How to Get CPU Temperature using Python
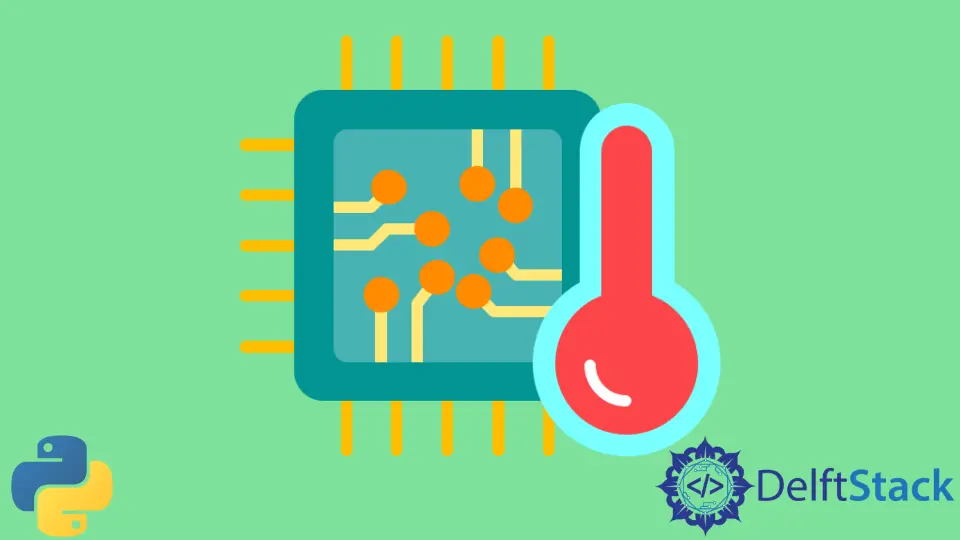
The main aim of this article is to demonstrate how to read and show CPU temperature with the help of the pythonnet
library in Python.
Python Get CPU Temperature
Based on what type of application you are designing, it can be a possibility that you might want to monitor the resources of the machine the program is running on.
This situation can arise due to plenty of reasons. Perhaps you need your program to act a certain way when the system resources reach a certain threshold.
The use cases can be different from program to program.
Out of these system resources, CPU temperature holds significant importance in specific applications and use cases.
Perhaps your program is straining the CPU too much and hogging up many unnecessary resources. You might want to take measures to mitigate this issue, due to which you probably need to monitor the temperature of different machine components, the CPU being one of them.
To solve this specific issue, we can use the DLLs (Dynamic Link Libraries) provided by OpenHardwareMonitor.
This section can be divided into the following parts:
- Installation
- Implementation
- Monitor
Installation
First of all, we need to download pythonnet
to be able to interface with the DLLs. To do so, execute the following command in your terminal.
pip install pythonnet
This will give the following output:
Collecting pythonnet
Downloading pythonnet-3.0.0.post1-py3-none-any.whl (279 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 279.4/279.4 kB 639.3 kB/s eta 0:00:00
Requirement already satisfied: clr-loader<0.3.0,>=0.2.2 in c:\program files\python310\lib\site-packages (from pythonnet) (0.2.4)
Requirement already satisfied: cffi>=1.13 in c:\program files\python310\lib\site-packages (from clr-loader<0.3.0,>=0.2.2->pythonnet) (1.15.1)
Requirement already satisfied: pycparser in c:\program files\python310\lib\site-packages (from cffi>=1.13->clr-loader<0.3.0,>=0.2.2->pythonnet) (2.21)
Installing collected packages: pythonnet
Successfully installed pythonnet-3.0.0.post1
After you have successfully installed pythonnet
, it’s time to download OpenHardwareMonitor. Download the software from here.
After you have done downloading, install the application. After which:
-
Go to the directory where you installed OpenHardwareMonitor.
-
Find
OpenHardwareMonitorLib.dll
from the files present inside the folder. -
Copy to your desired folder, preferably where your python script is stored.
Implementation
After the installation process is completed, it is time to implement the actual code.
Consider the following code:
import clr # package pythonnet, not clr
openhardwaremonitor_sensortypes = [
"Voltage",
"Clock",
"Temperature",
"Load",
"Fan",
"Flow",
"Control",
"Level",
"Factor",
"Power",
"Data",
"SmallData",
]
def initialize_openhardwaremonitor():
file = "D:\\Path_TO_DLL\\OpenHardwareMonitorLib.dll"
clr.AddReference(file)
from OpenHardwareMonitor import Hardware
handle = Hardware.Computer()
handle.MainboardEnabled = True
handle.CPUEnabled = True
handle.RAMEnabled = True
handle.GPUEnabled = True
handle.HDDEnabled = True
handle.Open()
return handle
def fetch_stats(handle):
for i in handle.Hardware:
i.Update()
for sensor in i.Sensors:
parse_sensor(sensor)
for j in i.SubHardware:
j.Update()
for subsensor in j.Sensors:
parse_sensor(subsensor)
def parse_sensor(sensor):
hardwaretypes = openhardwaremonitor_hwtypes
if sensor.Value is not None:
if str(sensor.SensorType) == "Temperature":
print(
u"%s %s Temperature Sensor #%i %s - %s\u00B0C"
% (
hardwaretypes[sensor.Hardware.HardwareType],
sensor.Hardware.Name,
sensor.Index,
sensor.Name,
sensor.Value,
)
)
if __name__ == "__main__":
print("OpenHardwareMonitor:")
HardwareHandle = initialize_openhardwaremonitor()
fetch_stats(HardwareHandle)
Using the clr
module, we can interface with the .NET DLL named OpenHardwareMonitorLib.dll
. We can continue to use its functions and attributes in our Python code and finally read the temperature of the CPU and other components we may wish to view.
For a detailed overview of what each attribute stands for and its function, you may refer to the documentation of OpenHardwareMonitor on Github.
For any other custom functionality, it is recommended to look at the code to gain a better understanding and insight into the internal workings of the code.
Monitor
After we have written the code, it is time to execute the program. Remember that you need to run this script as an administrator; otherwise, the code will not run properly and may not be able to show the necessary readings correctly or not show them at all.
Open the command prompt or any terminal you choose and execute the script. For the code above, the following output is shown when run as an administrator:
OpenHardwareMonitor: CPU Intel Core i7-4800MQ Temperature Sensor #0 CPU Core #1 - 60.0°C CPU Intel Core i7-4800MQ Temperature Sensor #1 CPU Core #2 - 66.0°C CPU Intel Core i7-4800MQ Temperature Sensor #2 CPU Core #3 - 58.0°C CPU Intel Core i7-4800MQ Temperature Sensor #3 CPU Core #4 - 58.0°C CPU Intel Core i7-4800MQ Temperature Sensor #4 CPU Package - 66.0°C GpuNvidia NVIDIA Quadro K1100M Temperature Sensor #0 GPU Core - 43.0°C HDD ST500LT012-9WS142 Temperature Sensor #0 Temperature - 37.0°C
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn