How to Calculate Average and Grade in Python
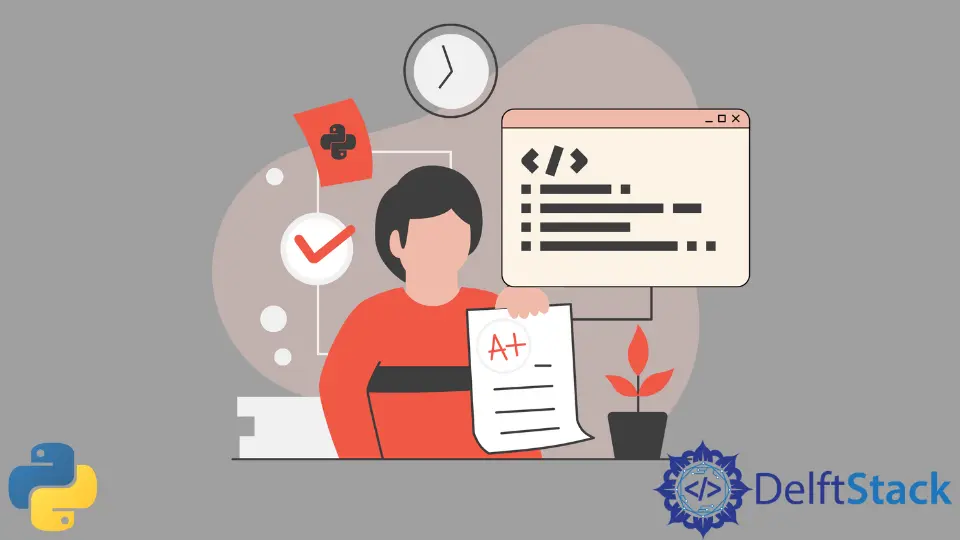
In this article, we’ll explore a Python program that tackles two essential tasks: calculating the average marks for five subjects and assigning grades based on a predefined grading scale.
This program is particularly useful for students looking for a simple solution to their school assignments or for those interested in creating a grading system for educational purposes.
Calculate Average and Grade in Python
This test average and grade Python program has two main tasks: calculate the average marks against 5 subjects and calculate the grade against the average marks.
Code example:
def calculate_average(total):
return total / 5
def find_score(grade):
if 90 <= grade <= 100:
return "A"
elif 80 <= grade <= 89:
return "B"
elif 70 <= grade <= 79:
return "C"
elif 60 <= grade <= 69:
return "D"
else:
return "F"
scores = []
for i in range(1, 6):
score = int(input("Enter score {0}: ".format(i)))
print("That's a(n): " + find_score(score))
scores.append(score)
total = sum(scores)
avg_marks = calculate_average(total)
final_grade = find_score(avg_marks)
print("Average grade is: " + str(avg_marks))
print("That's a(n): " + str(final_grade))
Let’s explain the code above. We have two main functions, the calculate_average(total)
and the find_score(grade)
.
The calculate_average(total)
calculates the average of the total marks provided as input. The find_score(grade)
assigns a grade (A
, B
, C
, D
, or F
) based on the input grade using conditional logic.
Inside a loop, we prompt the user to enter marks for 5 subjects one by one. It also displays the corresponding grade using the find_score()
function.
The entered scores are stored in the scores[]
list. After we enter all subject marks, the program calculates the total sum of the scores.
It then calculates the average marks by calling the calculate_average()
function and passing the total as an argument. Finally, we used the find_score()
function to assign a grade to the calculated average and display both the average and the corresponding grade to the user.
Output:
Enter score 1: 99
That's a(n): A
Enter score 2: 98
That's a(n): A
Enter score 3: 78
That's a(n): C
Enter score 4: 95
That's a(n): A
Enter score 5: 87
That's a(n): B
Average grade is: 91.4
That's a(n): A
In the output, the program summarizes our input by displaying grades for individual subjects based on a predefined scale immediately after each input. After all five subject marks are entered, the program calculates and displays the average mark and the corresponding final grade based on the average.
Conclusion
In this article, we’ve discussed how to calculate averages and assign grades in Python, making it valuable for both students and educators alike. The program stands out for its well-organized functions, ease of use in taking user inputs and providing feedback, and flexibility in allowing customization of the grading scale.
With its clear and understandable output and its adaptability, it serves as an invaluable resource for educational contexts. Moreover, it can be extended for more complex grading systems or specialized educational applications.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn