Epsilon in Python
- What is Epsilon in Python
- Calculate Epsilon in Python
-
Use the
sys
Module to Find Epsilon in Python -
Use the
Numpy
Module to Find Epsilon in Python - Conclusion
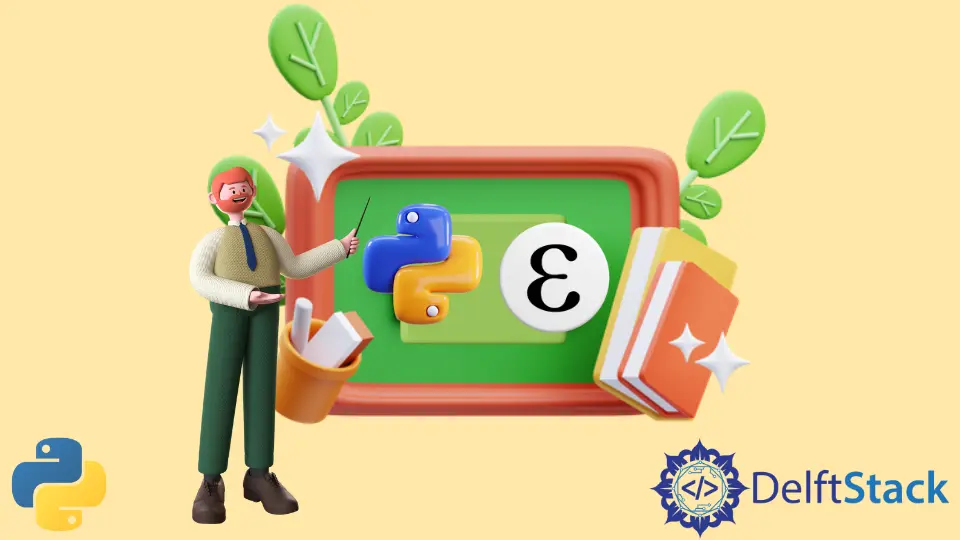
While solving integral calculus and mathematical domains, we often use epsilon as the smallest possible value.
In this article, we will discuss how we can calculate the value of epsilon in Python.
What is Epsilon in Python
Epsilon doesn’t have any special meaning in Python. It is not a keyword, and you can use the term epsilon to define variable names.
In Python, the semantic meaning of epsilon has been derived from its mathematical meaning. Thus, we can define epsilon as the smallest possible positive floating-point number.
In other words, epsilon is a number such that 1+epsilon
is the next floating-point number after 1 in Python. You can also define epsilon as the maximum possible error while representing a floating-point number in Python.
Having learned the meaning of epsilon, let us now calculate the value of epsilon in Python using different approaches.
Calculate Epsilon in Python
As epsilon is the smallest possible floating-point value such that 1+epsilon
is greater than 1, we will try to find the smallest value for epsilon such that 1+epsilon>1
. For this, we will initialize a variable epsilon with the value 1.0
.
After that, we will keep dividing epsilon by 2 until 1+epsilon
is greater than 1 in a while
loop. Once we find that the sum of 1 and the current value of epsilon is not greater than 1, we will exit the while
loop.
After that, we will multiply the current value of epsilon by 2 so that the sum of epsilon and 1 becomes greater than 1. In this way, we can find the value of epsilon in Python, as shown below.
epsilon = 1.0
while epsilon + 1 > 1:
epsilon = epsilon / 2
epsilon = epsilon * 2
print("The value of epsilon is:", epsilon)
Output:
The value of epsilon is: 2.220446049250313e-16
Instead of manually calculating the value of epsilon in Python, we can use built-in methods as discussed in the following sections.
Use the sys
Module to Find Epsilon in Python
Python provides us with the sys
module to perform various operations on the system using Python programs.
Using the sys
module, we can also find the value of epsilon in Python. For this, we will use the float_info
attribute of the sys
module.
The float_info
object in the sys
module contains definitions of various floating-point constants and limits. You can observe this in the following example.
import sys
print(sys.float_info)
Output:
sys.float_info(max=1.7976931348623157e+308, max_exp=1024, max_10_exp=308, min=2.2250738585072014e-308, min_exp=-1021, min_10_exp=-307, dig=15, mant_dig=53, epsilon=2.220446049250313e-16, radix=2, rounds=1)
In the output above, you can observe that the constant epsilon is also present in the float_info
object. Hence, we can obtain the value of epsilon using the sys.float_info
object in Python as follows.
import sys
epsilon = sys.float_info.epsilon
print("The value of epsilon is:", epsilon)
Output:
The value of epsilon is: 2.220446049250313e-16
Use the Numpy
Module to Find Epsilon in Python
Instead of using the sys
module, we can also use the numpy
module to find the value of epsilon in Python. For this, we will use the finfo()
method.
The finfo()
method is also used to obtain the floating-point constants and limits. It takes the literal float
as an input argument and returns an object of type numpy.finfo
.
We can use the eps
attribute of the object to find the value of epsilon in Python as follows.
import numpy
epsilon = numpy.finfo("float").eps
print("The value of epsilon is:", epsilon)
Output:
The value of epsilon is: 2.220446049250313e-16
Conclusion
This article has discussed various approaches to finding epsilon’s value in Python. For this, we have first calculated the value of epsilon by writing a program from scratch.
Later, we also used the sys
module and the numpy
module to find the value of epsilon in Python.
We hope you enjoyed reading this article. Stay tuned for more informative articles.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub