How to Calculate Partial Derivatives in Python Using Sympy
- What is Sympy?
- Calculating Partial Derivatives with Sympy
- Evaluating Partial Derivatives at Specific Points
- Higher-Order Partial Derivatives
- Conclusion
- FAQ
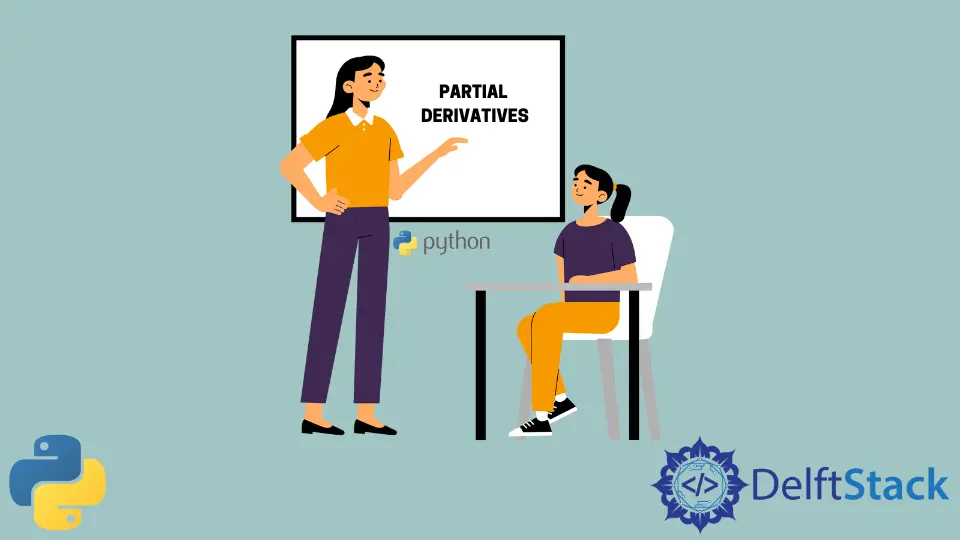
Partial derivatives are fundamental in calculus, particularly in multivariable functions. They help us understand how a function changes as we vary one variable while keeping others constant. For those venturing into the realm of data science, machine learning, or physics, mastering partial derivatives is essential.
In this article, we will explore how to use the Sympy module in Python to compute partial derivatives of functions. Sympy is a powerful library for symbolic mathematics, allowing us to perform algebraic operations, calculus, and much more. So, let’s dive into the world of Sympy and discover how to calculate partial derivatives efficiently!
What is Sympy?
Sympy is a Python library designed for symbolic mathematics. Unlike numerical libraries that provide approximate solutions, Sympy allows users to work with mathematical expressions symbolically. This means you can manipulate equations and perform calculus operations without losing precision. Whether you are solving equations, computing limits, or deriving functions, Sympy provides a comprehensive toolkit.
To get started, you need to install Sympy if you haven’t already. You can easily install it using pip:
pip install sympy
Once installed, you can import it into your Python environment and begin working with mathematical expressions.
Calculating Partial Derivatives with Sympy
To calculate partial derivatives using Sympy, you need to define your variables and the function you want to differentiate. Let’s go through the steps of computing partial derivatives for a simple function.
Example of a Partial Derivative
Suppose we have a function f(x, y) = x^2 * y + y^3. We want to compute the partial derivative of this function with respect to x and y.
Here’s how you can do it in Python:
import sympy as sp
x, y = sp.symbols('x y')
f = x**2 * y + y**3
partial_derivative_x = sp.diff(f, x)
partial_derivative_y = sp.diff(f, y)
partial_derivative_x, partial_derivative_y
Output:
(2*x*y, x**2 + 3*y**2)
In this code, we first import the Sympy library and define our symbols, x and y. We then define the function f as x^2 * y + y^3. Using the sp.diff()
function, we compute the partial derivatives with respect to x and y. The results show that the partial derivative with respect to x is 2xy, while the partial derivative with respect to y is x^2 + 3y^2.
This process highlights the simplicity and power of Sympy for calculating partial derivatives, making it an invaluable tool for anyone working with multivariable functions.
Evaluating Partial Derivatives at Specific Points
In many cases, you may want to evaluate the partial derivatives at specific points. Sympy makes this easy with its subs()
function, allowing you to substitute values for your variables.
Let’s evaluate the partial derivatives we calculated earlier at the point (x=1, y=2).
partial_derivative_x_at_point = partial_derivative_x.subs({x: 1, y: 2})
partial_derivative_y_at_point = partial_derivative_y.subs({x: 1, y: 2})
partial_derivative_x_at_point, partial_derivative_y_at_point
Output:
(4, 7)
In this code snippet, we use the subs()
method to substitute the values of x and y into our partial derivatives. The results indicate that at the point (1, 2), the partial derivative with respect to x is 4, while the partial derivative with respect to y is 7. This evaluation is crucial for applications in optimization and sensitivity analysis, where knowing the behavior of functions at specific points can lead to better decision-making.
Higher-Order Partial Derivatives
Sometimes, you might need to compute higher-order partial derivatives, which are derivatives of the derivatives. Sympy allows you to do this seamlessly. Let’s compute the second-order partial derivatives of our function f with respect to x and y.
Here’s how you can achieve this:
second_partial_derivative_xx = sp.diff(partial_derivative_x, x)
second_partial_derivative_yy = sp.diff(partial_derivative_y, y)
second_partial_derivative_xx, second_partial_derivative_yy
Output:
(2*y, 6*y)
In this example, we first compute the second partial derivative with respect to x, which results in 2y. Then, we compute the second partial derivative with respect to y, yielding 6y. Higher-order derivatives provide deeper insights into the curvature and behavior of functions, especially in optimization problems.
Conclusion
Calculating partial derivatives in Python using Sympy is a straightforward and efficient process. Whether you are working with basic functions or delving into higher-order derivatives, Sympy provides the tools necessary for symbolic computation. By mastering these techniques, you can enhance your analytical skills and apply them to various fields, including data science, engineering, and physics. As you continue to explore the capabilities of Sympy, you’ll find that it opens up a world of possibilities for mathematical modeling and problem-solving.
FAQ
-
What is a partial derivative?
A partial derivative is the derivative of a function with respect to one variable while keeping other variables constant. -
Why use Sympy for partial derivatives?
Sympy allows for symbolic computation, providing exact results rather than numerical approximations, which is essential for precise mathematical analysis. -
Can I calculate partial derivatives of functions with more than two variables?
Yes, Sympy can handle functions with multiple variables, allowing you to compute partial derivatives for any number of variables. -
Is Sympy suitable for large-scale computations?
While Sympy is excellent for symbolic mathematics, it may not be the best choice for large-scale numerical computations. For those tasks, consider libraries like NumPy or SciPy. -
How do I install Sympy?
You can install Sympy using pip with the commandpip install sympy
.