Python MRO (Method Resolution Order)
- What is Python MRO?
- How to Access MRO in Python
- The Significance of MRO in Multiple Inheritance
-
Customizing MRO with
super()
- Conclusion
- FAQ
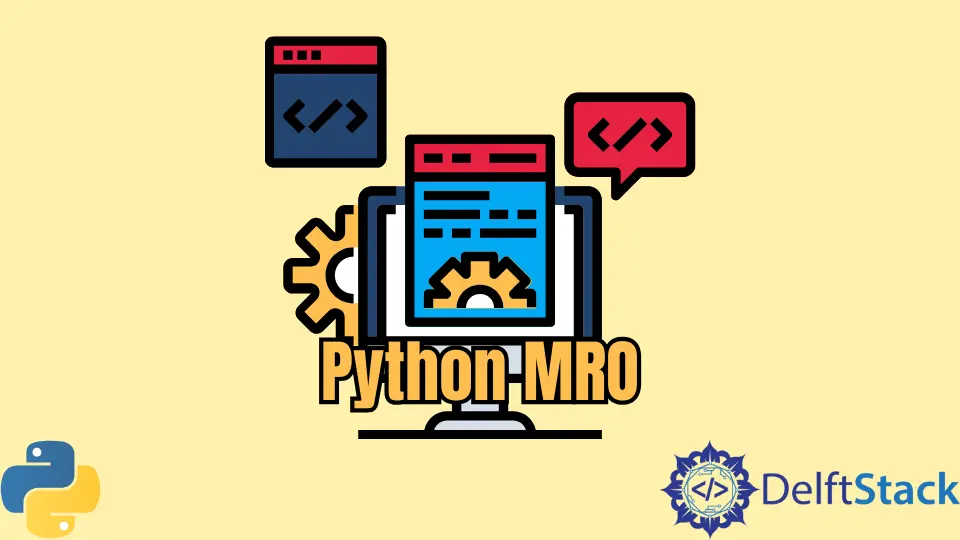
In the world of object-oriented programming, inheritance plays a crucial role, allowing us to create new classes based on existing ones. However, with great power comes great complexity, especially when it comes to multiple inheritance. This is where Python’s Method Resolution Order (MRO) comes into play. MRO defines the order in which Python looks for a method in a hierarchy of classes. Understanding MRO is essential for anyone who wants to master Python’s object-oriented features.
In this article, we will delve into the intricacies of Python MRO, explore its significance in inheritance, and provide clear code examples to illustrate its functionality.
What is Python MRO?
Python MRO stands for Method Resolution Order, which is the order in which Python searches for methods in a hierarchy of classes. When a method is called on an object, Python needs to determine which class’s method to execute, especially in scenarios involving multiple inheritance. The MRO ensures that the search for the method is done in a predictable manner.
Python uses the C3 linearization algorithm to establish the MRO. This algorithm ensures that the method resolution follows a specific order, which is vital for maintaining the integrity of the class hierarchy. The MRO can be accessed using the __mro__
attribute or the mro()
method on a class.
How to Access MRO in Python
To see the MRO of a class, you can use the __mro__
attribute or the mro()
method. Both methods provide the same output. Here’s a simple example demonstrating how to access the MRO of a class.
class A:
pass
class B(A):
pass
class C(A):
pass
class D(B, C):
pass
print(D.__mro__)
Output:
(<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
In this example, we define a class hierarchy with classes A, B, C, and D. The print(D.__mro__)
statement outputs the MRO for class D. As you can see, Python lists the classes in the order it will search for methods, starting from D and moving down to the base class object
.
This output indicates that when a method is called on an instance of D, Python will first look for that method in D, then in B, followed by C, A, and finally in the base class object
. Understanding this order is essential for effectively using multiple inheritance in Python.
The Significance of MRO in Multiple Inheritance
Multiple inheritance can lead to ambiguity, particularly when two parent classes define the same method. MRO helps resolve this ambiguity by providing a clear and consistent order for method resolution. This ensures that the method defined in the most derived class is executed first, followed by methods in parent classes, according to the defined MRO.
Consider the following example where two parent classes define the same method:
class A:
def show(self):
print("A's show method")
class B(A):
def show(self):
print("B's show method")
class C(A):
def show(self):
print("C's show method")
class D(B, C):
pass
d = D()
d.show()
In this case, class D inherits from both B and C. When we create an instance of D and call the show
method, Python uses the MRO to determine which method to execute. Since B appears before C in the MRO, the output is “B’s show method”. This illustrates how MRO provides a systematic approach to method resolution, avoiding confusion and ensuring that the correct method is called.
Customizing MRO with super()
The super()
function is a powerful tool in Python that allows you to call methods from a parent class, adhering to the MRO. This is particularly useful in scenarios where you want to ensure that the method resolution follows the established order. Here’s how you can use super()
to customize method calls in a multi-inheritance scenario.
class A:
def show(self):
print("A's show method")
class B(A):
def show(self):
print("B's show method")
super().show()
class C(A):
def show(self):
print("C's show method")
super().show()
class D(B, C):
pass
d = D()
d.show()
Output:
B's show method
C's show method
A's show method
In this example, when we call d.show()
, Python first executes the show
method in class B, which then calls super().show()
. This triggers the show
method in class C, which also calls super().show()
, leading to the execution of the method in class A. The output reflects the MRO, demonstrating how super()
can be used to maintain the order of method resolution.
Conclusion
Understanding Python’s Method Resolution Order (MRO) is essential for anyone working with object-oriented programming in Python, especially when dealing with multiple inheritance. MRO provides a systematic approach to resolving method calls, ensuring that the correct methods are executed in a predictable order. By using tools like super()
, you can leverage MRO to create flexible and maintainable class hierarchies. With this knowledge, you can navigate the complexities of inheritance with confidence, making the most out of Python’s powerful features.
FAQ
- What is MRO in Python?
MRO stands for Method Resolution Order, which defines the order in which Python looks for a method in a hierarchy of classes.
-
How can I access the MRO of a class?
You can access the MRO of a class using the__mro__
attribute or themro()
method. -
Why is MRO important in multiple inheritance?
MRO is important because it resolves ambiguities in method calls when multiple parent classes define the same method. -
How does
super()
work with MRO?
Thesuper()
function calls the next method in the MRO, allowing you to maintain the defined order of method resolution. -
Can MRO be customized?
While MRO follows a specific algorithm, you can influence method calls through the use ofsuper()
to ensure that methods are executed in the desired order.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn