Best Practices for Kwargs Parsing in Python
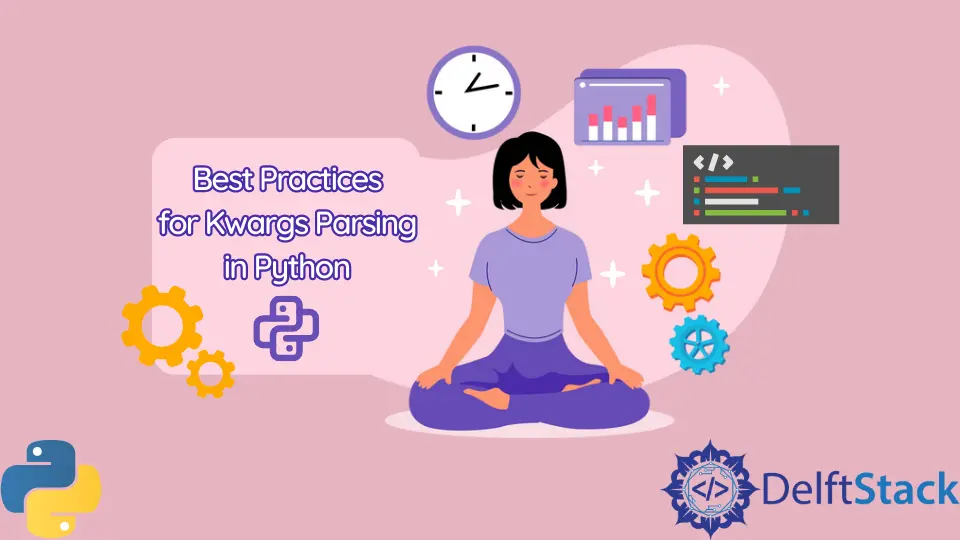
Using kwargs
parsing, a developer can easily extract the necessary information from a group of keywords and arguments. This is often used when a developer wants to specify a set of options or settings.
These are the three methods of kwargs
parsing:
- By using the built-in function
vars()
. - By using the
**
operator. - By using the
get()
method.
kwargs
in Python
Python kwargs
is a keyword argument that allows us to pass a variable number of keyword arguments to a function. The keyword ideas are passed as a dictionary to the function.
The following are a few best practices to remember when using keyword arguments (kwargs)
in Python.
- First,
kwargs
should be used sparingly and only when necessary. - Second,
kwargs
should be used in conjunction with positional arguments(args)
whenever possible. - Third,
kwargs
should be explicit and well-documented.
When used correctly, kwargs
can improve the readability and maintainability of your Python code; however, when used excessively or without proper documentation, kwargs
can make your code difficult to understand. When in doubt, err on caution and use args
instead of kwargs
.
Use kwargs
in Python
Python kwargs
are a powerful tool for creating flexible functions and methods, allowing you to pass an arbitrary number of keyword arguments to a function or method. kwargs
are often used to set default values for function arguments.
To use kwargs
in your functions and methods, add the **kwargs
keyword to the parameter list. Then, you can access the keyword arguments using the dictionary syntax.
For example, if you have a function that takes two keyword arguments, you can access them like this:
def my_func(**kwargs):
arg1 = kwargs["arg1"]
arg2 = kwargs["arg2"]
You can also use kwargs
to set default values for function arguments. For example, if you have a function that takes an optional keyword argument, you can set a default value like this:
def my_func(**kwargs):
arg1 = kwargs.get("arg1", "default_value")
This function will set arg1
to default_value
if the arg1
keyword argument is not passed to the function.
kwargs
are a powerful tool for creating flexible functions and methods. They can make your code more readable and easier to maintain.
Methods for kwargs
Parsing in Python
There are many ways to parse kwargs
in Python, but not all are best practices. When parsing kwargs
, it’s essential to be careful about typecasting and using keyword-only arguments to avoid confusion.
kwargs
Parsing by the **
Operator in Python
Another way to parse kwargs
is to use the **
operator. This operator unpacks a dictionary into keyword arguments.
This method is more explicit than using vars()
and can help avoid potential typecasting errors.
def intro(**data):
for key, value in data.items():
print("{} is {}".format(key, value))
intro(Firstname="Zeeshan", Lastname="Afridi", Age=24)
print("\n")
intro(
Firstname="Zeeshan",
Lastname="Afridi",
Email="zeeshan.afridi@example.com",
Country="Pakistan",
Age=24,
Phone=+923331234567,
)
Output:
formal arg: 1
keyword arg: myarg2: 2
keyword arg: myarg3: 3
Conclusion
Using kwargs
, we can write functions that take an arbitrary number of keyword arguments. This can be useful when we want to provide a flexible interface to a function.
For example, we might want to write a function that logs a message to a file. We might want to be able to specify the log level and the message to be logged.
Using kwargs
, we can write a function that takes these arguments as keyword arguments.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn