typically with thetraceback.print_exc()
function within an exception handling block. -
Can I customize the stack trace output in Python?
Yes, you can customize the output usingtraceback.format_exc()
to format the stack trace as a string and prepend custom messages. -
Is it better to print stack traces or log them?
In production environments, it’s generally better to log stack traces to a file for later analysis rather than printing them to the console. -
What are some common exceptions I might encounter in Python?
Common exceptions includeValueError
,TypeError
,IndexError
, andZeroDivisionError
, among others.