How to Parse Command Line Arguments Using Python
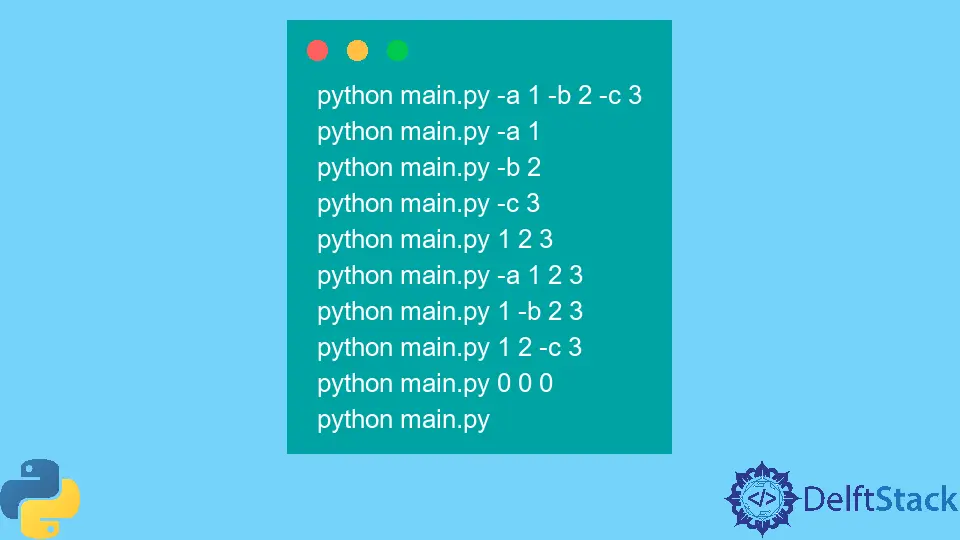
While running Python programs from command line or shell, we can pass values in the form of strings, technically, arguments or options, to the program. The program can access these values and carry out its execution and even use these values for computation.
This article will talk about how we can parse these command-line arguments using Python and use them.
Parse Command Line Arguments Using the optparse
Module
The optparse
module is a flexible and convenient Python package to parse command-line options. It comes built-in with the standard Python. It uses a declarative methodology to parse command-line options. To use this package, we first have to create an object of the class OptionParser
and then add details about the possible options or arguments with the help of the add_option()
method. The add_option()
method accepts arguments such as option
(the option name), type
(the data type of the option), help
(the help text for the option), and default
(the default value for the option, if not specified in the command). To get the options and their values from the command line command in the form of a dictionary, we can use the parse_args()
method.
Let us understand the use of this library better with the help of an example. We will implement a simple Python program that will check for three options: -a
, -b
, and -c
. Here, -a
and -b
represent the operands, +
is the operator, and -c
is essentially the target sum. Furthermore, the code will check for these values, assign them the 0
value if no value is found in the command line command, and lastly, check if a + b
is equal to c
or not. Moreover, it will also print the results in a well-formatted string.
import optparse
parser = optparse.OptionParser()
parser.add_option("-a", help="First Number", default=0)
parser.add_option("-b", help="Second Number", default=0)
parser.add_option("-c", help="Sum", default=0)
options, args = parser.parse_args()
a = int(options.a)
b = int(options.b)
c = int(options.c)
s = a + b
print(f"{a} + {b} = {s} and {s} {'==' if s == c else '!='} {c}")
Here is a brief explanation of the above code. First, it creates an OptionParser()
class object and adds information about the three target options; -a
, -b
, and -c
. Then it traverses the command line or shell command to check for the required options. Lastly, it stores all the values in separate variables, computes the sum or a + b
, and compares it to the target sum or c
.
Let us test this program for the following commands.
python main.py -a 1 -b 2 -c 3
python main.py -a 1
python main.py -b 2
python main.py -c 3
python main.py 1 2 3
python main.py -a 1 2 3
python main.py 1 -b 2 3
python main.py 1 2 -c 3
python main.py 0 0 0
python main.py
Output:
1 + 2 = 3 and 3 == 3
1 + 0 = 1 and 1 != 0
0 + 2 = 2 and 2 != 0
0 + 0 = 0 and 0 != 3
0 + 0 = 0 and 0 == 0
1 + 0 = 1 and 1 != 0
0 + 2 = 2 and 2 != 0
0 + 0 = 0 and 0 != 3
0 + 0 = 0 and 0 == 0
0 + 0 = 0 and 0 == 0
From the outputs, we can infer that the default values are considered when no keyword options or arguments are provided to the command line or shell command. Refer to the test commands except the first one (python main.py -a 1 -b 2 -c 3
) for better analysis.