The any() Function in Python
-
Use the
any()
Function With Python List -
Use the
any()
Function With Python Tuple -
Use the
any()
Function With Python Set -
Use the
any()
Function With Python Dictionary
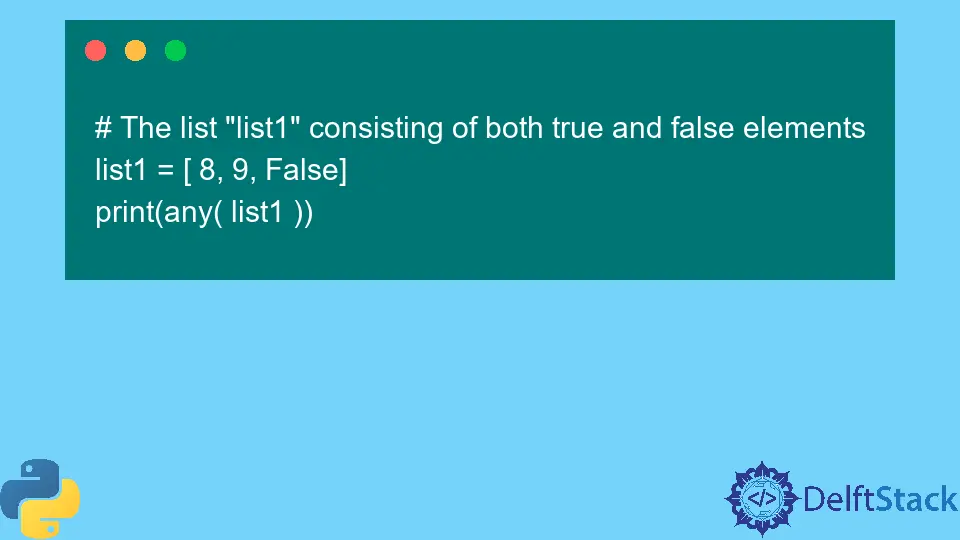
This tutorial demonstrates the use of the any()
function available in Python.
The any()
function is a simple Python built-in function that goes through the elements of a given iterable and provides either True
or False
value that indicates whether any one of the given pair of elements in the iterable is True
in a Boolean context.
In simple terms, when any of the items in a given iterable is True
, the any()
function returns True
. Technically, the working of the any()
function can be thought of as exactly the same as doing the OR
operation on the set of elements in the given iterable.
The syntax of the any()
function is:
any(iterable)
The any()
function takes only a single parameter, which could be any given iterable.
All the possible values that can be returned by the any()
function are,
- A
True
value is returned if any one of the elements of the given iterable is found to beTrue
. - A
False
value is returned if all of the elements of the given iterable are found to beFalse
.
The all()
function is similar to the any()
function in terms of syntax, with the only difference being that when all of the items in a given iterable is true
, then the all()
function returns True
, otherwise it returns False
in every other case. The all()
function technically behaves like the AND
operation on every iterable element.
The any()
function is versatile and can be used with various iterables.
Use the any()
Function With Python List
Lists are one of the four fundamental in-built data types that can be used in Python and are utilized to cluster several items in a single variable. Lists are changeable, ordered, and have a definite count.
The any()
function can be utilized on Lists
in the following way.
# The list "list1" consisting of both true and false elements
list1 = [8, 9, False]
print(any(list1))
The above code provides the following output:
True
Use the any()
Function With Python Tuple
Tuples are another of the four fundamental data types provided in Python and work similarly to lists. Tuples are ordered and unchangeable.
The any()
function can be utilized on tuples in the following way.
# The tuple "tuple1" consisting of both true and false elements
tuple1 = (8, 9, False)
print(any(tuple1))
The above code provides the following output:
True
Use the any()
Function With Python Set
In large, sets perform the same function of storing several items in a single variable like the other three built-in data types. The only difference is that sets are unordered and unindexed.
The any()
function can be utilized on sets in the following way.
# The set "set1" consisting of both true and false elements
set1 = {1, 2, 0, 8, False}
print(any(set1))
The above code provides the following output:
True
Use the any()
Function With Python Dictionary
The last of the four are Dictionaries. Dictionaries stock the data in key: value
pairs. The versatility of the any()
function makes it able to be used with Dictionaries as well.
The any()
function can be utilized on Dictionaries
in the following way.
# The dictionary "dict1" consisting of both true and false elements
dict1 = {1: "Hey", 2: "Great", 3: "Car"}
print(any(dict1))
The above code provides the following output:
True
The any()
function is a handy tool provided by Python, and it effectively comes into play when the programmers have to deal with complex conditional statements and booleans.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn