How to Clamp Numbers Within a Range Using Python
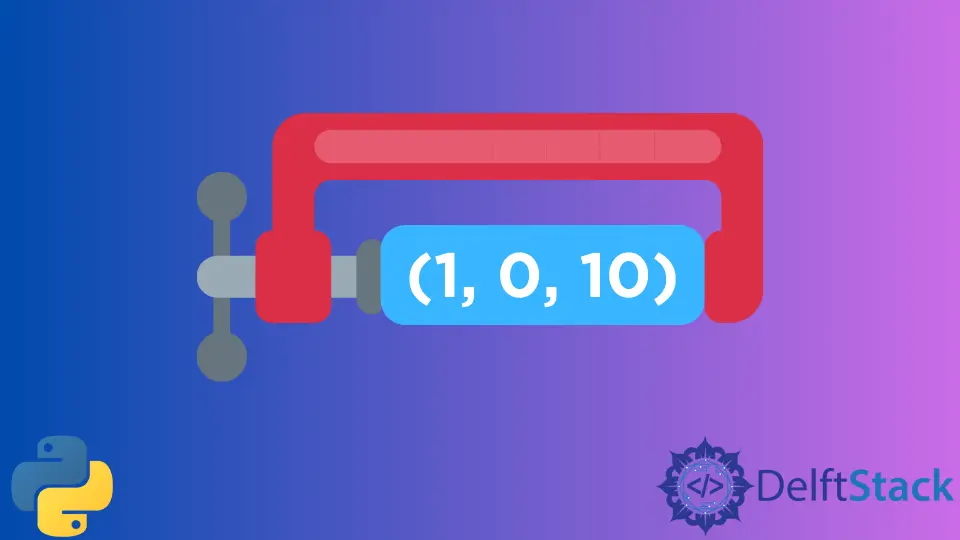
Clamping refers to limiting a value within a range. When a value exceeds a range, it is changed to the largest possible value, and if the value succeeds a range, it is changed to the smallest possible value.
In this article, we will learn how to clamp numbers within a range using Python.
Clamp Numbers Within a Range Using Python
The following Python code depicts how to clamp numbers within a range.
def clamp(n, smallest, largest):
return max(smallest, min(n, largest))
print(clamp(1.000001, 0, 1))
print(clamp(34.2, 0, 34))
print(clamp(45, 0, 10))
print(clamp(1, 0, 1))
print(clamp(-100, 0, 100))
Output:
1
34
10
1
0
The stub function above returns the input value within the range. Take note of how the min()
and the max()
functions have been used for the implementation.
A more creative way to write the above stub function is using a list and sorting operation. The following Python code depicts this.
def clamp(n, smallest, largest):
return sorted([smallest, n, largest])[1]
print(clamp(1.000001, 0, 1))
print(clamp(34.2, 0, 34))
print(clamp(45, 0, 10))
print(clamp(1, 0, 1))
print(clamp(-101, 0, 100))
Output:
1
34
10
1
0
The perfect or the clamped value will always lie in the middle of the list or at index 1
. In case the input value is greater than the largest possible value, the largest possible value will get in the middle of the list.
If the input value is smaller than the smallest possible value, the smallest possible value will lie in the middle of the list. And, if the input value is within the range, it will lie in the middle of the list.