DNS Lookups in Python
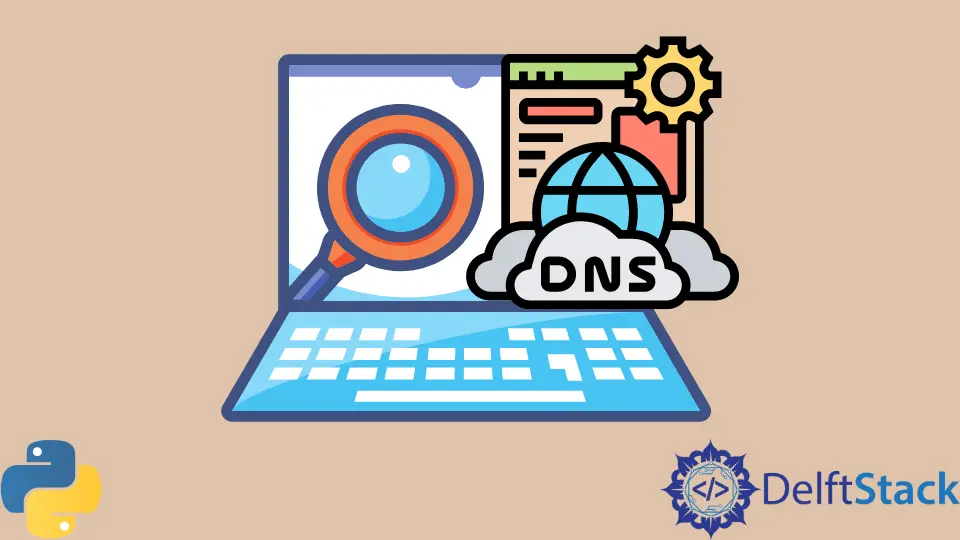
This article will discuss the concept of DNS and DNS lookup. After this, we discuss the method for the DNS lookups using Python.
What is DNS
The DNS (Domain Name System) converts a domain name into a public IP address. A DNS server converts the domain name of any website into the public IP of the host server of that website.
DNS Lookup
A DNS lookup is a process that initiates to find the public IP of any website whenever any user hits the website domain name or finds the domain name against the public IP.
The DNS lookup has two types:
-
Forward DNS Lookup
Forward DNS Lookup is also called Forward DNS. It is a process used to find the public IP of any domain by resolving the domain name using a DNS server.
-
Reverse DNS Lookup
Reverse DNS Lookup works as opposed to the forward DNS lookup. It is a process used to find the domain name against the public IP address.
Command for DNS Lookup
The nslookup
command finds the IP address or domain name system against a hostname. For example, the nslookup google.com
output is given below.
The nslookup google.com
command shows the domain name system and the public IP of the host google.com
.
DNS Lookups in Python
We can find the hostname or public IP of any domain in Python using the following code.
import socket
ip_addr = socket.gethostbyname("www.google.com")
print(ip_addr)
The Python library’s socket provides different functions and methods for developing network-based applications, including server-client programs. The gethostbyname
function is used to find the public IP address of any hostname. If you’re interested in learning more about processing data in Python, check out our article on Python Address Parser.
The gethostbyname
only returns the hostname’s IP address without considering the operating system’s configured rules. However, we can find the hostname information with all the configured rules of the operating system.
Consider the following code used to find the address information without ignoring the configured rules of the operating system.
import socket
print(socket.getaddrinfo("www.google.com", 80))
The getaddrinfo
function of the socket library in Python is used to get the information related to the hostname with considering the operating system configured rules.
The getaddrinfo
function returns a list of 5 elements family
, socktype
, proto
, and sockaddr
.
The family
, socktype
, and proto
are integers that can be used with the socket().canonname
, a string showing the host’s canonical name sockaddr
is a tuple with two elements address and port.