How to Encode a String as Base64 Using Python
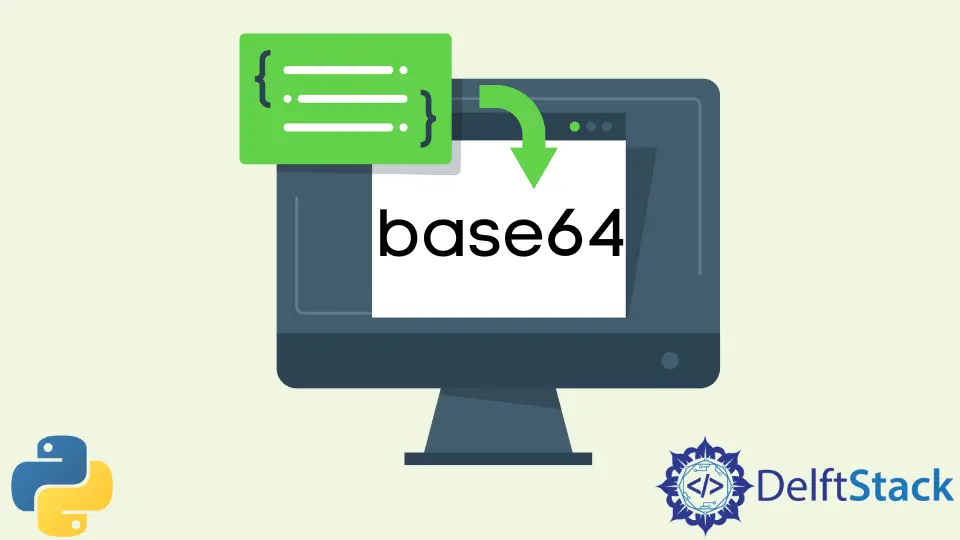
The Base64
is an encoding scheme that converts a sequence of 8-bit bytes
binary data to an ASCII or American Standard Code for Information Interchange string format by translating the binary data.
This conversion is generally used for transferring data without any modification.
All the programming languages contain utilities to convert data from one format to another. In this article, we will learn how to encode a string as base64 with the help of the Python programming language.
Encode a String as base64
Using Python
To perform encoding to base64
, we will use a Python-based module, base64
. This module is a part of the Python standard library.
This module has a b64encode(s, altchars = None)
method that accepts a bytes stream of data s
and converts it to a base64 encoded string.
Yet another parameter, altchars
, specifies an alternative alphabet for characters +
and /
. It is needed to ensure that the conversion of URLs and file paths to base64 is safe and sound.
This library has yet another method, b64decode(s, altchars = None, validate = False)
, that accepts a base64 encoded stream of data s
, an optional ASCII or bytes-like string representing alternative characters for +
and /
characters altchars
.
This method also has a parameter, validate
, a flag for performing validation over the offered string. By default, the method will ignore all the characters that do not fit inside the regular base-64 alphabet or the alternative alphabet string.
If set to True
, it will raise a binascii.Error
exception. Now that we are through with some theory let us look at relevant examples.
import base64
s1 = b"Python"
s2 = b"https://www.instagram.com"
s3 = b"C:\Program Files\User"
# Encoding
e1 = base64.b64encode(s1)
e2 = base64.b64encode(s2)
e3 = base64.b64encode(s3)
# Decoding
d1 = base64.b64decode(e1)
d2 = base64.b64decode(e2)
d3 = base64.b64decode(e3)
print("S1:", s1)
print("S2:", s2)
print("S3:", s3)
print("S1 Encoded to base64:", e1)
print("S2 Encoded to base64:", e2)
print("S3 Encoded to base64:", e3)
print("E1 Decoded:", d1)
print("E2 Decoded:", d2)
print("E3 Decoded:", d3)
Output:
S1: b'Python'
S2: b'https://www.instagram.com'
S3: b'C:\\Program Files\\User'
S1 Encoded to base64: b'UHl0aG9u'
S2 Encoded to base64: b'aHR0cHM6Ly93d3cuaW5zdGFncmFtLmNvbQ=='
S3 Encoded to base64: b'QzpcUHJvZ3JhbSBGaWxlc1xVc2Vy'
E1 Decoded: b'Python'
E2 Decoded: b'https://www.instagram.com'
E3 Decoded: b'C:\\Program Files\\User'
The Python code snippet above initializes three strings: a usual string, a URL, and a Microsoft Windows file path.
Next, it encodes all the three strings to base64 and further decodes base64 strings to bytes. The b
prefix in front of strings converts them into bytes.
Lastly, all the information is printed on the terminal. To learn more about the base64
library, refer to the official documentation here.