Ellipsis Object in Python
- Use Ellipsis as a Placeholder for Unwritten Code
-
Use Ellipsis as a Replacement for
pass
Keyword -
Use Ellipsis in Slicing Multidimensional
numpy
Array - Use Ellipsis for Type Hinting
- Conclusion
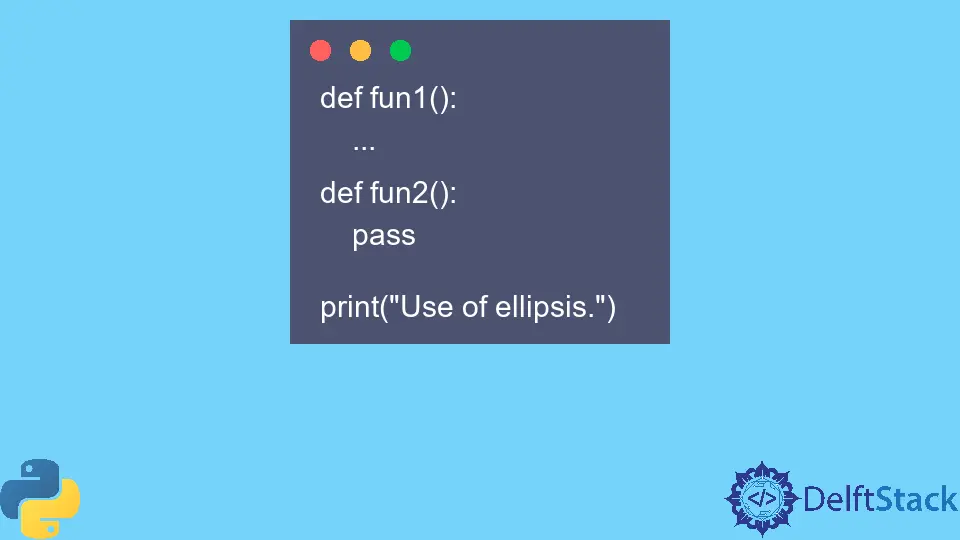
As a seasoned Python developer, you may have encountered the three dots ...
ellipsis object in Python. If you try to print this in the Python interpreter, it will display an ellipsis.
This tutorial will demonstrate the use of the ellipsis object in Python.
Use Ellipsis as a Placeholder for Unwritten Code
The ellipsis object can be used in different ways. It mainly acts as a placeholder for unwritten code.
Suppose we work in a team and need to define functions and classes. We define the functions and the class but are unsure of the code, so we place the three dots (ellipsis) in place.
This will prevent the Python interpreter from raising any exceptions.
See the code below.
def fun():
...
print("Use of ellipsis.")
Output:
Use of ellipsis.
The above example shows that we place the ellipsis object as a placeholder for the unwritten code in the fun()
method.
Use Ellipsis as a Replacement for pass
Keyword
The pass
keyword is used in Python when we want to add a line of code but do not wish to execute anything. Due to this, it is used as a placeholder for unwritten code.
As discussed, we can use the ellipsis object as a placeholder for unwritten code; thus, we can use it as a replacement for the pass
keyword.
We can see this in the following example.
def fun1():
...
def fun2():
pass
print("Use of ellipsis.")
Output:
Use of ellipsis.
The above example shows that the two functions have used pass
and ellipsis as a placeholder for unwritten code, demonstrating that we can replace pass
with the ellipsis
object.
Use Ellipsis in Slicing Multidimensional numpy
Array
Another significant use of this object comes in slicing.
Slicing refers to the technique of extracting portions of elements from an object based on their indexes. It is done in square brackets.
We can also perform slicing on multiple-dimensional arrays, but we need to be mindful of the total dimensions in the array. The ellipsis can be used as a placeholder in slice expressions to ignore the given dimension.
Consider the following code.
import numpy as np
arr = np.array([[7, 5, 2, 3], [1, 8, 9, 2], [3, 2, 1, 0]])
print(arr[:, 2])
print(arr[..., 2])
Output:
[2 9 1]
[2 9 1]
In the above example, notice how the same result is returned for different slicing expressions. The ellipsis as a placeholder in a slice expression is very helpful.
The expression arr[...,2]
can be transformed into arr[ : ,2]
for two dimensional-arrays, arr[ :, : , 2]
for three dimensional arrays and so on.
Use Ellipsis for Type Hinting
In Python 3.5 and above, another use for this singleton object was introduced.
Type hinting allows us to declare the types for variables, parameters, and return values. We can use ellipsis in type hinting to provide only part of the type.
We can use it like Tuple[int, ...]
, Callable[...,int]
, and more to help type hinting.
Conclusion
To conclude, we discussed the ellipsis object in Python and its uses. It is commonly used as a placeholder for unwritten code in functions and classes.
We can also use it as a placeholder in slicing to ignore multiple dimensions. Python 3.5 introduced a new way to use ellipsis in type hinting.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn