How to Print a Line Break in Python
- Use the Newline Character (`
-
Use a
for
Loop to Print a String With Line Breaks in Python - Use Triple-Quoted Strings to Print a Multiline Text With Line Breaks in Python
- Conclusion
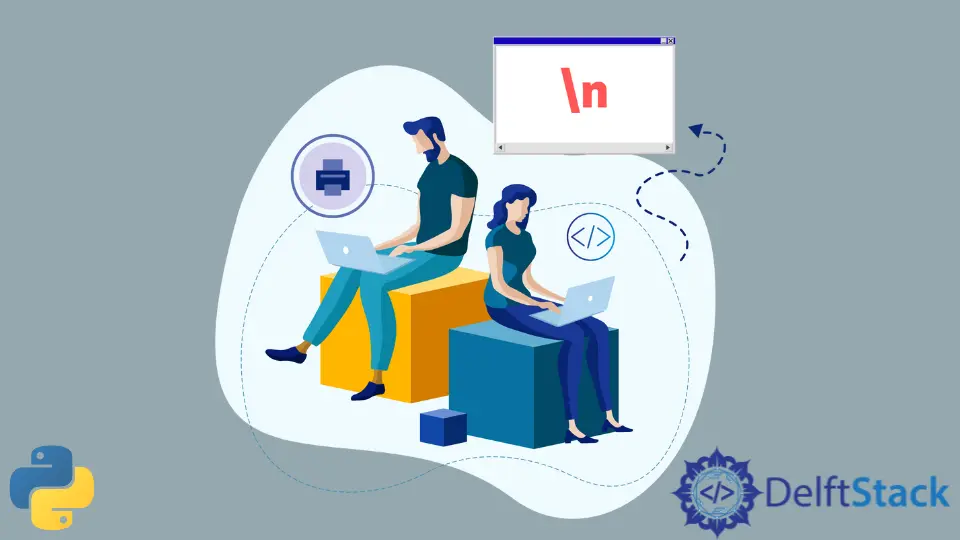
In a programming language, the next line is denoted by a newline character (\n
). This character indicates that whatever text is present after it will be printed in the next line, and strings are filled with these newline characters to represent the next lines.
In Python, we can use this character to print text in the next lines or create line breaks. We’ll also discuss the other ways to print a string, text or elements with line breaks.
Use the Newline Character (`
`) to Print a String With Line Breaks in Python
The newline character (\n
) in Python is used to create line breaks when printing strings. In the following code, we’ll see that each part of the string separated by \n
appears on a new line, creating a visually separated and well-formatted output.
Code 1:
print("Welcome\nto\nDelftstack")
Output:
Welcome
to
Delftstack
In the code above, we use the print
function to display the string "Welcome\nto\nDelftstack"
. The \n
characters within the string serve as newline indicators, prompting a line break at each occurrence.
The output presents the text on separate lines.
We can also use Python’s print
function, the sep
parameter, to specify the separator between the values that are passed to the print
function. By default, the sep
parameter is set to a space character (' '
).
However, you can customize it to use a different separator.
Code 2:
print("Welcome", "To", "Delftstack", sep="\n")
Output:
Welcome
To
Delftstack
In this code, we use the print
function with the sep
parameter set to \n
(sep="\n"
), enabling us to output the strings on individual lines. By customizing the separator to the newline character (\n
), we achieve a neatly formatted output with each string appearing on its own line.
Use a for
Loop to Print a String With Line Breaks in Python
We can utilize the for
loop to print each character of a string with a line break. The for
loop iterates through each character within the string variable, and the print(char)
statement prints each character on a new line.
Note that the comma and exclamation marks are also printed on separate lines due to the loop iterating over each character.
Code 1:
my_string = "Delftstack"
for char in my_string:
print(char)
Output:
D
e
l
f
t
s
t
a
c
k
In the code above, we initialize a string variable, my_string = "Delftstack"
. Subsequently, we employ a for
loop to iterate through each character in the string.
Within the loop, we use the print
function to output each character on a new line. We can see in the output that the characters of the string "Delftstack"
are printed sequentially, with each character appearing on its own line.
In this next example, we use the for
loop to iterate through the elements of the list a
and print each element followed by a newline character. The end="\n"
argument in the print
function sets the end parameter to "\n"
, which means that each print statement will end with a newline character.
Code 2:
a = [1, 2, 3, 4, 5]
for x in a:
print(x, end="\n")
Output:
1
2
3
4
5
In this code, we initialize a list, a = [1, 2, 3, 4, 5]
. Next, using a for
loop, we iterate through each element in the list, and within the loop, we use the print
function to display each element, denoted as x
, on a new line.
The end="\n"
parameter is set explicitly to ensure that each print statement concludes with a newline character, enhancing the clarity of the output. In the output, the numbers 1 through 5 are printed sequentially, each on its own line.
Use Triple-Quoted Strings to Print a Multiline Text With Line Breaks in Python
In Python, triple-quoted strings (either double-quoted """
or single-quoted '''
) allow you to define multiline strings. The primary advantage is that line breaks and formatting within the string are preserved when the string is printed or used in code.
Code:
# Double-quoted string
print(
"""
Welcome
To
Delftstack
"""
)
# Single-quoted string
print(
"""
Lets
learn
Programming
"""
)