kwargs in Python
-
Use
**kwargs
to Let Functions Take Arbitrary Number of Keyword Arguments in Python -
Use
*l
Argument to Unpack Arguments While Calling a Function in Python
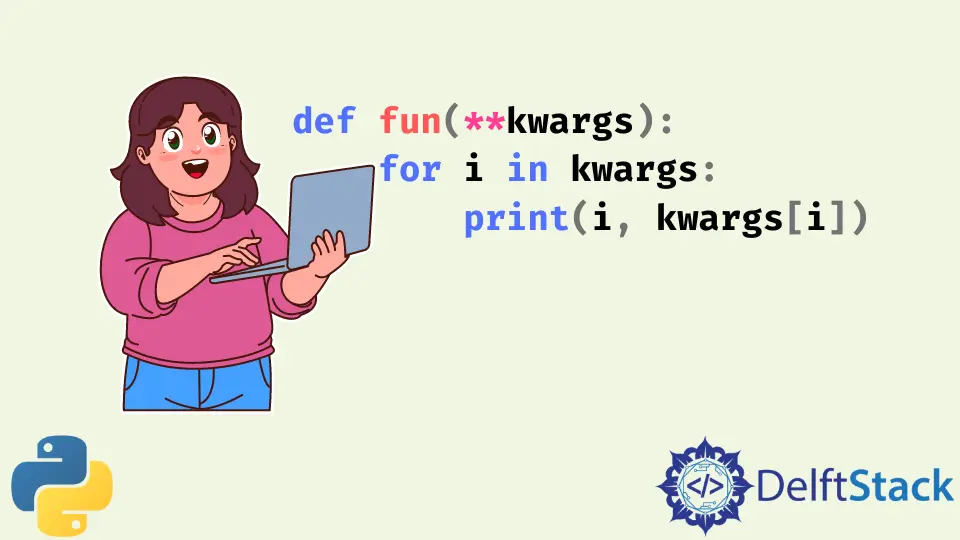
We can provide different arguments for a function. These arguments are used in the function to perform some desired work.
In programming, we specify the arguments in parenthesis with the function name. We can specify the required number of arguments in the function definition.
Use **kwargs
to Let Functions Take Arbitrary Number of Keyword Arguments in Python
There are keyword, positional, and arbitrary arguments in Python. Python provides an interesting feature of **kwargs
.
It is a standard notation that stands for keyword arguments. We can provide an arbitrary number of arguments to a function using this.
Here kwargs
is just a variable name and can be substituted with any other valid variable name. The main part here is the **
operator.
The **
is called the unpacking operator in Python. We use it to unpack dictionaries in Python.
We also have the *
operator that can unpack lists, tuples, and other iterables that can use both for providing an arbitrary number of arguments to a function.
The main concept behind kwargs
is that the function will receive these arguments packed into an object. It will unpack all the elements and use them in the function as desired.
First, let us demonstrate this with the *
operator. As discussed, it is used to unpack elements from an iterable like a list or a tuple.
The most basic way to use this is to provide *kwargs
in the function definition. This way, we can specify any desired number of arguments to the function.
def fun(*l):
for i in l:
print(i)
fun(1, 2, 3)
Output:
1
2
3
Use *l
Argument to Unpack Arguments While Calling a Function in Python
In the above example, we used the function’s *l
argument to unpack multiple arguments and print them.
Similarly, the *l
can be used to unpack arguments while calling a function. Here the roles are reversed.
def fun(x, y):
print(x, y)
l = [5, 7]
fun(*l)
Output:
5 7
In the above example, we could not have directly sent a list to the function. So we unpack it while calling the function.
Now let us discuss the **kwargs
. Using this method, we can provide many keyword arguments to a function.
The arguments are passed as key-value pairs of a dictionary and unpacked in the function using the **
operator.
def fun(**kwargs):
for i in kwargs:
print(i, kwargs[i])
fun(a=1, b=2, c=3)
Output:
a 1
b 2
c 3
We used the **kwargs
to pass multiple keyword arguments to the function in the above example.
We can also use both these methods to specify multiple arguments and positional arguments simultaneously.
def fun(x, **kwargs):
print("Multiple Keyword Arguments")
for i in kwargs:
print(i, kwargs[i])
print("Positional arguments", x)
fun(10, a=1, b=2, c=3)
Output:
Multiple Keyword Arguments
a 1
b 2
c 3
Positional arguments 10
It is important to ensure the order of keyword arguments because, in Python 3.6, their insertion order is remembered.
We can also use the *
operator for extended iterable unpacking in Python 3. We can use the operator on the left side of an assignment statement.
We can use this also to provide multiple arguments at function calls.
def fun(x, z, *l):
print("Multiple Arguments")
for i in l:
print(i)
print("Positional arguments", x, z)
x, *y, z = [1, 2, 3, 4]
fun(x, z, *y)
Output:
Multiple Arguments
2
3
Positional arguments 1 4
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn