How to Check Argument of Argparse in Python
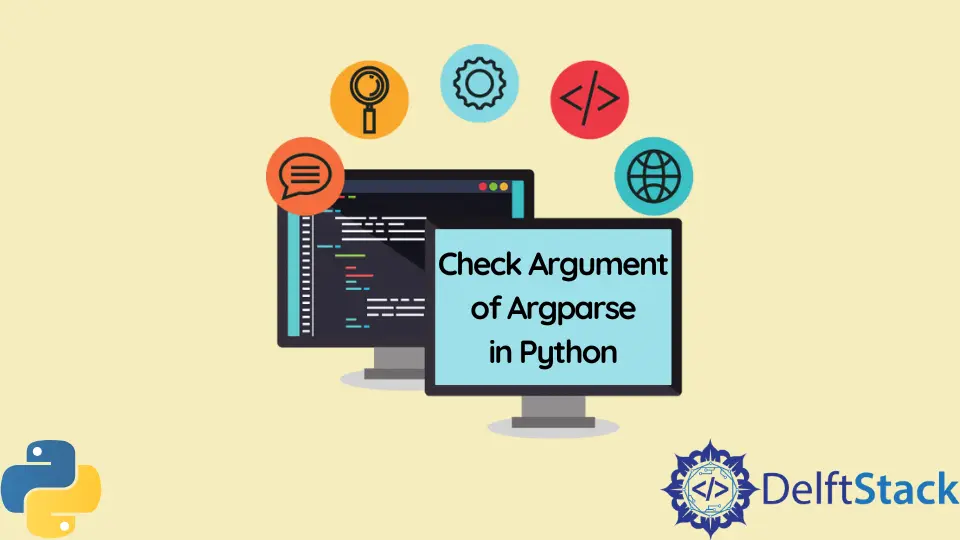
This tutorial will discuss the use of argparse
, and we will check if an argument exists in argparse
using a conditional statement and the argument’s name in Python.
Check Argument of argparse
in Python
The argparse
library of Python is used in the command line to write user-friendly interfaces. For example, we can run a script using the script name and provide the arguments required to run the script.
We can add arguments after the name of the script file, and the argparse
library will convert these arguments to objects which can be used inside the script to perform the required task. We can also attach help, usage, and error messages with each argument to help the user.
For example, if a user inputs an invalid argument, the argparse
library will show an error and how the user should enter the argument. For example, let’s discuss a simple example of the argparse
library.
See the code below.
import argparse
My_parser = argparse.ArgumentParser()
My_parser.parse_args()
Output:
PS C:\Users\ammar> python test.py -h
usage: test.py [-h]
optional arguments:
-h, --help show this help message and exit
To run the above script, we have to write python test.py -h
in the cmd
(command prompt) and ensure Python is added to the system path
or environmental variables on Windows. We can use a full path to the python.exe
file if it’s not added.
We also have to ensure the command prompt’s current directory is set to the Python file’s directory; if it is not, we have to provide the full path to the Python file.
For example, let’s consider we have to add Python to the system path
, and we are also not in the current directory of the Python file. We must change the first line in the above output to the below line.
& C:/Users/ammar/python.exe "c:/Users/ammar/test.py" -h
The above line is tested in Windows PowerShell, and for cmd
, we have to remove the &
at the start of the line. In the above output, we can observe that only one argument is used for help.
We can use the add_argument()
function to add arguments in the argument parser. We can set two types of argument: one is a positional argument, and the other is an optional one.
We must use the single -
or double hyphen --
before the argument to make it optional. We can also add help for an argument using the help
argument and setting its value to a string inside the add_argument()
function.
We can add text before and after the help section using the description and epilog argument inside the ArgumentParser()
function. The parse_args()
converts the arguments passed in the command prompt to objects and returns them, which can be used to perform operations later.
For example, let’s take a string argument from a user and display it. See the code below.
import argparse
import string
My_parser = argparse.ArgumentParser(
description="text before help.....", epilog="text after help....."
)
My_parser.add_argument("firstArg", help="You can use string here", type=str)
My_args = My_parser.parse_args()
print(My_args.firstArg)
Output:
PS C:\Users\ammar> python test.py
usage: test.py [-h] firstArg
test.py: error: the following arguments are required: firstArg
PS C:\Users\ammar> python test.py -h
usage: test.py [-h] firstArg
text before help.....
positional arguments:
firstArg You can use string here
optional arguments:
-h, --help show this help message and exit
text after help.....
PS C:\Users\ammar> python test.py hello
hello
We have run the above Python file three times and can see the result in the output. The first time, we ran the file without any argument, and an error message said that an argument was required, which was not passed.
The second time, we ran the script with the -h
argument, which is used for help, and the code displays the usage and help of the script. We ran the script a third time using a string displayed on the command prompt.
We can use the type
argument of the add_argument()
function to set the argument’s data type, like str for string and int for the integer data type. All the passed arguments are stored in the My_args
variable, and we can use this variable to check if a particular argument is passed or not.
Check if the argparse
Optional Argument Is Set
In the case of optional arguments, if no argument is passed, the parse_args()
method will return None
for that specific argument. We can use conditional statements to check if the argument is None
or not, and if the argument is None
, that means the argument is not passed.
For example, let’s add an optional argument and check if the argument is passed or not, and display a result accordingly. See the code below.
import argparse
import string
My_parser = argparse.ArgumentParser(
description="text before help.....", epilog="text after help....."
)
My_parser.add_argument("-firstArg", help="You can use string here", type=str)
My_args = My_parser.parse_args()
if My_args.firstArg is not None:
print("Argument passed")
else:
print("No Argument passed")
Output:
PS C:\Users\ammar> python test.py
No Argument passed
PS C:\Users\ammar> python test.py -firstArg hello
Argument passed
We ran the above script two times, with and without an argument, and displayed a text accordingly. We can use the is not None
and is None
statement with a conditional statement to determine if an argument is passed or not.
Note that in the case of optional arguments, we also have to use their name before passing an argument. Make sure the argument we want to check does not have a default value set because the argument will never be None
in the case of a default value.
For example, let’s add a default value in the above code using the default
keyword inside the add_argument()
function and repeat the above procedure. See the code below.
import argparse
import string
My_parser = argparse.ArgumentParser(
description="text before help.....", epilog="text after help....."
)
My_parser.add_argument(
"-firstArg", help="You can use string here", type=str, default="default_value"
)
My_args = My_parser.parse_args()
if My_args.firstArg is not None:
print("Argument passed")
else:
print("No Argument passed")
Output:
PS C:\Users\ammar> python test.py
Argument passed
PS C:\Users\ammar> python test.py -firstArg hello
Argument passed
We can observe in the above output that if we don’t pass an argument, the code will still display the argument passed because of the default value. We can use the add_argument()
function numerous times to add multiple arguments.
If we want to limit the user on the choice of inputs, we can use the choices
argument inside the add_argument()
and set its value to a list of values which will only be allowed to pass as the arguments to the script.