How to Make Compound Interest Calculator in Python
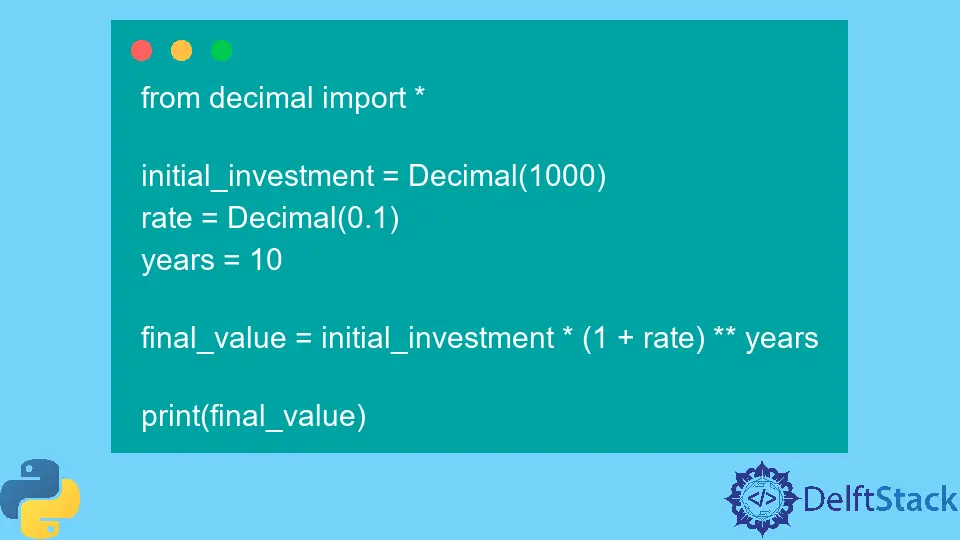
Python is an excellent language for financial analysis, one of the things you can do with Python is calculated compound interest. This is when interest is paid not only on the principal (the original amount of money) but also on the possible interest that has accrued.
In this article, we’ll discuss the Python compound interest function.
Python Compound Interest Function
The Python compound interest function is a mathematical function that calculates the interest that is accrued on a loan or investment over time. This function considers the principal amount, the interest rate, and the number of compounding periods.
First, import the math module to calculate compound interest in Python. This module has a function called pow()
, which calculates exponents, and once you import the math module, you can calculate compound interest using the pow()
function.
You can use the compound interest formula, A = P(1 + r/n)^nt
. In this formula, A
is the total amount of money after n
years, P
is the principal, n
is used for the number of times interest is compounded per year, and r
is the interest rate.
To use this formula in Python, you first need to calculate the value of (1 + r/n)
. This can be done by utilizing the pow()
function. Then, you would need to calculate the value of P(1 + r/n)^nt
, which can be done by first calculating P * (1 + r/n)
and then raising that value to its power.
Once you calculate the total amount of money after n
years, you can use the print()
function to print it out.
Implement Compound Interest Calculator in Python
Use the pow()
Function to Implement Compound Interest Calculator in Python
One way to implement a compound interest calculator in Python is to use the built-in function pow()
.
This function takes two arguments.
- The base value.
- And the exponent.
The base value is the initial investment amount, and the exponent is the number of compounding periods.
For example, if you have an initial investment of $1000 and want to compound it at a rate of 10% per year for 10 years, you would use the following calculation.
Code Example:
pow(1000, 1.0 + 0.1 * 10)
Output:
1000000.0
Use the decimal
Module to Implement Compound Interest Calculator in Python
The decimal
module allows you to define decimal values with a specified number of decimal places.
For example, if you want to calculate compound interest with an initial investment of $1000 and a rate of 10% per year for 10 years, use the following code.
Code Example:
from decimal import *
initial_investment = Decimal(1000)
rate = Decimal(0.1)
years = 10
final_value = initial_investment * (1 + rate) ** years
print(final_value)
Output:
2593.742460100000130892390868
You should know some points when using a Python compound interest calculator to get the correct answer.
- Ensure the rate is entered as a decimal, not a percentage.
- Second, remember to include the n (number of times interest is compounded per year) in the calculation.
- Finally, don’t forget to subtract 1 from the total number of years to get the correct answer.
Code Example:
P = int(input("Enter starting principle please. "))
n = int(input("Enter number of compounding periods per year. "))
r = float(input("Enter annual interest rate. e.g. 15 for 15% "))
y = int(input("Enter the amount of years. "))
FV = P * (((1 + ((r / 100.0) / n)) ** (n * y)))
print("The final amount after", y, "years is", FV)
Output:
Enter starting principle please. 1000
Enter number of compounding periods per year. 1
Enter annual interest rate. e.g. 15 for 15% 2
Enter the amount of years. 1
The final amount after 1 years is 1020.0
Conclusion
The Python compound interest formula is a powerful tool that can be used to estimate the future value of a loan or investment. The Python compound interest function is not the easiest to work with, and this article will help you get it right with Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn