How to Open Files in Different Directory in Python
-
Use the
\
Character to Open Files in Other Directories in Python - Use the Raw Strings to Open Files in Other Directories in Python
-
Use the
pathlib.Path()
Function to Open Files in Other Directories in Python
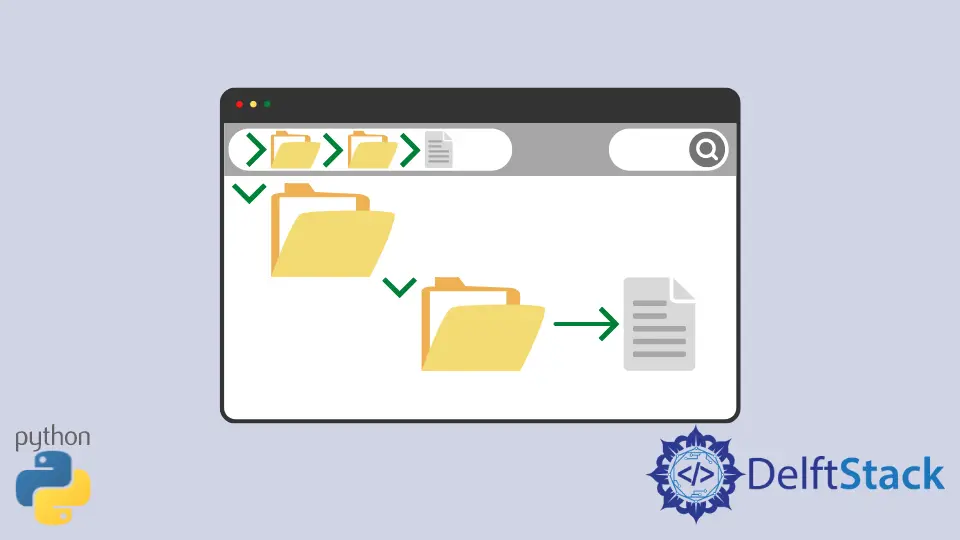
Python scripts are stored in a directory and can easily open files without specifying the full path. But, we may be required to open files in different directories also.
The open()
function is generally used to open files, and the path of such files is specified within the function.
We can specify the path normally in the function opening the file, like open('C:\Dir\Filename')
. But Python may interpret the \
as an escape character.
That is why we have other ways to specify the directory and filename in Python.
Use the \
Character to Open Files in Other Directories in Python
It is advisable to use \\
in place of \
. It removes the error of interpreting \
as an escape character.
For example,
f = open("C:\\Users\\Directory\\sample.txt")
Use the Raw Strings to Open Files in Other Directories in Python
By specifying r
before the path, we tell Python to interpret it as a raw string. This way, it will not consider \
as an escape character.
See the code below.
import os
f = open(r"C:\Users\Directory\sample.txt")
Use the pathlib.Path()
Function to Open Files in Other Directories in Python
The pathlib
module helps with the path-related tasks that include constructing new paths from the file names and checking different properties of paths.
We can use this module to create paths for different files and use it in the open()
function.
For example,
from pathlib import Path
file_path = Path(r"C:\Users\Directory\sample.txt")
f = open(file_path)
This method is available in Python 3.4 and above.