How to Validate Phone Number in Python
- Validate Phone Numbers in Python
-
Use the
Phonenumbers
Library in Python -
Parse Phone Numbers With Python
Phonenumbers
Library -
Validate Phone Numbers Using the
is_possible_number()
Method -
Validate Phone Numbers Using the
is_valid_number()
Method -
Use the
description_for_number()
Method in theGeocoder
Class to Find the Phone Number Location -
Use the
name_for_number()
Method in theCarrier
Class to Find the Phone Number Carrier - Validate Phone Numbers and Get Additional Information Using Python
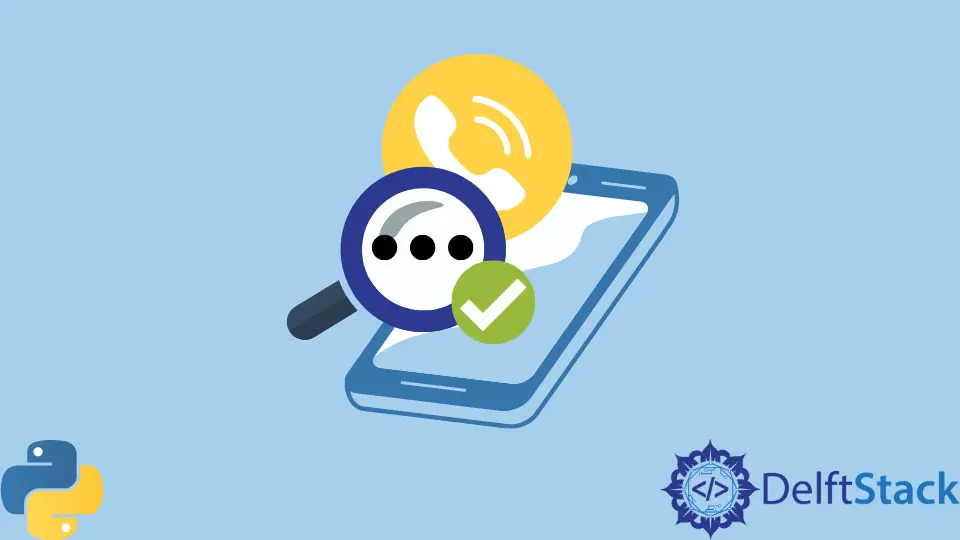
This Python article will show how we can validate phone numbers using Python’s phonenumbers
library. We will learn to use Python to validate phone numbers and then format them for display according to region, country, continent, and carrier.
We will start with a simple example and move to a complex one. So let’s get started and let Python validate and format phone numbers.
Validate Phone Numbers in Python
Verifying and validating the correctness of phone numbers can be very challenging. A phone number’s format can change depending on our country.
Even within the same nation, there may be significant differences. Some countries use the same country code, while others use multiple country codes.
Some countries even use the same country code more than once.
An illustration in Google’s libphonenumber
repository on GitHub demonstrates that the United States of America, Canada, and the Caribbean islands all use the same international dialing code of +1.
The phone numbers can be called from Kosovo using the country codes for Serbia, Slovenia, or Morocco. These are just a few difficulties when identifying or validating phone numbers.
At first glance, it appears that one can use RegEx
to validate the country code of a phone number. However, this indicates that to validate a country’s code, we must create a unique RegEx
rule for every country worldwide.
In addition, mobile phone carriers each have guidelines (for example, certain digits can only be used in a specific range of numbers). We can see that things have the potential to quickly spiral out of control, which would make it extremely difficult for us to validate phone number inputs on our own.
Use the Phonenumbers
Library in Python
We are lucky because a Python library can effectively guide us through the validation process. This library was derived from Google’s libphonenumber
library, which can also be used for other programming languages such as C++, Java, and JavaScript.
The Python Phonenumbers
library was created using this library.
This article will show us how to use Python to validate a mobile number.
Install the Phonenumbers
Library in Python
Validating phone numbers is made easy with Python thanks to an integrated module. This module is referred to as the phonenumbers
module.
To make use of the module, we must first install it. Using pip
, we can do this:
pip install phonenumbers
Once the module is installed, we can validate country code phone numbers.
Now that everything has been set up, we can explore the Phonenumbers
library.
Parse Phone Numbers With Python Phonenumbers
Library
The phone number will almost certainly be a string, regardless of whether the input comes from a web form or some other source, such as text extraction (which will be covered later in this guide). It needs to be parsed with phone numbers, then converted into a PhoneNumber
instance before we can use it for validation and other purposes.
Import the Library
First, we need to import the required libraries; we will import phonenumbers
. We will also need to import the carrier
, timezone
, and geocoder
.
