How to Clear Memory in Python
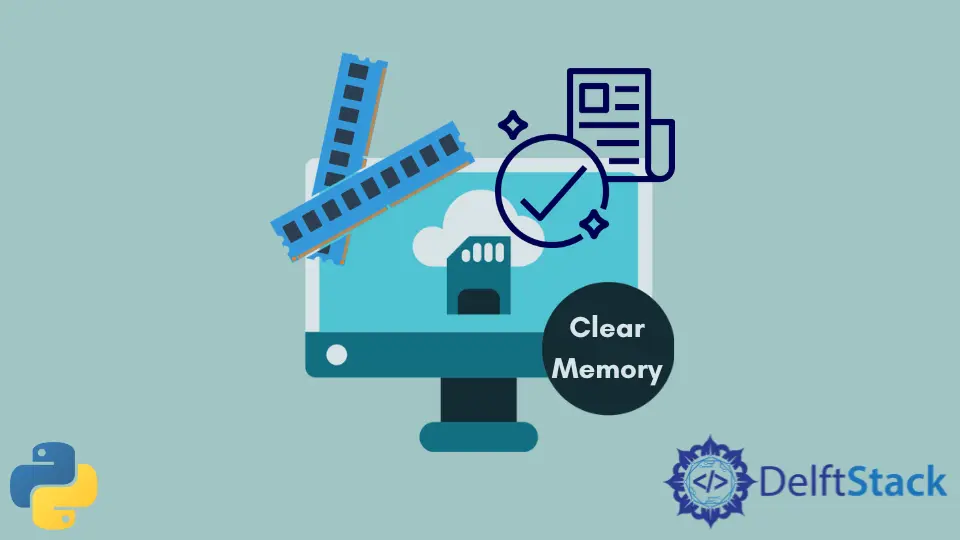
This tutorial will look into the methods to free or clear memory in Python during the program execution. When a program has to deal with large files, process a large amount of data, or keep the data in the memory. In these types of scenarios, the program can often run out of memory.
To prevent the program from running out of memory, we have to free or clear the memory by clearing the variable or data, which is no more needed in the program. We can clear the memory in Python using the following methods.
Clear Memory in Python Using the gc.collect()
Method
The gc.collect(generation=2)
method is used to clear or release the unreferenced memory in Python. The unreferenced memory is the memory that is inaccessible and can not be used. The optional argument generation
is an integer whose value ranges from 0
to 2
. It specifies the generation of the objects to collect using the gc.collect()
method.
In Python, the short-lived objects are stored in generation 0
and objects with a longer lifetime are stored in generation 1
or 2
. The list maintained by the garbage collector is cleared whenever the gc.collect()
with default generation
value equal to 2
is called.
The gc.collect()
method can help decrease memory usage and clear the unreferenced memory during the program execution. It can prevent the program from running out of memory and crashing by clearing the memory’s inaccessible data.
Clear Memory in Python Using the del
Statement
Along with the gc.collect()
method, the del
statement can be quite useful to clear memory during Python’s program execution. The del
statement is used to delete the variable in Python. We can first delete the variable like some large list, or array, etc., about which we are sure that are no more required by the program.
The below example code demonstrates how to use the del
statement to delete the variable.
import numpy as np
a = np.array([1, 2, 3])
del a
Suppose we try to use or access the variable after deleting it. In that case, the program will return the NameError
exception as the variable we are trying to access no more exists in the variable namespace.
Example code:
import numpy as np
a = np.array([1, 2, 3])
del a
print(a)
Output:
NameError: name 'a' is not defined
The del
statement removes the variable from the namespace, but it does not necessarily clear it from memory. Therefore, after deleting the variable using the del
statement, we can use the gc.collect()
method to clear the variable from memory.
The below example code demonstrates how to use the del
statement with the gc.collect()
method to clear the memory in Python.
import numpy as np
import gc
a = np.array([1, 2, 3])
del a
gc.collect()