How to Get Cartesian Product in Python
-
Get Cartesian Product in Python Using the
itertools
Module - Get Cartesian Product in Python Using the List Comprehension Method
- Get Cartesian Product in Python Using the Iterative Method
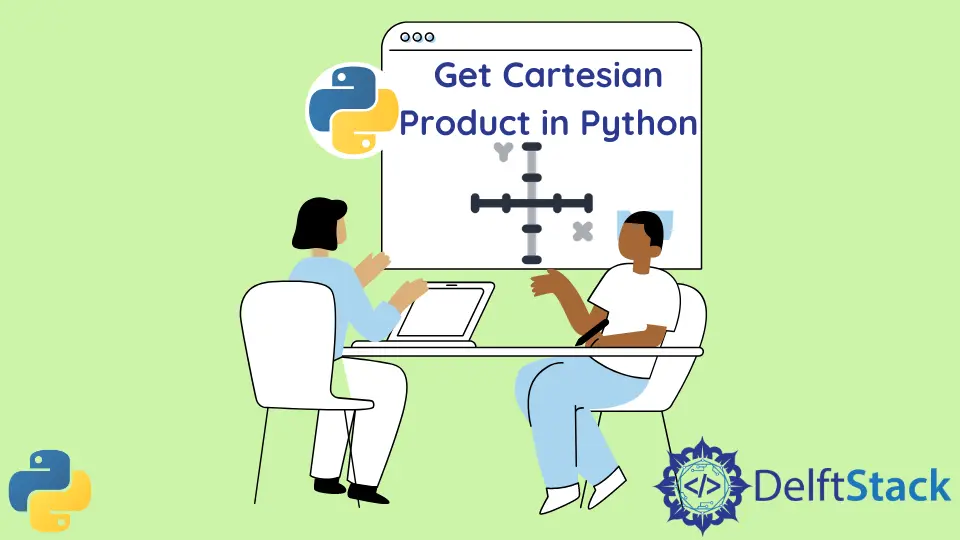
In this tutorial, we will learn different methods to get the cartesian product of a list in Python. The cartesian product of two sets will be a set of all possible ordered pairs with the first element of each ordered pair from the first set and the second element from the second set.
We can find the cartesian product of sets saved as a 2D list using the following methods in Python.
Get Cartesian Product in Python Using the itertools
Module
The product(*iterables, repeat=1)
method of the itertools
module takes iterables
as input and returns their cartesian product as output. The cartesian product order will be the order of each set/list in the provided argument iterables
. The optional keyword argument repeat
represents the number of times we want to repeat the product with the input iterables
. And the *
is used to unpack the argument iterables
.
The below example code demonstrates how to get the cartesian product in Python using the itertools.product()
method.
from itertools import product
mylists = [["a", "b"], [1, 2]]
for elem in product(*mylists):
print(elem)
Output:
('a', 1)
('a', 2)
('b', 1)
('b', 2)
Get Cartesian Product in Python Using the List Comprehension Method
We can use the list comprehension method to get the cartesian product of the lists if the total number of the lists is known.
If we know the number of lists or the number of lists is fixed, we will have to iterate through each list element using the for
loop to get their cartesian product.
The below example code demonstrates how to use the list comprehension method in Python to get the cartesian product of the list.
mylists = [["a", "b"], [1, 2]]
crt_prd = [(x, y) for x in mylists[0] for y in mylists[1]]
print(crt_prd)
Output:
[('a', 1), ('a', 2), ('b', 1), ('b', 2)]
Get Cartesian Product in Python Using the Iterative Method
Another way to get the cartesian product in Python is to use the iterative for
loop approach. It is a better approach than the list comprehension we used above, as in this method, we do not have to worry about the number of lists or sets for the cartesian product.
Therefore instead of accessing each element of each list, we use an iterative approach. The below example code demonstrates how to find the cartesian product in Python using the iterative method.
def get_cart_prd(pools):
result = [[]]
for pool in pools:
result = [x + [y] for x in result for y in pool]
return result
mylists = [["a", "b"], [1, 2, 3]]
print(get_cart_prd(mylists))
Output:
[['a', 1], ['a', 2], ['a', 3], ['b', 1], ['b', 2], ['b', 3]]