How to Run Python in HTML
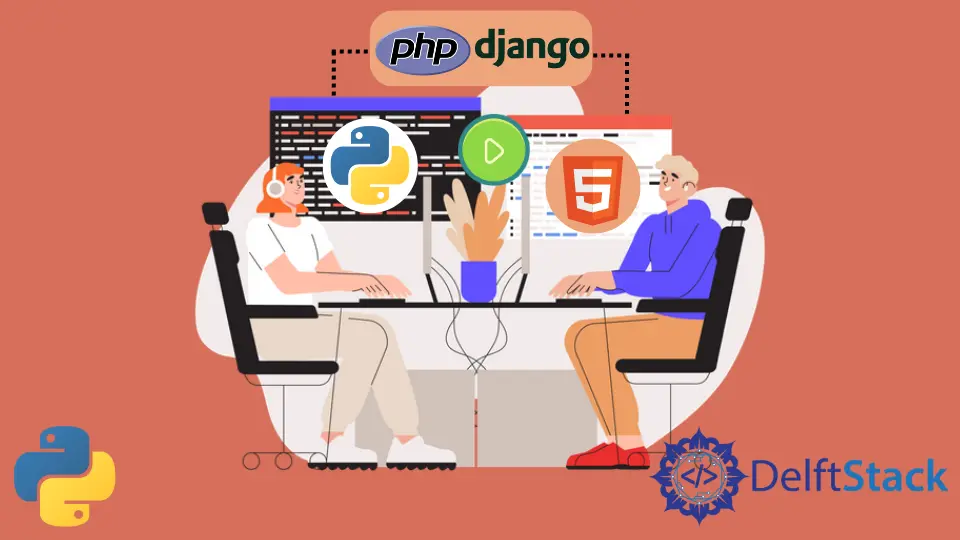
Web Development is a vast field, and there are endless opportunities and things that we can do. With complexity and demand come requirements. When building dynamic web pages, we often have to perform tasks that require the assistance of some programming language such as Python or PHP.
In this article, we will learn how to run a Python script in HTML. We will talk about a few ways in which we can achieve this.
Run Python Scripts in HTML using PHP
We can use PHP
or Hypertext Preprocessor
to run Python scripts in HTML. Refer following code depicts a simple example.
HTML File - index.html
<html>
<head>
<title>Running a Python script</title>
<?PHP
echo shell_exec("python script.py");
?>
</head>
<body>
<!-- BODY -->
</body>
</html>
Python File - script.py
a = 2000
b = 21
print(f"a = {a}")
print(f"b = {b}")
print(f"a + b = {a + b}")
This will print the following in the console.
a = 2000
b = 21
a + b = 2021
If we want to pass some values to the Python scripts, we can use the following code.
HTML File - index.html
<html>
<head>
<title>Running a Python script</title>
<?PHP
echo shell_exec("python script.py \"Parameter #1\" \"Parameter #2\"");
?>
</head>
<body>
<!-- BODY -->
</body>
</html>
Now, the Python script will look as follows.
Python File - script.py
import sys
a = sys.argv[1]
b = sys.argv[2]
print(f"a = {a}")
print(f"b = {b}")
print(f"a + b = {a + b}")
The output will remain the same, as shown above.
Run Python script in HTML using Django
Django is a famous and robust Python-based web development framework. Since it is Python-based, it makes it easier to run Python scripts inside the HTML. The way we do this is by using template tags. Django has some pre-built template tags such as date
, linebreaks
, safe
, random
, etc. You can learn more about them here.
Since Django is very customizable, it offers developers an easy way to create their custom template tags. Using template tags, we can return data to HTML templates, which can be embedded inside the HTML template.
Follow the following steps to create a simple template tag. We are assuming that we have a core
application in our Django project.
-
Create a new directory,
templatetags
, inside thecore
application. The app directory should look something like this.core/ __init__.py models.py admin.py views.py urls.py ... templatetags/ __init__.py ...
-
Inside the
templatetags
folder, create a Python file namedmy_custom_tags.py
. -
Inside this file, add the following code.
from django import template register = template.Library() @register.simple_tag def my_tag(): return "Hello World from my_tag() custom template tag."
my_custom_tags.py
module will hold all the custom template tags. As shown in the code above,my_tag
is a custom template tag that we just created and now it can be used inside any HTML template. This template tag will return"Hello World from my_tag() custom template tag."
this string to the template. We can create even more template tags here to perform specific and common tasks. -
Now that we have created our first template tag, it is time to load it inside our HTML template and use it.
{% load static %} {% load my_custom_tags %} <!DOCTYPE html> <html lang="en" dir="ltr"> <head> <title>Intro</title> </head> <body> <p>{% my_tag %}</p> </body> </html>
We first load the
my_custom_tags.py
module inside the template. Once the module is loaded, we can now use the template tags defined inside themy_custom_tags
module. Note that it is important to first load a module with custom template tags before using those template tags.
Instead of using a template tag, we can also create an end-point and make an AJAX request to that end-point to perform some task or get some data. We can use fetch()
or jquery
or any other available method to make an AJAX request. While making an end-point to handle an AJAX request, it is important to ensure that the end-point is secure and doesn’t give easy access to sensitive data or website features. Since anyone can make AJAX requests if they know the end-point, we can add CSRF (Cross Site Request Forgery
) validation to it and configure it to handle only POST requests. The POST data should contain the CSRF token.
You can learn more about CSRF here