How to Create requirements.txt in Python
- Method 1: Using pip freeze
- Method 2: Manually Creating requirements.txt
- Method 3: Using pipreqs
- Conclusion
- FAQ
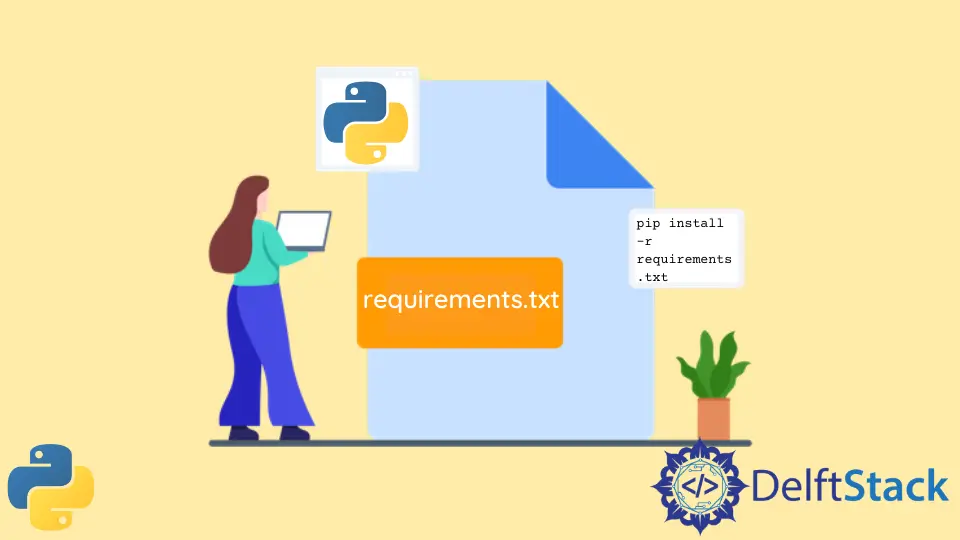
Creating a requirements.txt
file is a crucial step for any Python developer looking to manage project dependencies effectively. This simple text file lists all the packages your Python project requires, making it easier to replicate the environment on different machines or share your project with others.
In this article, we’ll explore how to create a requirements.txt
file using various methods. Whether you’re working on a collaborative project or deploying your application, understanding how to manage dependencies is essential. Let’s dive in and learn how to create your own requirements.txt
in Python!
Method 1: Using pip freeze
One of the most straightforward ways to create a requirements.txt
file is by using the pip freeze
command. This command outputs a list of all installed packages in your current Python environment, along with their respective versions. Here’s how to do it:
First, navigate to your project directory in the terminal. Then, run the following command:
pip freeze > requirements.txt
Output:
package1==version1
package2==version2
This command will generate a requirements.txt
file in the current directory. Each line of the file will contain the name of a package followed by its version, formatted as package==version
. This approach is particularly useful if you’ve already installed the necessary packages for your project.
Once the requirements.txt
file is created, you can easily share it with others or use it to set up the same environment elsewhere. To install the packages listed in your requirements.txt
, simply use:
pip install -r requirements.txt
This command reads the requirements.txt
file and installs all the specified packages, ensuring that anyone who works on your project has the same dependencies.
Method 2: Manually Creating requirements.txt
If you prefer to have more control over the contents of your requirements.txt
file, you can create it manually. This method is beneficial when you want to specify only certain packages or versions that are critical for your project. Here’s how to do it:
- Open a text editor of your choice.
- Create a new file and name it
requirements.txt
. - List the packages and their versions in the following format:
package1==version1
package2==version2
For example, your requirements.txt
might look like this:
Flask==2.0.1
requests==2.25.1
numpy==1.21.0
Output:
Flask==2.0.1
requests==2.25.1
numpy==1.21.0
By manually creating the file, you can tailor it to include only the packages that your project truly needs. This helps in keeping your environment clean and avoids unnecessary installations. After saving the file, you can use the same pip install -r requirements.txt
command to install the packages.
This method is particularly useful for smaller projects or when you’re starting a new application and want to define your dependencies from scratch.
Method 3: Using pipreqs
If you have a large project and want to generate a requirements.txt
file based on the imports in your code, pipreqs
is an excellent tool to consider. This command-line utility scans your Python files to identify which packages are being used and generates a requirements.txt
file accordingly. Here’s how to use it:
First, you need to install pipreqs
if you haven’t already:
pip install pipreqs
Next, navigate to your project directory and run:
pipreqs . --force
Output:
Flask==2.0.1
requests==2.25.1
numpy==1.21.0
The --force
option overwrites any existing requirements.txt
file. After executing this command, pipreqs
will analyze your project files and create or update the requirements.txt
file with the necessary packages and their versions.
This method is particularly beneficial for larger projects where manually tracking dependencies can become cumbersome. It ensures that all used packages are included, helping to avoid runtime errors due to missing dependencies.
Conclusion
Creating a requirements.txt
file is an essential practice for any Python developer. Whether you choose to use pip freeze
, manually create the file, or utilize tools like pipreqs
, understanding how to manage your project dependencies is crucial for maintaining a smooth workflow. By following the methods outlined in this article, you can ensure that your Python projects are well-organized and easily shareable with others. Happy coding!
FAQ
-
What is a requirements.txt file?
A requirements.txt file lists all the dependencies needed for a Python project, including package names and versions. -
How do I install packages from requirements.txt?
You can install packages listed in the requirements.txt file by running the commandpip install -r requirements.txt
in your terminal. -
Can I create requirements.txt for a virtual environment?
Yes, you can create a requirements.txt file for any Python environment, including virtual environments. Just activate the environment and use the same methods mentioned. -
What happens if I don’t specify package versions in requirements.txt?
If you don’t specify versions, pip will install the latest versions of the packages, which may lead to compatibility issues. -
Is it necessary to use requirements.txt for every Python project?
While it’s not mandatory, using a requirements.txt file is highly recommended for managing dependencies effectively, especially in collaborative projects.