How to End Program in Python
-
End Python Program With the
quit()
Method -
End Python Program With the
exit()
Method -
End Python Program With the
sys.exit()
Method -
End Python Program With the
os.exit()
Method - Conclusion
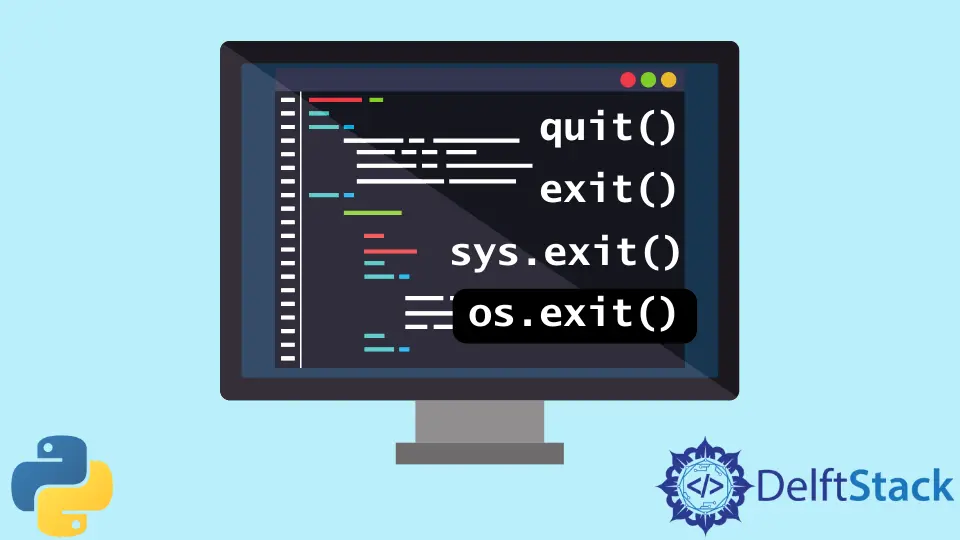
As in PHP, the die()
command terminates the running script. Similarly, the Python scripts can be terminated by using different built-in functions like quit()
, exit()
, sys.exit()
, and os.exit()
. Python executes the instructions in the top-to-down order, and as per the criteria, the loops which are defined in the code; however, when the Python Interpreter reaches the end of the file EOF
, it cannot read more instructions, so it ends the execution.
This article will introduce the methods to end the program in Python.
End Python Program With the quit()
Method
An easy and effective way to terminate a Python script using a built-in function is the quit()
method; this function can only be utilized in the Python Interpreter. When this command executes, it generates a SystemExit
exception in the OS. The complete example code is as follows:
for test in range(10):
if test == 5:
print(quit)
quit()
print(test)
Output:
0
1
2
3
4
Use quit() or Ctrl-Z plus Return to exit
End Python Program With the exit()
Method
Its functionality is the same as the quit()
method, but you don’t need to import Python libraries for this. The complete example code is as follows.
for test in range(10):
if test == 5:
print(exit)
exit()
print(test)
Output:
0
1
2
3
4
Use exit() or Ctrl-Z plus Return to exit
End Python Program With the sys.exit()
Method
This method is better than the quit()
and exit()
method. The syntax is:
sys.exit([arg])
The arg
is optional in the syntax. Mostly, it is an integer value, but the string value can also be passed. The zero argument value is considered to be the best case in successful termination. The complete example code is as follows.
import sys
weight = 70
if weight < 80:
sys.exit("weight less than 80")
else:
print("weight is not less than 80")
Output:
SystemExit: weight less than 80
End Python Program With the os.exit()
Method
This method is used to terminate the process with some special status like a child process in the script. A child process can be created using the os.fork()
method. The os.fork()
command will efficiently work on Linux; however, you have to utilize the Cygwin-built for Windows. Its reference is here.
The complete example code is as follows.
import os
parent_id = os.fork()
if parent_id > 0:
print("\nIn parent process")
info = os.waitpid(parent_id, 0)
if os.WIFEXITED(info[1]):
code = os.WEXITSTATUS(info[1])
print("Child's exit code:", code)
else:
print("child process")
print("Process ID:", os.getpid())
print("Test Code")
print("Child exiting..")
os._exit(os.EX_OK)
The os.wait()
method will return the child process ID with its termination status. We will get the exit code using the os._exit()
method.
Output:
AttributeError: module 'os' has no attribute 'fork'
Conclusion
The sys.exit()
method is preferred from all of the methods above. On the other hand, the os.exit()
command should be used for specific scenarios and instant termination.