Example Code:
import phonenumbers
Parse the Phone Number
We can extract useful information from the phone number thanks to the parse()
method, which includes the following:
Example Code:
import phonenumbers
My_number = "+92333920000"
Parse_number = phonenumbers.parse(My_number)
A phone number string is needed as an input when using the phonenumbers.parse()
function. We can provide the national information in ISO Alpha-2 format as additional optional input.
Take into mind, for instance, the following piece of code:
Parse_number = phonenumbers.parse(My_number)
In ISO Alpha-2 notation, RO
denotes Romania. We may use this page to look up the Alpha-2 and numeric country codes for every nation worldwide.
Unless essential, the ISO Alpha-2 country code will be omitted from most instances.
Phone numbers can be validated for common issues like length, leading zeros, and signs using phonenumbers.parse()
. It’s important to remember that if any of the required conditions are not satisfied, this method will throw an exception.
Always put it in a try/catch
block in our code.
Now that our phone number has been appropriately processed, we can proceed to the validation phase.
Validate Phone Numbers Using the is_possible_number()
Method
Two approaches can be used to validate a phone number on Phonenumbers
. The primary distinction between these approaches is their respective speed and accuracy levels.
To explain this further, let’s begin with the is_possible_number()
function.
Example Code:
import phonenumbers
My_number = "+92333920000"
Parse_number = phonenumbers.parse(My_number)
print(phonenumbers.is_possible_number(Parse_number))
Output:
True
The program will produce a value of True
as its output.
Validate Phone Numbers Using the is_valid_number()
Method
Now, we will use the same number, but this time we’ll check its validity using the is_valid_number()
method:
Example Code:
import phonenumbers
My_number = "+92333920000"
Parse_number = phonenumbers.parse(My_number)
print(phonenumbers.is_valid_number(Parse_number))
Output:
False
The output would be different even though the input remained the same.
is_possible_number()
guesses the phone number’s validity by checking its length, while the is_valid_number()
method checks its length, prefix, and region.
phonenumbers.is_possible_number()
is faster than phonenumbers.is_valid_number()
in iterating over a large phone numbers list. These results aren’t always accurate, as shown.
It’s helpful to eliminate long phone numbers quickly. It’s risky to use.
Use the description_for_number()
Method in the Geocoder
Class to Find the Phone Number Location
A phone number can reveal many details about its owner, some of which may be relevant to our research. Because the particular carrier associated with a phone number is a factor in the overall cost of the product, we might want to use various application programming interfaces (APIs) or API endpoints.
We might like to send our promotion notifications based on the timezone of our customers (phone numbers) location so that we can avoid sending them a message in the middle of the night. It will prevent us from waking them up accidentally.
We could also get information about the phone number’s location to provide information pertinent to the situation. The Phonenumbers
library gives users access to the tools required to satisfy these requirements.
We will use the description_for_number()
method available in the geocoder
class to start with the location. The parameters are a phone number that has been parsed and a short name for the language.
Let’s try this with the fake number we were using before.
Example Code:
import phonenumbers
from phonenumbers import geocoder
My_number = phonenumbers.parse("+92333920000")
print("This number is from: " + geocoder.description_for_number(My_number, "en"))
Output:
This number is from: Pakistan
Use the name_for_number()
Method in the Carrier
Class to Find the Phone Number Carrier
We will use the method name_for_number()
found in the carrier
class.
Example Code:
import phonenumbers
from phonenumbers import carrier
My_number = phonenumbers.parse("+92333920000")
print("The carrier of the number is: " + carrier.name_for_number(My_number, "en"))
The output of the code:
The carrier of the number is: Ufone
Validate Phone Numbers and Get Additional Information Using Python
Example Code:
import phonenumbers
from phonenumbers import carrier, timezone, geocoder
my_number = phonenumbers.parse("+92333920000", "GB")
print((phonenumbers.is_valid_number(my_number)))
print("The carrier of the number is: " + carrier.name_for_number(my_number, "en"))
print("The content and the city of the number are:")
print(timezone.time_zones_for_number(my_number))
print(
"The country this number belongs to is: "
+ geocoder.description_for_number(my_number, "en")
)
Output:
True
The carrier of the number is: Ufone
The content and the city of the number are:
('Asia/Karachi',)
The country this number belongs to is: Pakistan
This is how we can determine whether a number is valid. We see True
in the output, which shows that the number used in the Python code is correct.
We can also find out the carrier of the number. In our case, it is Ufone
.
Along with the carrier, we can find the name of the country, continent, and city. According to the number we have used, the number is from Asia, Pakistan, and the city of Karachi.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn