How to Peek A Deque in Python
- Overview of Python Deque Peek
-
Solution: Use Indexes to Peek the Front Element via
deque
Class - Conclusion
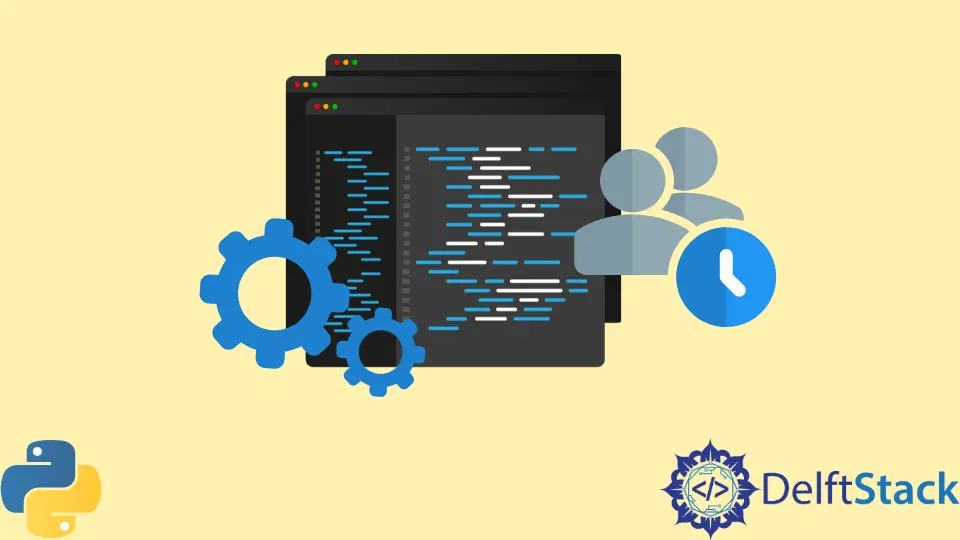
This article will demonstrate how to view/inspect the elements present at the front of a deque (double-ended queue) in Python without having to remove them from the deque.
Overview of Python Deque Peek
While using a deque in our program, we may want to check what is at the front of our deque. Based on what might be present at the front of the deque, we might want to take a different action.
While popping the element and checking for our necessary condition might seem the most obvious, it is not always the best choice. A typical scenario can be a constraint that does not allow altering the deque; other than that, it is also an unnecessary operation.
Consider the following code:
from collections import deque
data = deque([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
In this snippet of code, we are first importing the deque
data structure from the collections
module. After which, a deque
is initialized, with elements ranging from 1
to 10
.
Now, it is required that we access the deque
’s front without popping elements of a deque
from any direction. How can we do that? Let’s learn it below.
Solution: Use Indexes to Peek the Front Element via deque
Class
Before discussing the solution, we must learn how indexing works in Python and how we can use indexes to our advantage to access the front member of the deque
without popping any element.
Indexes in Python
The Python index()
method refers to specific elements within an iterable depending on their position. Therefore, we can also say that we can directly access the desired elements within an iterable and perform various actions based on your requirements.
Like almost all other programming languages, objects in Python are zero-indexed
, meaning the position count begins at zero. Numerous additional programming languages follow the same structure.
Therefore, if there are five items in a list, the initial element (the element on the far left) occupies the zero
position, followed by the elements in the first, second, third, and fourth positions.
The index()
method reveals the index of a specific item within the list if we call it on a list with the item’s name as the argument.
Consider the following code:
cars = ["Suzuki", "Honda", "Chevrolet", "Ford", "Tesla"]
print("Index of Suzuki: ", cars.index("Suzuki"))
print("Index of Tesla: ", cars.index("Tesla"))
Output:
Index of Suzuki: 0
Index of Tesla: 4
In this code, we have a list called cars
containing several car manufacturers, such as Suzuki, Honda, Chevrolet, Ford, and Tesla
. We are using the .index()
method to find the position of specific items within the list.
When we execute the code, it returns the indices of Suzuki
and Tesla
in the cars
list. The output tells us that Suzuki
is located at index 0
, while Tesla
is at index 4
within the list, and this means that Suzuki
is the first element in the list, and Tesla
is the fifth element when counting from the beginning.
The Python programming language follows a zero-based indexing system, with the first element at index 0
, the second at index 1
, and so on. Therefore, these print statements help us determine the precise positions of these car manufacturers within the cars
list.
Python Index Operators
Square brackets []
represent the index operator in Python. However, the syntax requires that you place a number within the brackets.
Syntax:
IterableObject[index]
Negative Indexes in Python
Until this point, we have always utilized a positive integer within our index operator (the square brackets, []
) in the previous examples; negative indexes also exist.
If we are interested in the final few members of a list, or if we want to index the list from the other end, we can frequently utilize negative integers.
Negative indexing describes this method of indexing from the other end. Consider the following code:
cars = ["Suzuki", "Honda", "Chevrolet", "Ford", "Tesla"]
print(cars[-1])
print(cars[-2])
Output:
Tesla
Ford
In this code, we have a list named cars
that contains the names of various car manufacturers, including Suzuki, Honda, Chevrolet, Ford, and Tesla
. We are using negative indexing to access elements from the end of the list, and when we execute the code, it prints out the elements at positions -1
and -2
in the cars
list.
The output reveals that when we use negative indexing, -1
corresponds to the last element in the list, which in this case is Tesla
. Similarly, -2
corresponds to the second-to-last element in the list, which is Ford
.
Negative indexing is a useful feature in Python, allowing us to access elements from the end of a list without needing to know its length. So, with these print statements, we effectively retrieve the last and second-to-last elements in the cars
list.
Now that we know how negative indexing works, the solution to our problem becomes crystal clear. Consider the following code:
from collections import deque
data = deque([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
print("First Element: " + str(data[0]))
print("Second Element: " + str(data[-1]))
In this code, we are using Python’s collections
module to work with a data structure called a deque, which is short for a double-ended queue. We create a deque called data
and initialize it with a sequence of integers from 1
to 10
.
Deques are versatile data structures that allow efficient access to both the front and back of the queue.
The code then proceeds to print the first and second elements of the data
deque. We use indexing to access these elements, with data[0]
representing the first element and data[-1]
representing the last element.
Output:
First Element: 1
Second Element: 10
This output demonstrates that we have successfully accessed the first element, which is 1
, and the last element, which is 10
, in the data
deque using the deque’s index-based access. This capability is valuable when you need to inspect elements at the front and back of a deque without altering its contents.
You can peek the front element using deque[0]
and peek the last using deque[-1]
. It works without elements popping from the left or right and also appears efficient.
It’s worth noting that deques support both positive and negative indexing.
Conclusion
In conclusion, this article has explored how to efficiently access elements at the front of a deque in Python without the need to remove them from the deque. We discussed the importance of this operation in scenarios where altering the deque is restricted or simply unnecessary.
Through the use of indexing, we showcased how to achieve this by retrieving the first and last elements of both a regular list and a deque, providing valuable insights for practical coding. By mastering this technique, you can confidently inspect the front and back of a deque, making informed decisions within your Python programs without disturbing the structure of the data.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn