File Naming Conventions in Python
- Naming Convention for Functions in Python
- Naming Convention for Variable in Python
- Naming Convention for Class in Python
- Naming Convention for File in Python
- Method Naming Convention in Python
- Module Name in Python
- Global Variable Naming Convention in Python
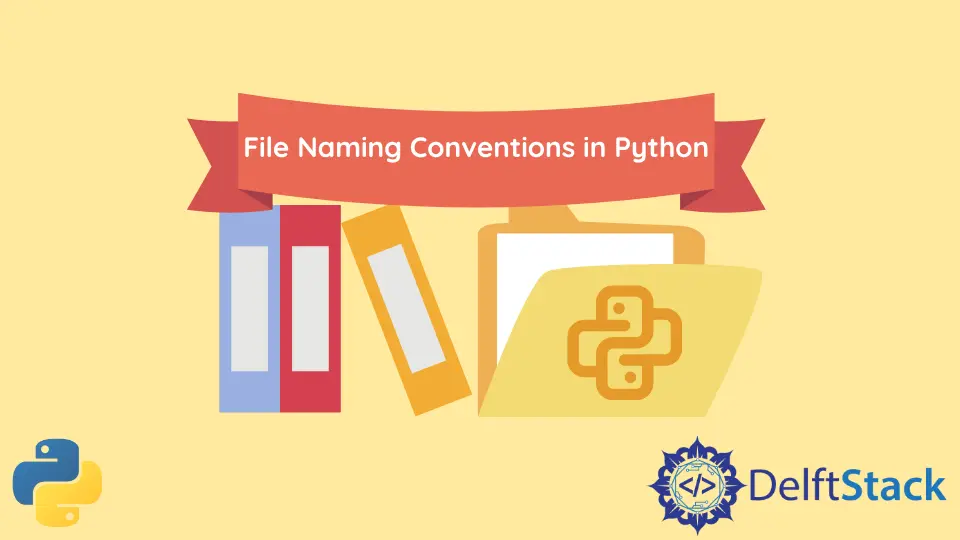
We will introduce Python naming conventions and discuss how to use naming conventions in Python when class, variable, functions, object, file name, module, constant, package, global, and variable are concerned.
We can name variables in many different ways, and Python has made it easy. The Naming convention is essential in the programming language, making tasks easy and fast.
Naming Convention for Functions in Python
We can’t just name a function in Python. Some evident rules must be fulfilled while naming a function in Python.
While writing the Python function name, we should only use all lower case characters. No uppercase characters should be used.
When naming a function, it is permissible to use underscore(_)
between the words as a substitute for space.
Code:
# python
def my_testFunction():
print("This is a test function using underscore!")
def our_Criticfunction():
print("This is a critic function.")
def newData_func():
print("This is a better function name.")
my_testFunction()
our_Criticfunction()
newData_func()
Output:
Naming Convention for Variable in Python
It is the same as that of function names. Some rules are necessary to be followed when naming a Python variable.
The rules are as follows.
- It is mandatory to start a variable with an alphabet or
underscore(_)
character. - A variable name should only be
A-Z,a-z,0-9
andunderscore(_)
. - A
number
shouldn’t be the start of a variable name. - Unique characters such as
$,%,#,&,@.-,^,
etc., are prohibited from using with the variable name. - Variable names are case-related. For example,
trs
andTrs
are two distinctive variables. - Keywords like
class, for, def, del, is else, try, and from
should be avoided while naming a variable.
The following example is about which names are allowed in Python, as shown below.
# python
# Variable Names that are allowed
a = 2
b = "Hello"
pythonVariable = "Python Tutorial"
python_variable = "Python Tutorial"
_python_variable = "Python Tutorial"
_pythonVariable = "Python Tutorial"
PYTHONVARIABLE = "Python Tutorial"
pythonVariable = "Python Tutorial"
pythonVairbale3 = "Python Tutorial"
Let’s discuss the names that are not allowed in Python.
# python
# Variable Names that are not allowed
7pythonvariable = "Python Tutorial"
-pythonvariable = "Python Tutorial"
pythonv@riable = "Python Tutorial"
python variable = "Python Tutorial"
for = "Python Tutorial"
If we have used variables that are not allowed, it will show invalid syntax. It will process them one by one, and as a result, an error will appear.
Output:
Naming Convention for Class in Python
The naming convention for classes in Python is identical to what it is for other programming languages, as there were rules in the case of variable and function. We also have to follow specific rules while naming classes in python as well.
The name determines the identity of anything. A unique name enhances your outlook.
So we should assign a proper name to a class as the program begins with the class. The rules are as follows.
CamelCase
convention should be followed.- In the case of classes with the exception, while writing then, we should end the name with an
Error
. - We can assign a class name like a function if we summon the class from someplace or callable.
- The classes within python are in lowercase.
Code:
# python
class OurClass
class HelloWorld
class UserError
Naming Convention for File in Python
When we are deciding on a name for your file, you need to keep the following rules in mind.
- A file name chosen should be a short name.
- All lowercase should be used when selecting a name for the file.
- The file name can also contain an
underscore()
.
Method Naming Convention in Python
Naming a method in python, follow the rules below.
- When selecting a name for a method, you should choose all
lowercase
. - To separate multiple words, if your method name had any, you should use an
underscore(_)
. - A name that is not for the public should begin with an
underscore()
. - Two
underscores(_)
should be used at the start when you wish to mangle a method name.
Constant Naming Convention in Python
Specific rules should be followed to name a constant in python.
- Always
capitalize
the constant name in Python. - To separate multiple words, if your constant name had any, we should use an
underscore(_)
.
Package Naming Convention in Python
Following are the rules that we should follow when naming a package.
- When selecting a name for a package, we should choose all
lowercase
. - To separate multiple words, we should use an
underscore(_)
if our method name had any. - It is beneficial to use a
single word
when naming a package.
Object Naming Convention in Python
When naming an object in python, we should follow the following rules.
- When selecting a name for an object, we should choose all
lowercase
. - The name chosen should be very
short
. - To separate multiple words, we should use an
underscore(_)
if our method name had any.
Module Name in Python
Some rules are necessary to be followed when you are naming a module in python.
- When selecting a name for a module, we should choose all
lowercase
. - To separate multiple words, if your module name had any, you should use an
underscore(_)
. - It is beneficial to use a
single word
when naming a module.
Global Variable Naming Convention in Python
Following are the rules for naming a global variable.
- When selecting a name for a global variable, you should choose all
lowercase
. - To separate multiple words, if your global variable had any, you should use an
underscore(_)
.
Arguments of a Method
For instance, methods use self
as the first argument. In the case of the class method, cls
should be used as a beginning argument.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn