How to Extend Python Dictionary
-
Using the
update()
Method to Extend a Dictionary in Python - Using the ** Operator to Extend a Python Dictionary
- Using Dictionary Comprehension to Extend a Python Dictionary
-
Using
collections.Chainmap
to Extend a Python Dictionary
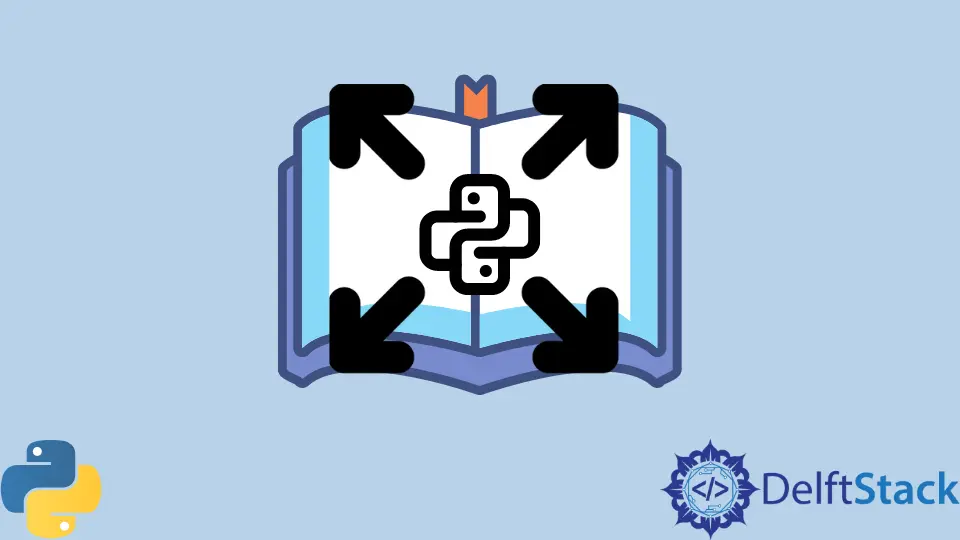
This article will show the user how to extend a python dictionary with another dictionary. A dictionary is a container of data that can be changed (is mutable), and it stores this data in the form of key-value pairs example {key: 'value'}
.
You can extend a dictionary with another element by concatenation. It means all the key-value pairs in one dictionary will be added to the other one.
Using the update()
Method to Extend a Dictionary in Python
The update()
method is one of the ways Python uses to implement dictionary concatenation. It is done by adding another dictionary’s key-value pair elements to the current dictionary’s end.
This method is especially useful when extending a dictionary that has different key-value pairs from another dictionary. Otherwise, using update()
will overwrite the existing values in the first dictionary with the elements in the second dictionary.
In simpler terms, the values in the second defined dictionary overwrite those in the first one because of overlapping.
first_dict = {"name": "Yolanda", "major": "Bsc Comp Science", "age": 23}
second_dict = {"name": "Beatrice", "major": "Bsc Comp Science", "age": 43}
first_dict.update(second_dict)
print(first_dict)
Output:
{'name': 'Beatrice', 'major': 'Bsc Comp Science', 'age': 43}
The above dictionaries have identical key
values, which are name
. Using update()
to concatenate these data stores results in the name
key value for the first_dict
to be overwritten by that of second_dict
. Therefore, it results in a single dictionary as displayed in the output.
However, when extending one dictionary with values that are not defined already, update()
displays a single dictionary with all key-value pairs from both the defined dictionaries.
Example:
dict_one = {'u':2, 'v':4, 'w':7}
dict_two = {'x':5, 'y':6, 'z': 8}
dict_one.update(dict_two)
print(dict_one)
Output:
{'u': 2, 'v': 4, 'w': 7, 'x': 5, 'y': 6, 'z': 8}
Using the ** Operator to Extend a Python Dictionary
Using the asterik
operator in a one-line statement would look like this:
dict(iterable, **kwargs)
iterable
is the first defined dictionary, and the second parameter is the second dictionary (keyword parameter).
Example
y = {"a": 5, "b": 6}
z = {"c": 7, "d": 9}
x = dict(y, **z)
print(x)
Output:
{'a': 5, 'b': 6, 'c': 7, 'd': 9}
If there is a key-value pair overlap in the defined dictionaries, the second dictionary’s items overlap those in the first dictionary the same way we saw in update()
.
Example:
first_dict = {"a": 2, "b": 3, "c": 5}
second_dict = {"c": 4, "e": 6, "f": 7}
dictt = dict(first_dict, **second_dict)
print(dictt)
Output:
{'a': 2, 'b': 3, 'c': 4, 'e': 6, 'f': 7}
It is also important to note that the argument names and the keys in the **dictionary
items must be of string data type. Else, a Typerror exception will be displayed.
Example:
first_dict = {"a": 2, "b": 3, "c": 5}
second_dict = {"c": 4, "e": 6, 7: 7}
dictt = dict(first_dict, **second_dict)
print(dictt)
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: keywords must be strings
Using Dictionary Comprehension to Extend a Python Dictionary
This transformation method transforms one Python dictionary to another by conditionally including items in an original dictionary to a new one. It works similarly to the use of asterisks like above.
first_dict = {"a": 2, "b": 3, "c": 5}
second_dict = {"c": 4, "e": 6, "f": 7}
the_dictionary = {**first_dict, **second_dict}
print(the_dictionary)
Output:
{'a': 2, 'b': 3, 'c': 4, 'e': 6, 'f': 7}
Using collections.Chainmap
to Extend a Python Dictionary
Python provides the ChainMap
class that groups several dictionaries together to form a single view that can be updated when necessary. We import it from the collections
module in Python.
Use this method for faster chaining of elements. This is because
chainmap
only uses the dictionaries’ views; therefore, it does not involve copying data.
Additionally, it overwrites keys at any point; therefore, one could tell from the output what the data source is.
Implement it like this:
from collections import ChainMap
first_dict = {"a": 2, "b": 3, "c": 5}
second_dict = {"c": 4, "e": 6, "f": 7}
a_dictionary = ChainMap(first_dict, second_dict)