How to Mock Return Value Based on Input in Python
- Mock Return Value Based on Input in Python
- Uses of Mocking in Python
- Use Mock Objects in Python
- The Meaning of Mocking in Python
- Write a Mock That Returns Different Values Based on the Input
- Python Mock That Returns Based on Input
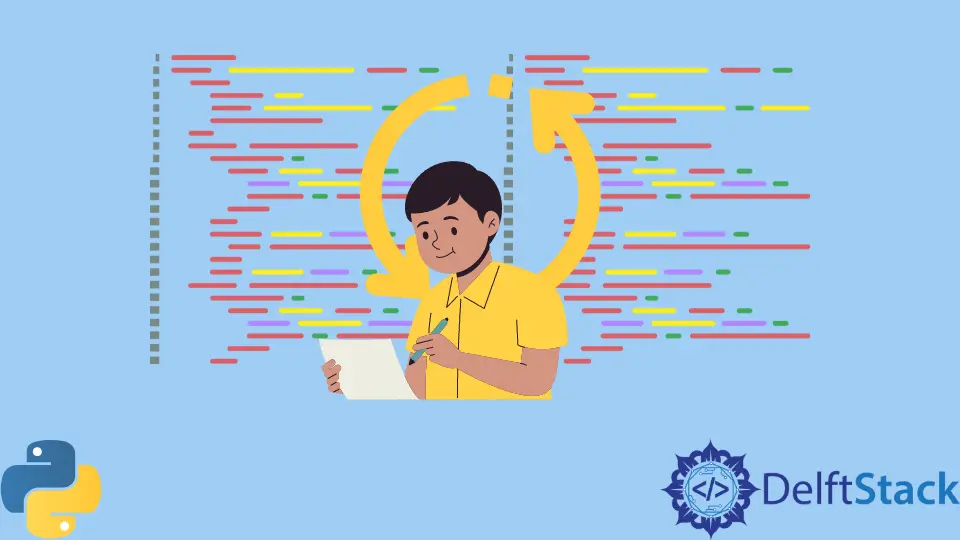
This article will demonstrate how to create a mock function that returns different values based on input in Python. We’ll also show you how to test our mock function.
Finally, we’ll show you some more advanced techniques for mocking functions. You do not need to know about programming or testing to follow along.
Mock Return Value Based on Input in Python
Python is an incredibly powerful language with a rich ecosystem of testing tools. It has many features that make it perfect for unit testing.
One popular tool is the mock
module, which allows us to replace parts of our system with mock objects and assert how they were used.
Mocking functions allow us to replace the functionality of a function with our code. This can be useful when we want to test the behavior of our code when a function is unavailable or when we want to test how our code reacts to different inputs.
Uses of Mocking in Python
Mocking is a powerful technique that can be used to improve the reliability and efficiency of our tests. This article will teach us how to use mock objects in our Python tests.
-
Mocking allows us to replace real objects with simulated versions. This can be useful when we want to test a component that depends on another component that is not yet available.
For example, we might want to test a database connection without actually connecting to a database.
-
Mocking can also be used to stub out external dependencies. This can be useful when we want to avoid making network calls or interacting with databases during our tests.
-
Mocking can be used to improve the efficiency of our tests. When we mock an object, we can avoid the overhead of instantiating the real object.
This can be particularly useful when testing code that instantiates many objects.
-
Mocking can also be used to make our tests more reliable. Stubbing out external dependencies can avoid flaky tests that rely on network or database availability.
Use Mock Objects in Python
To use mock objects in our tests, we first need to create a Mock class. This class can be used to create mock objects that look and behave like the real thing.
Once we have a Mock class, we can instantiate mock objects and use them in our tests. Mock objects have all the same methods as the real objects they’re replacing, so we can use them just like we would use the real thing.
When we are finished using a mock object, we can assert that it was used correctly. For example, we can assert that a mock database connection was used to execute a query.
Hence, mocking is a powerful tool that can be used to improve the reliability and efficiency of our Python tests.
By replacing real objects with mock objects, we can avoid the overhead of instantiating the real object. We can also stub out external dependencies to make our tests more reliable.
The Meaning of Mocking in Python
The word mocking
is used in several contexts; however, the following definition will be used throughout this document:
“The process of substituting fake calls or objects for one or more actual function calls or objects.”
A call to a mock function delivers an instantaneous value that has been preset without actual work. The characteristics and methods of a fake object are defined in the same manner fully inside the test without first generating the actual object or doing any other work.
When it comes to testing, the fact that the person who wrote the test can define the return values of the function call gives them tremendous power. However, it also means that the person writing the test needs to do some foundational work to get everything set up accurately.
The unittest.mock
module in Python is what’s responsible for mocking in the programming language. The patch function, which acts as a decorator and context manager, and the MagicMock
class, are two essential classes and functions in this module.
It also includes several other helpful classes and methods. Most of the work involved in mocking Python may be done by using these two robust components.
Write a Mock That Returns Different Values Based on the Input
Now that we are well aware of mock and its functionalities. Let’s understand mock better with coding examples.
To create the function, we will use *args
and **kwargs
because these parameters take simple and keyword arguments. So, we can pass any data type: string, integer, float and Boolean, and we can assign values to keyword arguments too.
-
Create a function.
-
Pass arguments
*args
and keyword argument**kwargs
-
Return a tuple having
*args
and**kwargs
-
Call the function
-
Print the result
Look at the code below for a better understanding.
def mock_function(*args, **kwargs):
return (args, kwargs)
mock_function("x", "y")
(("x", "y"), {})
mock_function("x", y=2, z="mock test")
Output:
(('x',), {'y': 2, 'z': 'mock test'})
In the code output, we can see that the *args
value is printed first, which is x
and **kwargs
value {'y': 2, 'z': 'mock test'})
is printed after it.
Python Mock That Returns Based on Input
Now we will create a mock that returns the function we discussed earlier.
-
To create a mock function, we will first import
MagicMock
fromunittest.mock
module. -
Create a variable and use the
MagicMock
fromunittest.mock
. -
Print the result.
Example code:
from unittest.mock import MagicMock
my_mock_1 = MagicMock(name="abid", return_value=mock_function)
my_mock_1
Output:
<MagicMock name='abid' id='139916486559696'>
The code output shows that the MagicMock
command is used. And we can see the name abid
we used in the code and an id number generated by the code.
Now we will create another variable to see where is our variable my_mock_1
.
Example code:
func_from_mock2 = my_mock_1()
func_from_mock2
Output:
<function __main__.mock_function(*args, **kwargs)>
Code output shows that the function carries arguments *args
and keyword argument **kwargs
.
Now we will give some values to our variable func_from_mock2
. So we will pass 10, 10
and print it.
Example code:
func_from_mock2(10, 10)
Output:
((10, 10), {})
The output ((10, 10))
means we have used arguments *args
and {}
means we can use keyword argument **kwargs
.
Now we will use the keyword argument **kwargs
.
Example code:
func_from_mock2(15, 18, param=50)
Output:
((15, 18), {'param': 50})
We have used both arguments *args
and keyword argument **kwargs
. ((15, 18),
shows the argument part of the mock input and {'param': 50})
shows the keyword argument part of the mock input.
So, this is how Mock
returns different values based on input.
We hope you find this Python article helpful in understanding how to use Mock
in Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn