Python Decorator Order
- Python Decorator Order
- Prerequisites for Decorator Order in Python
-
Syntax of
@decorator
in Python - How Decorator Order is Determined in Python
-
Decorator
@decorator
Order in Python - Two Different Kinds of Decorators in Python
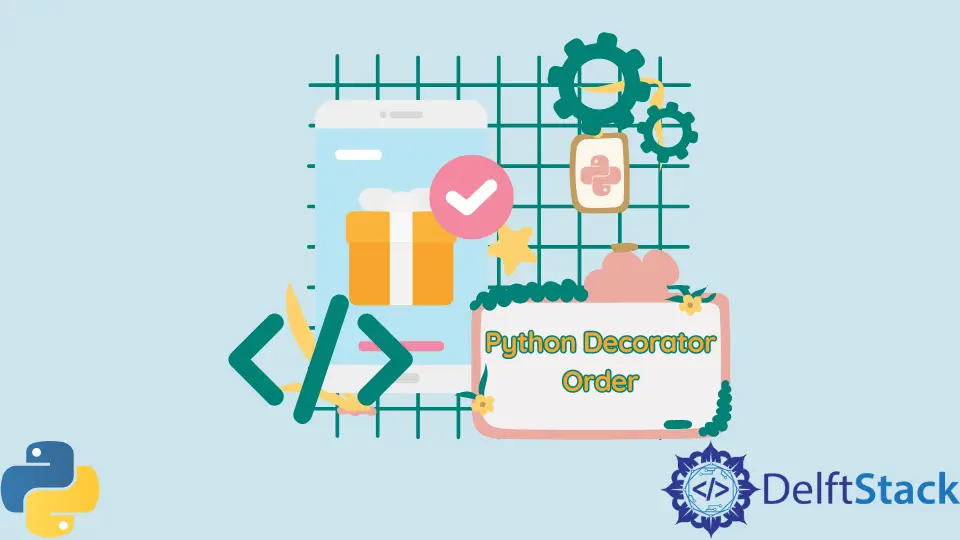
In this Python article, we will look at what decorators are, how they are used, and how we can utilize them to build code. We will see how a decorator is a strong tool that can be used to add functionality to our applications and can be found in the Python programming language.
Python Decorator Order
In Python, a decorator is a special function that modifies another function’s behavior. The decorator is a design pattern that adds new functionality to an existing object without changing its structure and is usually called before defining a function or a class.
Decorators in Python are a powerful tool for modifying functions and classes. A decorator is a function that takes another function as an argument and returns a new function that wraps the original function.
It can also be used to modify the behavior of a function without changing the code of the function itself. This is useful for adding features to existing functions or changing a function’s behavior in a specific context.
Decorators are often used to implement features such as:
- Caching
- Logging
- Access Control
Prerequisites for Decorator Order in Python
We must have a fundamental understanding of Python before we can learn about decorators.
We must come to terms with the idea that everything in Python is considered objects, even classes. The names we provide to these things are only identifiers connected to them.
There are no exceptions here; functions are also considered objects (with attributes). Multiple distinct names have the potential to be tied to a single function object.
So, we must have basic knowledge of the following:
- Python Programming Language
- Functions
- Classes
Then, we can easily work with decorator orders in Python.
Syntax of @decorator
in Python
The next step is to look into the syntax of Python’s decorators and inquire: how does Python determine the order in which to interpret decorators?
Example Code:
@mydecorator
def my_function():
pass
So, this is the syntax of a decorator
in Python. We will be using decorators with functions and classes with the same syntax.
How Decorator Order is Determined in Python
When numerous decorators are applied to a function or class, the decorators are used in the order they were created; this might lead to unexpected behavior. We must keep this fact in mind since the order in which decorators are applied might affect how a function or class behaves.
It is essential to remember that the order in which decorators are applied might affect the operation of the class or function. When several decorators are put on a component, the decorators are put in the order they were declared.
Decorator @decorator
Order in Python
In Python, decorators are typically implemented as functions that take a function as an argument and return a modified function. For example, we can consider the following decorator.
Follow the steps below to write the code:
-
Define the function using the
def
keyword. -
Define another function inside the function using the
def
keyword. -
Print any statement or add some functionality in the function.
-
Use the
return
statement for both functions. -
Define the decorator.
-
Follow the steps earlier mentioned in the syntax of the decorator.
-
Define another function for decorator.
-
Add some functionalities to the function. See the code below for a better understanding.
-
Print the decorator function and provide a value for the parameter
x
.
Example Code:
def decorator(func):
def wrapper(x):
print("Before calling ", func.__name__)
result = func(x)
print("After calling ", func.__name__)
return result
return wrapper
@decorator
def foo(x):
return x + 1
print(foo(2))
Here we are simply printing to see the output of our entire code.
The output of the code:
Before calling foo
After calling foo
3
We can see that the first statement is printed: (Before calling foo
). The second statement in the code (After calling foo
) is printed second.
And the decorator
part is printed at the end, which is x+1 because we passed the value 2 for the decorator’s parameter value. And we got 3
as a result.
Now, we will look into another code to better understand the decorators.
So, we have already gone through the steps of defining functions and decorators. We will again follow the same steps according to our requirements.
In this code, suppose we want the decorator
part to run earlier than the second statement of the nested function. So we will use the following code.
Example Code:
def my_decorator(func):
def wrapper():
print("Before the function is called.")
func()
print("After the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello, Abid!")
say_hello()
The output of the code:
Before the function is called.
Hello, Abid!
After the function is called.
Here we can see that Before the function is called.
is printed first. Hello, Abid!
the decorator part is printed second, and the After the function is called.
is printed at the end.
Two Different Kinds of Decorators in Python
Now we will see how two different decorator functions can be applied to the num()
method. The inner decorator will work first, followed by the outer decorator after a short break.
Example Code:
# code for testing decorator chaining
def decorator1(function):
def inner():
a = function()
return a + a
return inner
def decorator(function):
def inner():
a = function()
return 4 * a
return inner
@decorator1
@decorator
def num():
return 10
print(num())
The output of the code:
80
So, in the example above, the inner decorator
worked first, and the outer worked after it. And therefore, we get the output of code 80
.
The order in which decorators are applied can be important. In general, we should apply decorators to functions and classes in the order in which they will be called.
However, decorators used in classes should always be applied before decorators are applied to functions.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn